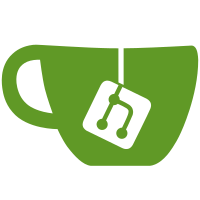
1 changed files with 110 additions and 1 deletions
@ -1 +1,110 @@ |
|||
HULU |
|||
<!DOCTYPE html> |
|||
<html lang="en"> |
|||
<head> |
|||
<title>three.js webgl - shader [Monjori]</title> |
|||
<meta charset="utf-8"> |
|||
<script id="vertexShader" type="x-shader/x-vertex"> |
|||
varying vec2 vUv; |
|||
|
|||
void main() { |
|||
vUv = uv; |
|||
gl_Position = vec4(position, 1.0); |
|||
} |
|||
</script> |
|||
<script id="fragmentShader" type="x-shader/x-fragment"> |
|||
#ifdef GL_ES |
|||
precision highp float; |
|||
#endif |
|||
|
|||
// #extension GL_OES_standard_derivatives : enable |
|||
|
|||
uniform float time; |
|||
uniform vec2 mouse; |
|||
uniform vec2 resolution; |
|||
|
|||
void main(void) { |
|||
vec2 uv = (gl_FragCoord.xy - resolution * 0.7) / max(resolution.x, resolution.y) * 2.998; |
|||
vec2 position = gl_FragCoord.xy / resolution.xy; |
|||
float t = 0.255 / abs(abs(sin(1.)) - length(uv)); |
|||
uv *= 1.0; |
|||
|
|||
float e = 0.25; |
|||
for (float i=3.0;i<=15.0;i+=1.0) { |
|||
e += 0.01/abs((i/15.) +sin((time/2.0) + 0.15*i*(uv.x) *(cos(i/4.0666 + (time / 2.0) + uv.x*2.2))) + 2.5*uv.y); |
|||
|
|||
gl_FragColor = vec4(vec3(e/1.5, e/1.5, e/1.5), 1.6); |
|||
//gl_FragColor.r = 1.0; |
|||
gl_FragColor.g = mouse.x / 15.0 + 0.128; |
|||
gl_FragColor.b = uv.y / 10.0 + 0.25; |
|||
gl_FragColor.a = uv.y + 1.4; |
|||
} |
|||
|
|||
} |
|||
</script> |
|||
</head> |
|||
|
|||
<body> |
|||
<div id="container"></div> |
|||
|
|||
<script type="module"> |
|||
import * as THREE from 'https://unpkg.com/three/build/three.module.js'; |
|||
|
|||
let camera, scene, renderer; |
|||
let uniforms; |
|||
init(); |
|||
animate(); |
|||
|
|||
function init() { |
|||
const container = document.getElementById( 'container' ); |
|||
camera = new THREE.OrthographicCamera( - 1, 1, 1, - 1, 0, 1 ); |
|||
scene = new THREE.Scene(); |
|||
const geometry = new THREE.PlaneGeometry( 2, 2 ); |
|||
|
|||
let quality = 1 |
|||
uniforms = { |
|||
time: { value: 1.0 }, |
|||
resolution: { value: new THREE.Vector2( window.innerWidth / quality, window.innerHeight / quality ) }, |
|||
mouse: { value: new THREE.Vector2(0.0,0.0) } |
|||
}; |
|||
|
|||
const material = new THREE.ShaderMaterial( { |
|||
uniforms: uniforms, |
|||
vertexShader: document.getElementById( 'vertexShader' ).textContent, |
|||
fragmentShader: document.getElementById( 'fragmentShader' ).textContent |
|||
|
|||
} ); |
|||
|
|||
const mesh = new THREE.Mesh( geometry, material ); |
|||
scene.add( mesh ); |
|||
renderer = new THREE.WebGLRenderer(); |
|||
renderer.setPixelRatio( window.devicePixelRatio ); |
|||
container.appendChild( renderer.domElement ); |
|||
onWindowResize(); |
|||
window.addEventListener( 'resize', onWindowResize ); |
|||
document.addEventListener( 'pointermove', onPointerMove); |
|||
} |
|||
|
|||
function onPointerMove(event) { |
|||
var clientX = event.clientX; |
|||
var clientY = event.clientY; |
|||
|
|||
var mouseX = clientX / window.innerWidth; |
|||
var mouseY = 1 - clientY / window.innerHeight; |
|||
|
|||
uniforms[ 'mouse' ].value = new THREE.Vector2(mouseX, mouseY); |
|||
} |
|||
|
|||
function onWindowResize() { |
|||
renderer.setSize( window.innerWidth, window.innerHeight ); |
|||
} |
|||
|
|||
|
|||
function animate() { |
|||
requestAnimationFrame( animate ); |
|||
uniforms[ 'time' ].value = performance.now() / 1000; |
|||
renderer.render( scene, camera ); |
|||
} |
|||
|
|||
</script> |
|||
</body> |
|||
</html> |
|||
|
Loading…
Reference in new issue