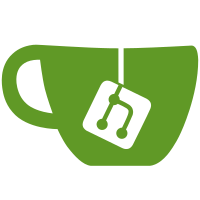
commit
987174db31
3 changed files with 192 additions and 0 deletions
@ -0,0 +1,67 @@ |
|||
4 => int device; |
|||
13 => int ctlNrFreq; |
|||
16 => int ctlNrVol; |
|||
9 => int ctlNrAux1; |
|||
10 => int ctlNrAux2; |
|||
|
|||
64 => int voicesNr; |
|||
|
|||
// Midi |
|||
MidiIn min; |
|||
MidiMsg msg; |
|||
if( !min.open( device ) ) { |
|||
me.exit(); |
|||
} |
|||
<<< "MIDI device:", min.num(), " -> ", min.name() >>>; |
|||
|
|||
//UGENs |
|||
SinOsc s[voicesNr]; |
|||
for(0 => int i; i < voicesNr-2; i++) { |
|||
s[i] => s[i+1]; |
|||
s[i].sync(2); |
|||
} |
|||
s[voicesNr-3] => dac; |
|||
|
|||
|
|||
//params |
|||
0. => float aux1; |
|||
0. => float aux2; |
|||
0. => float freq; |
|||
0.1 => float gain; |
|||
|
|||
while( true ) |
|||
{ |
|||
min => now; |
|||
|
|||
while( min.recv(msg) ) { |
|||
<<< msg.data1, msg.data2, msg.data3 >>>; |
|||
} |
|||
|
|||
|
|||
if(msg.data2 == ctlNrFreq) { |
|||
msg.data3 => freq; |
|||
} |
|||
if(msg.data2 == ctlNrVol) { |
|||
msg.data3/127. => gain; |
|||
} |
|||
if(msg.data2 == ctlNrAux1) { |
|||
msg.data3/127. => aux1; |
|||
} |
|||
if(msg.data2 == ctlNrAux2) { |
|||
msg.data3/127. => aux2; |
|||
} |
|||
|
|||
for(0 => int i; i < voicesNr; i++) { |
|||
voice(i); |
|||
} |
|||
|
|||
} |
|||
|
|||
fun void voice(int i) { |
|||
1. / voicesNr => s[i].gain; |
|||
50 + i => s[i].freq; |
|||
freq * (i+aux1) => s[i].sfreq; |
|||
gain => s[i].gain; |
|||
|
|||
} |
|||
|
@ -0,0 +1,97 @@ |
|||
4 => int device; |
|||
13 => int ctlNrFreq; |
|||
16 => int ctlNrVol; |
|||
12 => int ctlNrVoiceNr; |
|||
9 => int ctlNrAux1; |
|||
10 => int ctlNrAux2; |
|||
|
|||
64 => int voicesMax; |
|||
1 => int voicesNr; |
|||
|
|||
// Midi |
|||
MidiIn min; |
|||
MidiMsg msg; |
|||
if( !min.open( device ) ) { |
|||
me.exit(); |
|||
} |
|||
<<< "MIDI device:", min.num(), " -> ", min.name() >>>; |
|||
|
|||
//UGENs |
|||
SinOsc s[voicesMax]; |
|||
UGen sum; |
|||
sum => dac; |
|||
|
|||
//params |
|||
0. => float freq; |
|||
0.7 => float gain; |
|||
0.2 => float voicesRaw; |
|||
0. => float aux1; |
|||
0. => float aux2; |
|||
|
|||
|
|||
1 => int synthInvalidated; |
|||
1 => int patchInvalidated; |
|||
while( true ) { |
|||
//Patch |
|||
if(patchInvalidated) { |
|||
<<< "voices: " + voicesNr >>>; |
|||
setupOscPatch(voicesRaw); |
|||
0 => patchInvalidated; |
|||
} |
|||
//Sythesis |
|||
if(synthInvalidated == 1) { |
|||
<<< freq,aux1,aux2,gain >>>; |
|||
|
|||
for(0 => int i; i < voicesNr; i++) { |
|||
voice(i); |
|||
} |
|||
0 => synthInvalidated; |
|||
} |
|||
min => now; |
|||
//Parsing |
|||
min.recv(msg); |
|||
// <<< msg.data1, msg.data2, msg.data3 >>>; |
|||
|
|||
if(msg.data2 == ctlNrVoiceNr) { |
|||
1 => patchInvalidated; |
|||
1 => synthInvalidated; |
|||
msg.data3/127. => voicesRaw; |
|||
} |
|||
if(msg.data2 == ctlNrFreq) { |
|||
1 => synthInvalidated; |
|||
msg.data3 => freq; |
|||
} |
|||
if(msg.data2 == ctlNrVol) { |
|||
1 => synthInvalidated; |
|||
msg.data3/127. => gain; |
|||
} |
|||
if(msg.data2 == ctlNrAux1) { |
|||
1 => synthInvalidated; |
|||
msg.data3/127. => aux1; |
|||
} |
|||
if(msg.data2 == ctlNrAux2) { |
|||
1 => synthInvalidated; |
|||
msg.data3/127. => aux2; |
|||
} |
|||
|
|||
} |
|||
|
|||
fun void voice(int i) { |
|||
50 + freq +i => s[i].sfreq; |
|||
i * (aux1/voicesNr+1) => float phase; |
|||
phase => s[i].phase; |
|||
gain => s[i].gain; |
|||
} |
|||
|
|||
fun void setupOscPatch(float pVoicesRaw) { |
|||
|
|||
Math.max(1.,Math.floor(voicesMax * pVoicesRaw)) $ int => voicesNr; |
|||
for(0 => int i; i < voicesMax; i++) { |
|||
s[i] =< sum; |
|||
} |
|||
for(0 => int i; i < voicesNr; i++) { |
|||
s[i] => sum; |
|||
} |
|||
1./voicesNr => sum.gain; |
|||
} |
|||
|
@ -0,0 +1,28 @@ |
|||
4 => int device; |
|||
|
|||
MidiIn min; |
|||
MidiMsg msg; |
|||
|
|||
SinOsc s => dac; |
|||
|
|||
|
|||
if( !min.open( device ) ) { |
|||
me.exit(); |
|||
} |
|||
<<< "MIDI device:", min.num(), " -> ", min.name() >>>; |
|||
|
|||
// infinite time-loop |
|||
while( true ) |
|||
{ |
|||
min => now; |
|||
|
|||
while( min.recv(msg) ) |
|||
{ |
|||
<<< msg.data1, msg.data2, msg.data3 >>>; |
|||
} |
|||
13 => int ctlNrFreq; |
|||
|
|||
if(msg.data2 == 13) { |
|||
msg.data3 => s.freq; |
|||
} |
|||
} |
Loading…
Reference in new issue