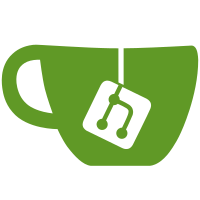
3 changed files with 134 additions and 0 deletions
@ -0,0 +1,11 @@ |
|||
#ifndef INC_TYPES_H |
|||
#define INC_TYPES_H |
|||
|
|||
#include <cstdint> |
|||
|
|||
using u8 = uint8_t; |
|||
using u16 = uint16_t; |
|||
using u32 = uint32_t; |
|||
using u64 = uint64_t; |
|||
|
|||
#endif |
@ -0,0 +1,82 @@ |
|||
#include "utils.h" |
|||
#include "init.h" |
|||
#include "usbd_cdc_if.h" |
|||
#include <sstream> |
|||
#include <bitset> |
|||
|
|||
namespace Heck::Utils { |
|||
bool debug_suspend_active = false; |
|||
|
|||
void debug_suspend_continue() |
|||
{ |
|||
debug_suspend_active = false; |
|||
} |
|||
|
|||
void log(std::string msg) |
|||
{ |
|||
std::string out{ msg }; |
|||
out.append("\r\n"); |
|||
u8 status = CDC_Transmit_FS((uint8_t *)out.data(), out.size()); |
|||
if (status == USBD_BUSY) { |
|||
// usb buffer overflow
|
|||
} |
|||
} |
|||
|
|||
std::string reg_to_string(uint32_t val) |
|||
{ |
|||
std::stringstream ss{}; |
|||
const std::bitset<32> x{ val }; |
|||
ss << "0b" << x.to_string(); |
|||
ss << " - "; |
|||
ss << std::to_string(val); |
|||
return ss.str(); |
|||
} |
|||
|
|||
u32 random(u32 max) |
|||
{ |
|||
u32 ret{ 0 }; |
|||
HAL_RNG_GenerateRandomNumber(&hrng, &ret); |
|||
ret %= max; |
|||
return ret; |
|||
} |
|||
|
|||
|
|||
} // namespace Heck::Utils
|
|||
|
|||
extern "C" void heck_debug_suspend(void) |
|||
{ |
|||
Heck::Utils::debug_suspend_active = true; |
|||
while (Heck::Utils::debug_suspend_active == true) { |
|||
Heck::Utils::log("debug_suspend..."); |
|||
HAL_Delay(10); |
|||
} |
|||
} |
|||
|
|||
extern "C" void heck_log(char *msg) |
|||
{ |
|||
Heck::Utils::log(std::string(msg)); |
|||
} |
|||
|
|||
/**
|
|||
* @brief Reports the name of the source file and the source line number |
|||
* where the assert_param error has occurred. |
|||
* @param file: pointer to the source file name |
|||
* @param line: assert_param error line source number |
|||
* @retval None |
|||
*/ |
|||
extern "C" void assert_failed(uint8_t *file, uint32_t line) |
|||
{ |
|||
/* User can add his own implementation to report the file name and line number,
|
|||
ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */ |
|||
int str_size = 1024; |
|||
char str[str_size]; |
|||
snprintf(str, str_size, "assert failed: %s:%d", file, line); |
|||
heck_log(str); |
|||
} |
|||
|
|||
extern "C" void Error_Handler(void) |
|||
{ |
|||
heck_log("Error_Handler StR1keZ!"); |
|||
__disable_irq(); |
|||
while (1) {} |
|||
} |
@ -0,0 +1,41 @@ |
|||
#ifndef INC_UTILS_H |
|||
#define INC_UTILS_H |
|||
|
|||
|
|||
// ----------------------------------------------------------------------------------------------
|
|||
// C Interface
|
|||
// ----------------------------------------------------------------------------------------------
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
#include "stdint.h" |
|||
void heck_debug_suspend(void); |
|||
void heck_log(char* msg); |
|||
|
|||
void assert_failed(uint8_t* file, uint32_t line); |
|||
void Error_Handler(void); |
|||
#ifdef __cplusplus |
|||
}; |
|||
#endif |
|||
|
|||
// ----------------------------------------------------------------------------------------------
|
|||
// C++ Interface
|
|||
// ----------------------------------------------------------------------------------------------
|
|||
|
|||
|
|||
#ifdef __cplusplus |
|||
#include "types.hh" |
|||
#include <iostream> |
|||
#include <cstdint> |
|||
#include <string> |
|||
|
|||
namespace Heck::Utils { |
|||
void debug_suspend_continue(); |
|||
void log(std::string msg); |
|||
std::string reg_to_string(uint32_t val); |
|||
u32 random(u32 max); |
|||
|
|||
} // namespace Heck::Utils
|
|||
|
|||
#endif |
|||
#endif /* INC_CPPMAIN_H */ |
Loading…
Reference in new issue