Browse Source
yes, sorry, was actually quite well formatted (clang-format default) ... but its probably just me working on the code ever. So, i will just use my preferences here. I find it important. visual guy and all other excuses...master
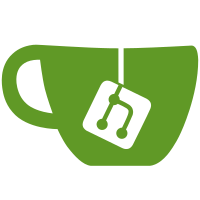
22 changed files with 7154 additions and 6336 deletions
@ -1,80 +1,98 @@ |
|||
#include "gbuffer.h" |
|||
|
|||
void gbuffer_copy_subrect(Glyph *src, Glyph *dest, Usz src_height, |
|||
Usz src_width, Usz dest_height, Usz dest_width, |
|||
Usz src_y, Usz src_x, Usz dest_y, Usz dest_x, |
|||
Usz height, Usz width) { |
|||
if (src_height <= src_y || src_width <= src_x || dest_height <= dest_y || |
|||
dest_width <= dest_x) |
|||
return; |
|||
Usz ny_0 = src_height - src_y; |
|||
Usz ny_1 = dest_height - dest_y; |
|||
Usz ny = height; |
|||
if (ny_0 < ny) |
|||
ny = ny_0; |
|||
if (ny_1 < ny) |
|||
ny = ny_1; |
|||
if (ny == 0) |
|||
return; |
|||
Usz row_copy_0 = src_width - src_x; |
|||
Usz row_copy_1 = dest_width - dest_x; |
|||
Usz row_copy = width; |
|||
if (row_copy_0 < row_copy) |
|||
row_copy = row_copy_0; |
|||
if (row_copy_1 < row_copy) |
|||
row_copy = row_copy_1; |
|||
Usz copy_bytes = row_copy * sizeof(Glyph); |
|||
Glyph *src_p = src + src_y * src_width + src_x; |
|||
Glyph *dest_p = dest + dest_y * dest_width + dest_x; |
|||
Isz src_stride; |
|||
Isz dest_stride; |
|||
if (src_y >= dest_y) { |
|||
src_stride = (Isz)src_width; |
|||
dest_stride = (Isz)dest_width; |
|||
} else { |
|||
src_p += (ny - 1) * src_width; |
|||
dest_p += (ny - 1) * dest_width; |
|||
src_stride = -(Isz)src_width; |
|||
dest_stride = -(Isz)dest_width; |
|||
} |
|||
Usz iy = 0; |
|||
for (;;) { |
|||
memmove(dest_p, src_p, copy_bytes); |
|||
++iy; |
|||
if (iy == ny) |
|||
break; |
|||
src_p += src_stride; |
|||
dest_p += dest_stride; |
|||
} |
|||
void gbuffer_copy_subrect( |
|||
Glyph *src, |
|||
Glyph *dest, |
|||
Usz src_height, |
|||
Usz src_width, |
|||
Usz dest_height, |
|||
Usz dest_width, |
|||
Usz src_y, |
|||
Usz src_x, |
|||
Usz dest_y, |
|||
Usz dest_x, |
|||
Usz height, |
|||
Usz width) |
|||
{ |
|||
if (src_height <= src_y || src_width <= src_x || dest_height <= dest_y || dest_width <= dest_x) |
|||
return; |
|||
Usz ny_0 = src_height - src_y; |
|||
Usz ny_1 = dest_height - dest_y; |
|||
Usz ny = height; |
|||
if (ny_0 < ny) |
|||
ny = ny_0; |
|||
if (ny_1 < ny) |
|||
ny = ny_1; |
|||
if (ny == 0) |
|||
return; |
|||
Usz row_copy_0 = src_width - src_x; |
|||
Usz row_copy_1 = dest_width - dest_x; |
|||
Usz row_copy = width; |
|||
if (row_copy_0 < row_copy) |
|||
row_copy = row_copy_0; |
|||
if (row_copy_1 < row_copy) |
|||
row_copy = row_copy_1; |
|||
Usz copy_bytes = row_copy * sizeof(Glyph); |
|||
Glyph *src_p = src + src_y * src_width + src_x; |
|||
Glyph *dest_p = dest + dest_y * dest_width + dest_x; |
|||
Isz src_stride; |
|||
Isz dest_stride; |
|||
if (src_y >= dest_y) { |
|||
src_stride = (Isz)src_width; |
|||
dest_stride = (Isz)dest_width; |
|||
} else { |
|||
src_p += (ny - 1) * src_width; |
|||
dest_p += (ny - 1) * dest_width; |
|||
src_stride = -(Isz)src_width; |
|||
dest_stride = -(Isz)dest_width; |
|||
} |
|||
Usz iy = 0; |
|||
for (;;) { |
|||
memmove(dest_p, src_p, copy_bytes); |
|||
++iy; |
|||
if (iy == ny) |
|||
break; |
|||
src_p += src_stride; |
|||
dest_p += dest_stride; |
|||
} |
|||
} |
|||
|
|||
void gbuffer_fill_subrect(Glyph *gbuffer, Usz f_height, Usz f_width, Usz y, |
|||
Usz x, Usz height, Usz width, Glyph fill_char) { |
|||
if (y >= f_height || x >= f_width) |
|||
return; |
|||
Usz rows_0 = f_height - y; |
|||
Usz rows = height; |
|||
if (rows_0 < rows) |
|||
rows = rows_0; |
|||
if (rows == 0) |
|||
return; |
|||
Usz columns_0 = f_width - x; |
|||
Usz columns = width; |
|||
if (columns_0 < columns) |
|||
columns = columns_0; |
|||
Usz fill_bytes = columns * sizeof(Glyph); |
|||
Glyph *p = gbuffer + y * f_width + x; |
|||
Usz iy = 0; |
|||
for (;;) { |
|||
memset(p, fill_char, fill_bytes); |
|||
++iy; |
|||
if (iy == rows) |
|||
break; |
|||
p += f_width; |
|||
} |
|||
void gbuffer_fill_subrect( |
|||
Glyph *gbuffer, |
|||
Usz f_height, |
|||
Usz f_width, |
|||
Usz y, |
|||
Usz x, |
|||
Usz height, |
|||
Usz width, |
|||
Glyph fill_char) |
|||
{ |
|||
if (y >= f_height || x >= f_width) |
|||
return; |
|||
Usz rows_0 = f_height - y; |
|||
Usz rows = height; |
|||
if (rows_0 < rows) |
|||
rows = rows_0; |
|||
if (rows == 0) |
|||
return; |
|||
Usz columns_0 = f_width - x; |
|||
Usz columns = width; |
|||
if (columns_0 < columns) |
|||
columns = columns_0; |
|||
Usz fill_bytes = columns * sizeof(Glyph); |
|||
Glyph *p = gbuffer + y * f_width + x; |
|||
Usz iy = 0; |
|||
for (;;) { |
|||
memset(p, fill_char, fill_bytes); |
|||
++iy; |
|||
if (iy == rows) |
|||
break; |
|||
p += f_width; |
|||
} |
|||
} |
|||
|
|||
void mbuffer_clear(Mark *mbuf, Usz height, Usz width) { |
|||
Usz cleared_size = height * width; |
|||
memset(mbuf, 0, cleared_size); |
|||
void mbuffer_clear(Mark *mbuf, Usz height, Usz width) |
|||
{ |
|||
Usz cleared_size = height * width; |
|||
memset(mbuf, 0, cleared_size); |
|||
} |
|||
|
@ -1,85 +1,122 @@ |
|||
#pragma once |
|||
#include "base.h" |
|||
|
|||
ORCA_PURE static inline Glyph gbuffer_peek_relative(Glyph *gbuf, Usz height, |
|||
Usz width, Usz y, Usz x, |
|||
Isz delta_y, Isz delta_x) { |
|||
Isz y0 = (Isz)y + delta_y; |
|||
Isz x0 = (Isz)x + delta_x; |
|||
if (y0 < 0 || x0 < 0 || (Usz)y0 >= height || (Usz)x0 >= width) |
|||
return '.'; |
|||
return gbuf[(Usz)y0 * width + (Usz)x0]; |
|||
ORCA_PURE static inline Glyph gbuffer_peek_relative( |
|||
Glyph *gbuf, |
|||
Usz height, |
|||
Usz width, |
|||
Usz y, |
|||
Usz x, |
|||
Isz delta_y, |
|||
Isz delta_x) |
|||
{ |
|||
Isz y0 = (Isz)y + delta_y; |
|||
Isz x0 = (Isz)x + delta_x; |
|||
if (y0 < 0 || x0 < 0 || (Usz)y0 >= height || (Usz)x0 >= width) |
|||
return '.'; |
|||
return gbuf[(Usz)y0 * width + (Usz)x0]; |
|||
} |
|||
|
|||
static inline void gbuffer_poke(Glyph *gbuf, Usz height, Usz width, Usz y, |
|||
Usz x, Glyph g) { |
|||
assert(y < height && x < width); |
|||
(void)height; |
|||
gbuf[y * width + x] = g; |
|||
static inline void gbuffer_poke(Glyph *gbuf, Usz height, Usz width, Usz y, Usz x, Glyph g) |
|||
{ |
|||
assert(y < height && x < width); |
|||
(void)height; |
|||
gbuf[y * width + x] = g; |
|||
} |
|||
|
|||
static inline void gbuffer_poke_relative(Glyph *gbuf, Usz height, Usz width, |
|||
Usz y, Usz x, Isz delta_y, Isz delta_x, |
|||
Glyph g) { |
|||
Isz y0 = (Isz)y + delta_y; |
|||
Isz x0 = (Isz)x + delta_x; |
|||
if (y0 < 0 || x0 < 0 || (Usz)y0 >= height || (Usz)x0 >= width) |
|||
return; |
|||
gbuf[(Usz)y0 * width + (Usz)x0] = g; |
|||
static inline void gbuffer_poke_relative( |
|||
Glyph *gbuf, |
|||
Usz height, |
|||
Usz width, |
|||
Usz y, |
|||
Usz x, |
|||
Isz delta_y, |
|||
Isz delta_x, |
|||
Glyph g) |
|||
{ |
|||
Isz y0 = (Isz)y + delta_y; |
|||
Isz x0 = (Isz)x + delta_x; |
|||
if (y0 < 0 || x0 < 0 || (Usz)y0 >= height || (Usz)x0 >= width) |
|||
return; |
|||
gbuf[(Usz)y0 * width + (Usz)x0] = g; |
|||
} |
|||
|
|||
ORCA_NOINLINE |
|||
void gbuffer_copy_subrect(Glyph *src, Glyph *dest, Usz src_grid_h, |
|||
Usz src_grid_w, Usz dest_grid_h, Usz dest_grid_w, |
|||
Usz src_y, Usz src_x, Usz dest_y, Usz dest_x, |
|||
Usz height, Usz width); |
|||
void gbuffer_copy_subrect( |
|||
Glyph *src, |
|||
Glyph *dest, |
|||
Usz src_grid_h, |
|||
Usz src_grid_w, |
|||
Usz dest_grid_h, |
|||
Usz dest_grid_w, |
|||
Usz src_y, |
|||
Usz src_x, |
|||
Usz dest_y, |
|||
Usz dest_x, |
|||
Usz height, |
|||
Usz width); |
|||
|
|||
ORCA_NOINLINE |
|||
void gbuffer_fill_subrect(Glyph *gbuf, Usz grid_h, Usz grid_w, Usz y, Usz x, |
|||
Usz height, Usz width, Glyph fill_char); |
|||
void gbuffer_fill_subrect( |
|||
Glyph *gbuf, |
|||
Usz grid_h, |
|||
Usz grid_w, |
|||
Usz y, |
|||
Usz x, |
|||
Usz height, |
|||
Usz width, |
|||
Glyph fill_char); |
|||
|
|||
typedef enum { |
|||
Mark_flag_none = 0, |
|||
Mark_flag_input = 1 << 0, |
|||
Mark_flag_output = 1 << 1, |
|||
Mark_flag_haste_input = 1 << 2, |
|||
Mark_flag_lock = 1 << 3, |
|||
Mark_flag_sleep = 1 << 4, |
|||
typedef enum |
|||
{ |
|||
Mark_flag_none = 0, |
|||
Mark_flag_input = 1 << 0, |
|||
Mark_flag_output = 1 << 1, |
|||
Mark_flag_haste_input = 1 << 2, |
|||
Mark_flag_lock = 1 << 3, |
|||
Mark_flag_sleep = 1 << 4, |
|||
} Mark_flags; |
|||
|
|||
ORCA_OK_IF_UNUSED |
|||
static Mark_flags mbuffer_peek(Mark *mbuf, Usz height, Usz width, Usz y, |
|||
Usz x) { |
|||
(void)height; |
|||
return mbuf[y * width + x]; |
|||
static Mark_flags mbuffer_peek(Mark *mbuf, Usz height, Usz width, Usz y, Usz x) |
|||
{ |
|||
(void)height; |
|||
return mbuf[y * width + x]; |
|||
} |
|||
|
|||
ORCA_OK_IF_UNUSED |
|||
static Mark_flags mbuffer_peek_relative(Mark *mbuf, Usz height, Usz width, |
|||
Usz y, Usz x, Isz offs_y, Isz offs_x) { |
|||
Isz y0 = (Isz)y + offs_y; |
|||
Isz x0 = (Isz)x + offs_x; |
|||
if (y0 >= (Isz)height || x0 >= (Isz)width || y0 < 0 || x0 < 0) |
|||
return Mark_flag_none; |
|||
return mbuf[(Usz)y0 * width + (Usz)x0]; |
|||
static Mark_flags mbuffer_peek_relative(Mark *mbuf, Usz height, Usz width, Usz y, Usz x, Isz offs_y, Isz offs_x) |
|||
{ |
|||
Isz y0 = (Isz)y + offs_y; |
|||
Isz x0 = (Isz)x + offs_x; |
|||
if (y0 >= (Isz)height || x0 >= (Isz)width || y0 < 0 || x0 < 0) |
|||
return Mark_flag_none; |
|||
return mbuf[(Usz)y0 * width + (Usz)x0]; |
|||
} |
|||
|
|||
ORCA_OK_IF_UNUSED |
|||
static void mbuffer_poke_flags_or(Mark *mbuf, Usz height, Usz width, Usz y, |
|||
Usz x, Mark_flags flags) { |
|||
(void)height; |
|||
mbuf[y * width + x] |= (Mark)flags; |
|||
static void mbuffer_poke_flags_or(Mark *mbuf, Usz height, Usz width, Usz y, Usz x, Mark_flags flags) |
|||
{ |
|||
(void)height; |
|||
mbuf[y * width + x] |= (Mark)flags; |
|||
} |
|||
|
|||
ORCA_OK_IF_UNUSED |
|||
static void mbuffer_poke_relative_flags_or(Mark *mbuf, Usz height, Usz width, |
|||
Usz y, Usz x, Isz offs_y, Isz offs_x, |
|||
Mark_flags flags) { |
|||
Isz y0 = (Isz)y + offs_y; |
|||
Isz x0 = (Isz)x + offs_x; |
|||
if (y0 >= (Isz)height || x0 >= (Isz)width || y0 < 0 || x0 < 0) |
|||
return; |
|||
mbuf[(Usz)y0 * width + (Usz)x0] |= (Mark)flags; |
|||
static void mbuffer_poke_relative_flags_or( |
|||
Mark *mbuf, |
|||
Usz height, |
|||
Usz width, |
|||
Usz y, |
|||
Usz x, |
|||
Isz offs_y, |
|||
Isz offs_x, |
|||
Mark_flags flags) |
|||
{ |
|||
Isz y0 = (Isz)y + offs_y; |
|||
Isz x0 = (Isz)x + offs_x; |
|||
if (y0 >= (Isz)height || x0 >= (Isz)width || y0 < 0 || x0 < 0) |
|||
return; |
|||
mbuf[(Usz)y0 * width + (Usz)x0] |= (Mark)flags; |
|||
} |
|||
|
|||
void mbuffer_clear(Mark *mbuf, Usz height, Usz width); |
|||
|
File diff suppressed because it is too large
File diff suppressed because it is too large
File diff suppressed because it is too large
File diff suppressed because it is too large
@ -1,33 +1,42 @@ |
|||
#include "vmio.h" |
|||
|
|||
void oevent_list_init(Oevent_list *olist) { |
|||
olist->buffer = NULL; |
|||
olist->count = 0; |
|||
olist->capacity = 0; |
|||
void oevent_list_init(Oevent_list *olist) |
|||
{ |
|||
olist->buffer = NULL; |
|||
olist->count = 0; |
|||
olist->capacity = 0; |
|||
} |
|||
void oevent_list_deinit(Oevent_list *olist) { free(olist->buffer); } |
|||
void oevent_list_clear(Oevent_list *olist) { olist->count = 0; } |
|||
void oevent_list_copy(Oevent_list const *src, Oevent_list *dest) { |
|||
Usz src_count = src->count; |
|||
if (dest->capacity < src_count) { |
|||
Usz new_cap = orca_round_up_power2(src_count); |
|||
dest->buffer = realloc(dest->buffer, new_cap * sizeof(Oevent)); |
|||
dest->capacity = new_cap; |
|||
} |
|||
memcpy(dest->buffer, src->buffer, src_count * sizeof(Oevent)); |
|||
dest->count = src_count; |
|||
void oevent_list_deinit(Oevent_list *olist) |
|||
{ |
|||
free(olist->buffer); |
|||
} |
|||
Oevent *oevent_list_alloc_item(Oevent_list *olist) { |
|||
Usz count = olist->count; |
|||
if (olist->capacity == count) { |
|||
// Note: no overflow check, but you're probably out of memory if this
|
|||
// happens anyway. Like other uses of realloc in orca, we also don't check
|
|||
// for a failed allocation.
|
|||
Usz capacity = count < 16 ? 16 : orca_round_up_power2(count + 1); |
|||
olist->buffer = realloc(olist->buffer, capacity * sizeof(Oevent)); |
|||
olist->capacity = capacity; |
|||
} |
|||
Oevent *result = olist->buffer + count; |
|||
olist->count = count + 1; |
|||
return result; |
|||
void oevent_list_clear(Oevent_list *olist) |
|||
{ |
|||
olist->count = 0; |
|||
} |
|||
void oevent_list_copy(Oevent_list const *src, Oevent_list *dest) |
|||
{ |
|||
Usz src_count = src->count; |
|||
if (dest->capacity < src_count) { |
|||
Usz new_cap = orca_round_up_power2(src_count); |
|||
dest->buffer = realloc(dest->buffer, new_cap * sizeof(Oevent)); |
|||
dest->capacity = new_cap; |
|||
} |
|||
memcpy(dest->buffer, src->buffer, src_count * sizeof(Oevent)); |
|||
dest->count = src_count; |
|||
} |
|||
Oevent *oevent_list_alloc_item(Oevent_list *olist) |
|||
{ |
|||
Usz count = olist->count; |
|||
if (olist->capacity == count) { |
|||
// Note: no overflow check, but you're probably out of memory if this
|
|||
// happens anyway. Like other uses of realloc in orca, we also don't check
|
|||
// for a failed allocation.
|
|||
Usz capacity = count < 16 ? 16 : orca_round_up_power2(count + 1); |
|||
olist->buffer = realloc(olist->buffer, capacity * sizeof(Oevent)); |
|||
olist->capacity = capacity; |
|||
} |
|||
Oevent *result = olist->buffer + count; |
|||
olist->count = count + 1; |
|||
return result; |
|||
} |
|||
|
Loading…
Reference in new issue