Browse Source
Previously, the Qnav blocks (the menu panels) would have their position determined once when they're first created, and then never updated. The positioning of Qnav blocks is determined by some code that may cause the blocks to wrap or be positioned differently depending on how much screen space is available. For example, if you open a nested menu that's three blocks/panels deep, and the third panel doesn't have enough room on the right in the terminal, it may be shown below the second panel instead of to the right. This had a flaw. If the user resized their terminal after the menus were already open, the positions of the menu panels wouldn't be adjusted to accommodate the new screen size. There wasn't a way to trigger a full re-layout of all the menu items when the terminal was resized. This commit adds qnav_adjust_term_size(), which is called from tui_main.c when the terminal is resized. This function will re-layout the menu panels.master
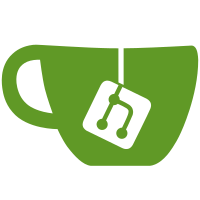
3 changed files with 57 additions and 33 deletions
Loading…
Reference in new issue