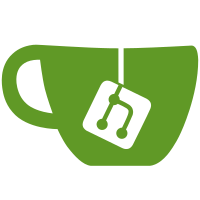
35 changed files with 13018 additions and 90 deletions
File diff suppressed because one or more lines are too long
@ -0,0 +1,49 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file gpio.h |
|||
* @brief This file contains all the function prototypes for |
|||
* the gpio.c file |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __GPIO_H__ |
|||
#define __GPIO_H__ |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "main.h" |
|||
|
|||
/* USER CODE BEGIN Includes */ |
|||
|
|||
/* USER CODE END Includes */ |
|||
|
|||
/* USER CODE BEGIN Private defines */ |
|||
|
|||
/* USER CODE END Private defines */ |
|||
|
|||
void MX_GPIO_Init(void); |
|||
|
|||
/* USER CODE BEGIN Prototypes */ |
|||
|
|||
/* USER CODE END Prototypes */ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
#endif /*__ GPIO_H__ */ |
|||
|
@ -0,0 +1,67 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file gpio.c |
|||
* @brief This file provides code for the configuration |
|||
* of all used GPIO pins. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "gpio.h" |
|||
|
|||
/* USER CODE BEGIN 0 */ |
|||
|
|||
/* USER CODE END 0 */ |
|||
|
|||
/*----------------------------------------------------------------------------*/ |
|||
/* Configure GPIO */ |
|||
/*----------------------------------------------------------------------------*/ |
|||
/* USER CODE BEGIN 1 */ |
|||
|
|||
/* USER CODE END 1 */ |
|||
|
|||
/** Configure pins as
|
|||
* Analog |
|||
* Input |
|||
* Output |
|||
* EVENT_OUT |
|||
* EXTI |
|||
*/ |
|||
void MX_GPIO_Init(void) |
|||
{ |
|||
|
|||
GPIO_InitTypeDef GPIO_InitStruct = {0}; |
|||
|
|||
/* GPIO Ports Clock Enable */ |
|||
__HAL_RCC_GPIOC_CLK_ENABLE(); |
|||
__HAL_RCC_GPIOH_CLK_ENABLE(); |
|||
__HAL_RCC_GPIOD_CLK_ENABLE(); |
|||
__HAL_RCC_GPIOA_CLK_ENABLE(); |
|||
|
|||
/*Configure GPIO pin Output Level */ |
|||
HAL_GPIO_WritePin(LED_GREEN_GPIO_Port, LED_GREEN_Pin, GPIO_PIN_RESET); |
|||
|
|||
/*Configure GPIO pin : PtPin */ |
|||
GPIO_InitStruct.Pin = LED_GREEN_Pin; |
|||
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; |
|||
GPIO_InitStruct.Pull = GPIO_NOPULL; |
|||
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; |
|||
HAL_GPIO_Init(LED_GREEN_GPIO_Port, &GPIO_InitStruct); |
|||
|
|||
} |
|||
|
|||
/* USER CODE BEGIN 2 */ |
|||
|
|||
/* USER CODE END 2 */ |
@ -0,0 +1,459 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file stm32f4xx_hal_pcd.h |
|||
* @author MCD Application Team |
|||
* @brief Header file of PCD HAL module. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2016 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef STM32F4xx_HAL_PCD_H |
|||
#define STM32F4xx_HAL_PCD_H |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "stm32f4xx_ll_usb.h" |
|||
|
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
|
|||
/** @addtogroup STM32F4xx_HAL_Driver
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @addtogroup PCD
|
|||
* @{ |
|||
*/ |
|||
|
|||
/* Exported types ------------------------------------------------------------*/ |
|||
/** @defgroup PCD_Exported_Types PCD Exported Types
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @brief PCD State structure definition |
|||
*/ |
|||
typedef enum |
|||
{ |
|||
HAL_PCD_STATE_RESET = 0x00, |
|||
HAL_PCD_STATE_READY = 0x01, |
|||
HAL_PCD_STATE_ERROR = 0x02, |
|||
HAL_PCD_STATE_BUSY = 0x03, |
|||
HAL_PCD_STATE_TIMEOUT = 0x04 |
|||
} PCD_StateTypeDef; |
|||
|
|||
/* Device LPM suspend state */ |
|||
typedef enum |
|||
{ |
|||
LPM_L0 = 0x00, /* on */ |
|||
LPM_L1 = 0x01, /* LPM L1 sleep */ |
|||
LPM_L2 = 0x02, /* suspend */ |
|||
LPM_L3 = 0x03, /* off */ |
|||
} PCD_LPM_StateTypeDef; |
|||
|
|||
typedef enum |
|||
{ |
|||
PCD_LPM_L0_ACTIVE = 0x00, /* on */ |
|||
PCD_LPM_L1_ACTIVE = 0x01, /* LPM L1 sleep */ |
|||
} PCD_LPM_MsgTypeDef; |
|||
|
|||
typedef enum |
|||
{ |
|||
PCD_BCD_ERROR = 0xFF, |
|||
PCD_BCD_CONTACT_DETECTION = 0xFE, |
|||
PCD_BCD_STD_DOWNSTREAM_PORT = 0xFD, |
|||
PCD_BCD_CHARGING_DOWNSTREAM_PORT = 0xFC, |
|||
PCD_BCD_DEDICATED_CHARGING_PORT = 0xFB, |
|||
PCD_BCD_DISCOVERY_COMPLETED = 0x00, |
|||
|
|||
} PCD_BCD_MsgTypeDef; |
|||
|
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
typedef USB_OTG_GlobalTypeDef PCD_TypeDef; |
|||
typedef USB_OTG_CfgTypeDef PCD_InitTypeDef; |
|||
typedef USB_OTG_EPTypeDef PCD_EPTypeDef; |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
/**
|
|||
* @brief PCD Handle Structure definition |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
typedef struct __PCD_HandleTypeDef |
|||
#else |
|||
typedef struct |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
PCD_TypeDef *Instance; /*!< Register base address */ |
|||
PCD_InitTypeDef Init; /*!< PCD required parameters */ |
|||
__IO uint8_t USB_Address; /*!< USB Address */ |
|||
PCD_EPTypeDef IN_ep[16]; /*!< IN endpoint parameters */ |
|||
PCD_EPTypeDef OUT_ep[16]; /*!< OUT endpoint parameters */ |
|||
HAL_LockTypeDef Lock; /*!< PCD peripheral status */ |
|||
__IO PCD_StateTypeDef State; /*!< PCD communication state */ |
|||
__IO uint32_t ErrorCode; /*!< PCD Error code */ |
|||
uint32_t Setup[12]; /*!< Setup packet buffer */ |
|||
PCD_LPM_StateTypeDef LPM_State; /*!< LPM State */ |
|||
uint32_t BESL; |
|||
uint32_t FrameNumber; /*!< Store Current Frame number */ |
|||
|
|||
|
|||
uint32_t lpm_active; /*!< Enable or disable the Link Power Management .
|
|||
This parameter can be set to ENABLE or DISABLE */ |
|||
|
|||
uint32_t battery_charging_active; /*!< Enable or disable Battery charging.
|
|||
This parameter can be set to ENABLE or DISABLE */ |
|||
void *pData; /*!< Pointer to upper stack Handler */ |
|||
|
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
void (* SOFCallback)(struct __PCD_HandleTypeDef *hpcd); /*!< USB OTG PCD SOF callback */ |
|||
void (* SetupStageCallback)(struct __PCD_HandleTypeDef *hpcd); /*!< USB OTG PCD Setup Stage callback */ |
|||
void (* ResetCallback)(struct __PCD_HandleTypeDef *hpcd); /*!< USB OTG PCD Reset callback */ |
|||
void (* SuspendCallback)(struct __PCD_HandleTypeDef *hpcd); /*!< USB OTG PCD Suspend callback */ |
|||
void (* ResumeCallback)(struct __PCD_HandleTypeDef *hpcd); /*!< USB OTG PCD Resume callback */ |
|||
void (* ConnectCallback)(struct __PCD_HandleTypeDef *hpcd); /*!< USB OTG PCD Connect callback */ |
|||
void (* DisconnectCallback)(struct __PCD_HandleTypeDef *hpcd); /*!< USB OTG PCD Disconnect callback */ |
|||
|
|||
void (* DataOutStageCallback)(struct __PCD_HandleTypeDef *hpcd, uint8_t epnum); /*!< USB OTG PCD Data OUT Stage callback */ |
|||
void (* DataInStageCallback)(struct __PCD_HandleTypeDef *hpcd, uint8_t epnum); /*!< USB OTG PCD Data IN Stage callback */ |
|||
void (* ISOOUTIncompleteCallback)(struct __PCD_HandleTypeDef *hpcd, uint8_t epnum); /*!< USB OTG PCD ISO OUT Incomplete callback */ |
|||
void (* ISOINIncompleteCallback)(struct __PCD_HandleTypeDef *hpcd, uint8_t epnum); /*!< USB OTG PCD ISO IN Incomplete callback */ |
|||
void (* BCDCallback)(struct __PCD_HandleTypeDef *hpcd, PCD_BCD_MsgTypeDef msg); /*!< USB OTG PCD BCD callback */ |
|||
void (* LPMCallback)(struct __PCD_HandleTypeDef *hpcd, PCD_LPM_MsgTypeDef msg); /*!< USB OTG PCD LPM callback */ |
|||
|
|||
void (* MspInitCallback)(struct __PCD_HandleTypeDef *hpcd); /*!< USB OTG PCD Msp Init callback */ |
|||
void (* MspDeInitCallback)(struct __PCD_HandleTypeDef *hpcd); /*!< USB OTG PCD Msp DeInit callback */ |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
} PCD_HandleTypeDef; |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* Include PCD HAL Extended module */ |
|||
#include "stm32f4xx_hal_pcd_ex.h" |
|||
|
|||
/* Exported constants --------------------------------------------------------*/ |
|||
/** @defgroup PCD_Exported_Constants PCD Exported Constants
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup PCD_Speed PCD Speed
|
|||
* @{ |
|||
*/ |
|||
#define PCD_SPEED_HIGH USBD_HS_SPEED |
|||
#define PCD_SPEED_HIGH_IN_FULL USBD_HSINFS_SPEED |
|||
#define PCD_SPEED_FULL USBD_FS_SPEED |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup PCD_PHY_Module PCD PHY Module
|
|||
* @{ |
|||
*/ |
|||
#define PCD_PHY_ULPI 1U |
|||
#define PCD_PHY_EMBEDDED 2U |
|||
#define PCD_PHY_UTMI 3U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup PCD_Error_Code_definition PCD Error Code definition
|
|||
* @brief PCD Error Code definition |
|||
* @{ |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
#define HAL_PCD_ERROR_INVALID_CALLBACK (0x00000010U) /*!< Invalid Callback error */ |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* Exported macros -----------------------------------------------------------*/ |
|||
/** @defgroup PCD_Exported_Macros PCD Exported Macros
|
|||
* @brief macros to handle interrupts and specific clock configurations |
|||
* @{ |
|||
*/ |
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
#define __HAL_PCD_ENABLE(__HANDLE__) (void)USB_EnableGlobalInt ((__HANDLE__)->Instance) |
|||
#define __HAL_PCD_DISABLE(__HANDLE__) (void)USB_DisableGlobalInt ((__HANDLE__)->Instance) |
|||
|
|||
#define __HAL_PCD_GET_FLAG(__HANDLE__, __INTERRUPT__) \ |
|||
((USB_ReadInterrupts((__HANDLE__)->Instance) & (__INTERRUPT__)) == (__INTERRUPT__)) |
|||
|
|||
#define __HAL_PCD_CLEAR_FLAG(__HANDLE__, __INTERRUPT__) (((__HANDLE__)->Instance->GINTSTS) &= (__INTERRUPT__)) |
|||
#define __HAL_PCD_IS_INVALID_INTERRUPT(__HANDLE__) (USB_ReadInterrupts((__HANDLE__)->Instance) == 0U) |
|||
|
|||
#define __HAL_PCD_UNGATE_PHYCLOCK(__HANDLE__) \ |
|||
*(__IO uint32_t *)((uint32_t)((__HANDLE__)->Instance) + USB_OTG_PCGCCTL_BASE) &= ~(USB_OTG_PCGCCTL_STOPCLK) |
|||
|
|||
#define __HAL_PCD_GATE_PHYCLOCK(__HANDLE__) \ |
|||
*(__IO uint32_t *)((uint32_t)((__HANDLE__)->Instance) + USB_OTG_PCGCCTL_BASE) |= USB_OTG_PCGCCTL_STOPCLK |
|||
|
|||
#define __HAL_PCD_IS_PHY_SUSPENDED(__HANDLE__) \ |
|||
((*(__IO uint32_t *)((uint32_t)((__HANDLE__)->Instance) + USB_OTG_PCGCCTL_BASE)) & 0x10U) |
|||
|
|||
#define __HAL_USB_OTG_HS_WAKEUP_EXTI_ENABLE_IT() EXTI->IMR |= (USB_OTG_HS_WAKEUP_EXTI_LINE) |
|||
#define __HAL_USB_OTG_HS_WAKEUP_EXTI_DISABLE_IT() EXTI->IMR &= ~(USB_OTG_HS_WAKEUP_EXTI_LINE) |
|||
#define __HAL_USB_OTG_HS_WAKEUP_EXTI_GET_FLAG() EXTI->PR & (USB_OTG_HS_WAKEUP_EXTI_LINE) |
|||
#define __HAL_USB_OTG_HS_WAKEUP_EXTI_CLEAR_FLAG() EXTI->PR = (USB_OTG_HS_WAKEUP_EXTI_LINE) |
|||
|
|||
#define __HAL_USB_OTG_HS_WAKEUP_EXTI_ENABLE_RISING_EDGE() \ |
|||
do { \ |
|||
EXTI->FTSR &= ~(USB_OTG_HS_WAKEUP_EXTI_LINE); \ |
|||
EXTI->RTSR |= USB_OTG_HS_WAKEUP_EXTI_LINE; \ |
|||
} while(0U) |
|||
#define __HAL_USB_OTG_FS_WAKEUP_EXTI_ENABLE_IT() EXTI->IMR |= USB_OTG_FS_WAKEUP_EXTI_LINE |
|||
#define __HAL_USB_OTG_FS_WAKEUP_EXTI_DISABLE_IT() EXTI->IMR &= ~(USB_OTG_FS_WAKEUP_EXTI_LINE) |
|||
#define __HAL_USB_OTG_FS_WAKEUP_EXTI_GET_FLAG() EXTI->PR & (USB_OTG_FS_WAKEUP_EXTI_LINE) |
|||
#define __HAL_USB_OTG_FS_WAKEUP_EXTI_CLEAR_FLAG() EXTI->PR = USB_OTG_FS_WAKEUP_EXTI_LINE |
|||
|
|||
#define __HAL_USB_OTG_FS_WAKEUP_EXTI_ENABLE_RISING_EDGE() \ |
|||
do { \ |
|||
EXTI->FTSR &= ~(USB_OTG_FS_WAKEUP_EXTI_LINE); \ |
|||
EXTI->RTSR |= USB_OTG_FS_WAKEUP_EXTI_LINE; \ |
|||
} while(0U) |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* Exported functions --------------------------------------------------------*/ |
|||
/** @addtogroup PCD_Exported_Functions PCD Exported Functions
|
|||
* @{ |
|||
*/ |
|||
|
|||
/* Initialization/de-initialization functions ********************************/ |
|||
/** @addtogroup PCD_Exported_Functions_Group1 Initialization and de-initialization functions
|
|||
* @{ |
|||
*/ |
|||
HAL_StatusTypeDef HAL_PCD_Init(PCD_HandleTypeDef *hpcd); |
|||
HAL_StatusTypeDef HAL_PCD_DeInit(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_MspInit(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_MspDeInit(PCD_HandleTypeDef *hpcd); |
|||
|
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
/** @defgroup HAL_PCD_Callback_ID_enumeration_definition HAL USB OTG PCD Callback ID enumeration definition
|
|||
* @brief HAL USB OTG PCD Callback ID enumeration definition |
|||
* @{ |
|||
*/ |
|||
typedef enum |
|||
{ |
|||
HAL_PCD_SOF_CB_ID = 0x01, /*!< USB PCD SOF callback ID */ |
|||
HAL_PCD_SETUPSTAGE_CB_ID = 0x02, /*!< USB PCD Setup Stage callback ID */ |
|||
HAL_PCD_RESET_CB_ID = 0x03, /*!< USB PCD Reset callback ID */ |
|||
HAL_PCD_SUSPEND_CB_ID = 0x04, /*!< USB PCD Suspend callback ID */ |
|||
HAL_PCD_RESUME_CB_ID = 0x05, /*!< USB PCD Resume callback ID */ |
|||
HAL_PCD_CONNECT_CB_ID = 0x06, /*!< USB PCD Connect callback ID */ |
|||
HAL_PCD_DISCONNECT_CB_ID = 0x07, /*!< USB PCD Disconnect callback ID */ |
|||
|
|||
HAL_PCD_MSPINIT_CB_ID = 0x08, /*!< USB PCD MspInit callback ID */ |
|||
HAL_PCD_MSPDEINIT_CB_ID = 0x09 /*!< USB PCD MspDeInit callback ID */ |
|||
|
|||
} HAL_PCD_CallbackIDTypeDef; |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup HAL_PCD_Callback_pointer_definition HAL USB OTG PCD Callback pointer definition
|
|||
* @brief HAL USB OTG PCD Callback pointer definition |
|||
* @{ |
|||
*/ |
|||
|
|||
typedef void (*pPCD_CallbackTypeDef)(PCD_HandleTypeDef *hpcd); /*!< pointer to a common USB OTG PCD callback function */ |
|||
typedef void (*pPCD_DataOutStageCallbackTypeDef)(PCD_HandleTypeDef *hpcd, uint8_t epnum); /*!< pointer to USB OTG PCD Data OUT Stage callback */ |
|||
typedef void (*pPCD_DataInStageCallbackTypeDef)(PCD_HandleTypeDef *hpcd, uint8_t epnum); /*!< pointer to USB OTG PCD Data IN Stage callback */ |
|||
typedef void (*pPCD_IsoOutIncpltCallbackTypeDef)(PCD_HandleTypeDef *hpcd, uint8_t epnum); /*!< pointer to USB OTG PCD ISO OUT Incomplete callback */ |
|||
typedef void (*pPCD_IsoInIncpltCallbackTypeDef)(PCD_HandleTypeDef *hpcd, uint8_t epnum); /*!< pointer to USB OTG PCD ISO IN Incomplete callback */ |
|||
typedef void (*pPCD_LpmCallbackTypeDef)(PCD_HandleTypeDef *hpcd, PCD_LPM_MsgTypeDef msg); /*!< pointer to USB OTG PCD LPM callback */ |
|||
typedef void (*pPCD_BcdCallbackTypeDef)(PCD_HandleTypeDef *hpcd, PCD_BCD_MsgTypeDef msg); /*!< pointer to USB OTG PCD BCD callback */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_RegisterCallback(PCD_HandleTypeDef *hpcd, HAL_PCD_CallbackIDTypeDef CallbackID, |
|||
pPCD_CallbackTypeDef pCallback); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_UnRegisterCallback(PCD_HandleTypeDef *hpcd, HAL_PCD_CallbackIDTypeDef CallbackID); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_RegisterDataOutStageCallback(PCD_HandleTypeDef *hpcd, |
|||
pPCD_DataOutStageCallbackTypeDef pCallback); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_UnRegisterDataOutStageCallback(PCD_HandleTypeDef *hpcd); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_RegisterDataInStageCallback(PCD_HandleTypeDef *hpcd, |
|||
pPCD_DataInStageCallbackTypeDef pCallback); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_UnRegisterDataInStageCallback(PCD_HandleTypeDef *hpcd); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_RegisterIsoOutIncpltCallback(PCD_HandleTypeDef *hpcd, |
|||
pPCD_IsoOutIncpltCallbackTypeDef pCallback); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_UnRegisterIsoOutIncpltCallback(PCD_HandleTypeDef *hpcd); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_RegisterIsoInIncpltCallback(PCD_HandleTypeDef *hpcd, |
|||
pPCD_IsoInIncpltCallbackTypeDef pCallback); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_UnRegisterIsoInIncpltCallback(PCD_HandleTypeDef *hpcd); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_RegisterBcdCallback(PCD_HandleTypeDef *hpcd, pPCD_BcdCallbackTypeDef pCallback); |
|||
HAL_StatusTypeDef HAL_PCD_UnRegisterBcdCallback(PCD_HandleTypeDef *hpcd); |
|||
|
|||
HAL_StatusTypeDef HAL_PCD_RegisterLpmCallback(PCD_HandleTypeDef *hpcd, pPCD_LpmCallbackTypeDef pCallback); |
|||
HAL_StatusTypeDef HAL_PCD_UnRegisterLpmCallback(PCD_HandleTypeDef *hpcd); |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* I/O operation functions ***************************************************/ |
|||
/* Non-Blocking mode: Interrupt */ |
|||
/** @addtogroup PCD_Exported_Functions_Group2 Input and Output operation functions
|
|||
* @{ |
|||
*/ |
|||
HAL_StatusTypeDef HAL_PCD_Start(PCD_HandleTypeDef *hpcd); |
|||
HAL_StatusTypeDef HAL_PCD_Stop(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_IRQHandler(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_WKUP_IRQHandler(PCD_HandleTypeDef *hpcd); |
|||
|
|||
void HAL_PCD_SOFCallback(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_SetupStageCallback(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_ResetCallback(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_SuspendCallback(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_ResumeCallback(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_ConnectCallback(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCD_DisconnectCallback(PCD_HandleTypeDef *hpcd); |
|||
|
|||
void HAL_PCD_DataOutStageCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum); |
|||
void HAL_PCD_DataInStageCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum); |
|||
void HAL_PCD_ISOOUTIncompleteCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum); |
|||
void HAL_PCD_ISOINIncompleteCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum); |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* Peripheral Control functions **********************************************/ |
|||
/** @addtogroup PCD_Exported_Functions_Group3 Peripheral Control functions
|
|||
* @{ |
|||
*/ |
|||
HAL_StatusTypeDef HAL_PCD_DevConnect(PCD_HandleTypeDef *hpcd); |
|||
HAL_StatusTypeDef HAL_PCD_DevDisconnect(PCD_HandleTypeDef *hpcd); |
|||
HAL_StatusTypeDef HAL_PCD_SetAddress(PCD_HandleTypeDef *hpcd, uint8_t address); |
|||
HAL_StatusTypeDef HAL_PCD_EP_Open(PCD_HandleTypeDef *hpcd, uint8_t ep_addr, uint16_t ep_mps, uint8_t ep_type); |
|||
HAL_StatusTypeDef HAL_PCD_EP_Close(PCD_HandleTypeDef *hpcd, uint8_t ep_addr); |
|||
HAL_StatusTypeDef HAL_PCD_EP_Receive(PCD_HandleTypeDef *hpcd, uint8_t ep_addr, uint8_t *pBuf, uint32_t len); |
|||
HAL_StatusTypeDef HAL_PCD_EP_Transmit(PCD_HandleTypeDef *hpcd, uint8_t ep_addr, uint8_t *pBuf, uint32_t len); |
|||
HAL_StatusTypeDef HAL_PCD_EP_SetStall(PCD_HandleTypeDef *hpcd, uint8_t ep_addr); |
|||
HAL_StatusTypeDef HAL_PCD_EP_ClrStall(PCD_HandleTypeDef *hpcd, uint8_t ep_addr); |
|||
HAL_StatusTypeDef HAL_PCD_EP_Flush(PCD_HandleTypeDef *hpcd, uint8_t ep_addr); |
|||
HAL_StatusTypeDef HAL_PCD_EP_Abort(PCD_HandleTypeDef *hpcd, uint8_t ep_addr); |
|||
HAL_StatusTypeDef HAL_PCD_ActivateRemoteWakeup(PCD_HandleTypeDef *hpcd); |
|||
HAL_StatusTypeDef HAL_PCD_DeActivateRemoteWakeup(PCD_HandleTypeDef *hpcd); |
|||
HAL_StatusTypeDef HAL_PCD_SetTestMode(PCD_HandleTypeDef *hpcd, uint8_t testmode); |
|||
|
|||
uint32_t HAL_PCD_EP_GetRxCount(PCD_HandleTypeDef *hpcd, uint8_t ep_addr); |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* Peripheral State functions ************************************************/ |
|||
/** @addtogroup PCD_Exported_Functions_Group4 Peripheral State functions
|
|||
* @{ |
|||
*/ |
|||
PCD_StateTypeDef HAL_PCD_GetState(PCD_HandleTypeDef *hpcd); |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* Private constants ---------------------------------------------------------*/ |
|||
/** @defgroup PCD_Private_Constants PCD Private Constants
|
|||
* @{ |
|||
*/ |
|||
/** @defgroup USB_EXTI_Line_Interrupt USB EXTI line interrupt
|
|||
* @{ |
|||
*/ |
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
#define USB_OTG_FS_WAKEUP_EXTI_LINE (0x1U << 18) /*!< USB FS EXTI Line WakeUp Interrupt */ |
|||
#define USB_OTG_HS_WAKEUP_EXTI_LINE (0x1U << 20) /*!< USB HS EXTI Line WakeUp Interrupt */ |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
#ifndef USB_OTG_DOEPINT_OTEPSPR |
|||
#define USB_OTG_DOEPINT_OTEPSPR (0x1UL << 5) /*!< Status Phase Received interrupt */ |
|||
#endif /* defined USB_OTG_DOEPINT_OTEPSPR */ |
|||
|
|||
#ifndef USB_OTG_DOEPMSK_OTEPSPRM |
|||
#define USB_OTG_DOEPMSK_OTEPSPRM (0x1UL << 5) /*!< Setup Packet Received interrupt mask */ |
|||
#endif /* defined USB_OTG_DOEPMSK_OTEPSPRM */ |
|||
|
|||
#ifndef USB_OTG_DOEPINT_NAK |
|||
#define USB_OTG_DOEPINT_NAK (0x1UL << 13) /*!< NAK interrupt */ |
|||
#endif /* defined USB_OTG_DOEPINT_NAK */ |
|||
|
|||
#ifndef USB_OTG_DOEPMSK_NAKM |
|||
#define USB_OTG_DOEPMSK_NAKM (0x1UL << 13) /*!< OUT Packet NAK interrupt mask */ |
|||
#endif /* defined USB_OTG_DOEPMSK_NAKM */ |
|||
|
|||
#ifndef USB_OTG_DOEPINT_STPKTRX |
|||
#define USB_OTG_DOEPINT_STPKTRX (0x1UL << 15) /*!< Setup Packet Received interrupt */ |
|||
#endif /* defined USB_OTG_DOEPINT_STPKTRX */ |
|||
|
|||
#ifndef USB_OTG_DOEPMSK_NYETM |
|||
#define USB_OTG_DOEPMSK_NYETM (0x1UL << 14) /*!< Setup Packet Received interrupt mask */ |
|||
#endif /* defined USB_OTG_DOEPMSK_NYETM */ |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
/* Private macros ------------------------------------------------------------*/ |
|||
/** @defgroup PCD_Private_Macros PCD Private Macros
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* STM32F4xx_HAL_PCD_H */ |
@ -0,0 +1,88 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file stm32f4xx_hal_pcd_ex.h |
|||
* @author MCD Application Team |
|||
* @brief Header file of PCD HAL Extension module. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2016 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef STM32F4xx_HAL_PCD_EX_H |
|||
#define STM32F4xx_HAL_PCD_EX_H |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif /* __cplusplus */ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "stm32f4xx_hal_def.h" |
|||
|
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
/** @addtogroup STM32F4xx_HAL_Driver
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @addtogroup PCDEx
|
|||
* @{ |
|||
*/ |
|||
/* Exported types ------------------------------------------------------------*/ |
|||
/* Exported constants --------------------------------------------------------*/ |
|||
/* Exported macros -----------------------------------------------------------*/ |
|||
/* Exported functions --------------------------------------------------------*/ |
|||
/** @addtogroup PCDEx_Exported_Functions PCDEx Exported Functions
|
|||
* @{ |
|||
*/ |
|||
/** @addtogroup PCDEx_Exported_Functions_Group1 Peripheral Control functions
|
|||
* @{ |
|||
*/ |
|||
|
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
HAL_StatusTypeDef HAL_PCDEx_SetTxFiFo(PCD_HandleTypeDef *hpcd, uint8_t fifo, uint16_t size); |
|||
HAL_StatusTypeDef HAL_PCDEx_SetRxFiFo(PCD_HandleTypeDef *hpcd, uint16_t size); |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
#if defined(STM32F446xx) || defined(STM32F469xx) || defined(STM32F479xx) || defined(STM32F412Zx) || defined(STM32F412Vx) || defined(STM32F412Rx) || defined(STM32F412Cx) || defined(STM32F413xx) || defined(STM32F423xx) |
|||
HAL_StatusTypeDef HAL_PCDEx_ActivateLPM(PCD_HandleTypeDef *hpcd); |
|||
HAL_StatusTypeDef HAL_PCDEx_DeActivateLPM(PCD_HandleTypeDef *hpcd); |
|||
#endif /* defined(STM32F446xx) || defined(STM32F469xx) || defined(STM32F479xx) || defined(STM32F412Zx) || defined(STM32F412Vx) || defined(STM32F412Rx) || defined(STM32F412Cx) || defined(STM32F413xx) || defined(STM32F423xx) */ |
|||
#if defined(STM32F412Zx) || defined(STM32F412Vx) || defined(STM32F412Rx) || defined(STM32F412Cx) || defined(STM32F413xx) || defined(STM32F423xx) |
|||
HAL_StatusTypeDef HAL_PCDEx_ActivateBCD(PCD_HandleTypeDef *hpcd); |
|||
HAL_StatusTypeDef HAL_PCDEx_DeActivateBCD(PCD_HandleTypeDef *hpcd); |
|||
void HAL_PCDEx_BCD_VBUSDetect(PCD_HandleTypeDef *hpcd); |
|||
#endif /* defined(STM32F412Zx) || defined(STM32F412Vx) || defined(STM32F412Rx) || defined(STM32F412Cx) || defined(STM32F413xx) || defined(STM32F423xx) */ |
|||
void HAL_PCDEx_LPM_Callback(PCD_HandleTypeDef *hpcd, PCD_LPM_MsgTypeDef msg); |
|||
void HAL_PCDEx_BCD_Callback(PCD_HandleTypeDef *hpcd, PCD_BCD_MsgTypeDef msg); |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif /* __cplusplus */ |
|||
|
|||
|
|||
#endif /* STM32F4xx_HAL_PCD_EX_H */ |
@ -0,0 +1,536 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file stm32f4xx_ll_usb.h |
|||
* @author MCD Application Team |
|||
* @brief Header file of USB Low Layer HAL module. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2016 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef STM32F4xx_LL_USB_H |
|||
#define STM32F4xx_LL_USB_H |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif /* __cplusplus */ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "stm32f4xx_hal_def.h" |
|||
|
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
/** @addtogroup STM32F4xx_HAL_Driver
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @addtogroup USB_LL
|
|||
* @{ |
|||
*/ |
|||
|
|||
/* Exported types ------------------------------------------------------------*/ |
|||
|
|||
/**
|
|||
* @brief USB Mode definition |
|||
*/ |
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
|
|||
typedef enum |
|||
{ |
|||
USB_DEVICE_MODE = 0, |
|||
USB_HOST_MODE = 1, |
|||
USB_DRD_MODE = 2 |
|||
} USB_OTG_ModeTypeDef; |
|||
|
|||
/**
|
|||
* @brief URB States definition |
|||
*/ |
|||
typedef enum |
|||
{ |
|||
URB_IDLE = 0, |
|||
URB_DONE, |
|||
URB_NOTREADY, |
|||
URB_NYET, |
|||
URB_ERROR, |
|||
URB_STALL |
|||
} USB_OTG_URBStateTypeDef; |
|||
|
|||
/**
|
|||
* @brief Host channel States definition |
|||
*/ |
|||
typedef enum |
|||
{ |
|||
HC_IDLE = 0, |
|||
HC_XFRC, |
|||
HC_HALTED, |
|||
HC_NAK, |
|||
HC_NYET, |
|||
HC_STALL, |
|||
HC_XACTERR, |
|||
HC_BBLERR, |
|||
HC_DATATGLERR |
|||
} USB_OTG_HCStateTypeDef; |
|||
|
|||
|
|||
/**
|
|||
* @brief USB Instance Initialization Structure definition |
|||
*/ |
|||
typedef struct |
|||
{ |
|||
uint32_t dev_endpoints; /*!< Device Endpoints number.
|
|||
This parameter depends on the used USB core. |
|||
This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ |
|||
|
|||
uint32_t Host_channels; /*!< Host Channels number.
|
|||
This parameter Depends on the used USB core. |
|||
This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ |
|||
|
|||
uint32_t speed; /*!< USB Core speed.
|
|||
This parameter can be any value of @ref PCD_Speed/HCD_Speed |
|||
(HCD_SPEED_xxx, HCD_SPEED_xxx) */ |
|||
|
|||
uint32_t dma_enable; /*!< Enable or disable of the USB embedded DMA used only for OTG HS. */ |
|||
|
|||
uint32_t ep0_mps; /*!< Set the Endpoint 0 Max Packet size. */ |
|||
|
|||
uint32_t phy_itface; /*!< Select the used PHY interface.
|
|||
This parameter can be any value of @ref PCD_PHY_Module/HCD_PHY_Module */ |
|||
|
|||
uint32_t Sof_enable; /*!< Enable or disable the output of the SOF signal. */ |
|||
|
|||
uint32_t low_power_enable; /*!< Enable or disable the low power mode. */ |
|||
|
|||
uint32_t lpm_enable; /*!< Enable or disable Link Power Management. */ |
|||
|
|||
uint32_t battery_charging_enable; /*!< Enable or disable Battery charging. */ |
|||
|
|||
uint32_t vbus_sensing_enable; /*!< Enable or disable the VBUS Sensing feature. */ |
|||
|
|||
uint32_t use_dedicated_ep1; /*!< Enable or disable the use of the dedicated EP1 interrupt. */ |
|||
|
|||
uint32_t use_external_vbus; /*!< Enable or disable the use of the external VBUS. */ |
|||
|
|||
} USB_OTG_CfgTypeDef; |
|||
|
|||
typedef struct |
|||
{ |
|||
uint8_t num; /*!< Endpoint number
|
|||
This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ |
|||
|
|||
uint8_t is_in; /*!< Endpoint direction
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ |
|||
|
|||
uint8_t is_stall; /*!< Endpoint stall condition
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ |
|||
|
|||
uint8_t is_iso_incomplete; /*!< Endpoint isoc condition
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ |
|||
|
|||
uint8_t type; /*!< Endpoint type
|
|||
This parameter can be any value of @ref USB_LL_EP_Type */ |
|||
|
|||
uint8_t data_pid_start; /*!< Initial data PID
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ |
|||
|
|||
uint8_t even_odd_frame; /*!< IFrame parity
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ |
|||
|
|||
uint16_t tx_fifo_num; /*!< Transmission FIFO number
|
|||
This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ |
|||
|
|||
uint32_t maxpacket; /*!< Endpoint Max packet size
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 64KB */ |
|||
|
|||
uint8_t *xfer_buff; /*!< Pointer to transfer buffer */ |
|||
|
|||
uint32_t dma_addr; /*!< 32 bits aligned transfer buffer address */ |
|||
|
|||
uint32_t xfer_len; /*!< Current transfer length */ |
|||
|
|||
uint32_t xfer_size; /*!< requested transfer size */ |
|||
|
|||
uint32_t xfer_count; /*!< Partial transfer length in case of multi packet transfer */ |
|||
} USB_OTG_EPTypeDef; |
|||
|
|||
typedef struct |
|||
{ |
|||
uint8_t dev_addr; /*!< USB device address.
|
|||
This parameter must be a number between Min_Data = 1 and Max_Data = 255 */ |
|||
|
|||
uint8_t ch_num; /*!< Host channel number.
|
|||
This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ |
|||
|
|||
uint8_t ep_num; /*!< Endpoint number.
|
|||
This parameter must be a number between Min_Data = 1 and Max_Data = 15 */ |
|||
|
|||
uint8_t ep_is_in; /*!< Endpoint direction
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ |
|||
|
|||
uint8_t speed; /*!< USB Host Channel speed.
|
|||
This parameter can be any value of @ref HCD_Device_Speed: |
|||
(HCD_DEVICE_SPEED_xxx) */ |
|||
|
|||
uint8_t do_ping; /*!< Enable or disable the use of the PING protocol for HS mode. */ |
|||
|
|||
uint8_t process_ping; /*!< Execute the PING protocol for HS mode. */ |
|||
|
|||
uint8_t ep_type; /*!< Endpoint Type.
|
|||
This parameter can be any value of @ref USB_LL_EP_Type */ |
|||
|
|||
uint16_t max_packet; /*!< Endpoint Max packet size.
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 64KB */ |
|||
|
|||
uint8_t data_pid; /*!< Initial data PID.
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ |
|||
|
|||
uint8_t *xfer_buff; /*!< Pointer to transfer buffer. */ |
|||
|
|||
uint32_t XferSize; /*!< OTG Channel transfer size. */ |
|||
|
|||
uint32_t xfer_len; /*!< Current transfer length. */ |
|||
|
|||
uint32_t xfer_count; /*!< Partial transfer length in case of multi packet transfer. */ |
|||
|
|||
uint8_t toggle_in; /*!< IN transfer current toggle flag.
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ |
|||
|
|||
uint8_t toggle_out; /*!< OUT transfer current toggle flag
|
|||
This parameter must be a number between Min_Data = 0 and Max_Data = 1 */ |
|||
|
|||
uint32_t dma_addr; /*!< 32 bits aligned transfer buffer address. */ |
|||
|
|||
uint32_t ErrCnt; /*!< Host channel error count. */ |
|||
|
|||
USB_OTG_URBStateTypeDef urb_state; /*!< URB state.
|
|||
This parameter can be any value of @ref USB_OTG_URBStateTypeDef */ |
|||
|
|||
USB_OTG_HCStateTypeDef state; /*!< Host Channel state.
|
|||
This parameter can be any value of @ref USB_OTG_HCStateTypeDef */ |
|||
} USB_OTG_HCTypeDef; |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
|
|||
/* Exported constants --------------------------------------------------------*/ |
|||
|
|||
/** @defgroup PCD_Exported_Constants PCD Exported Constants
|
|||
* @{ |
|||
*/ |
|||
|
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
/** @defgroup USB_OTG_CORE VERSION ID
|
|||
* @{ |
|||
*/ |
|||
#define USB_OTG_CORE_ID_300A 0x4F54300AU |
|||
#define USB_OTG_CORE_ID_310A 0x4F54310AU |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_Core_Mode_ USB Core Mode
|
|||
* @{ |
|||
*/ |
|||
#define USB_OTG_MODE_DEVICE 0U |
|||
#define USB_OTG_MODE_HOST 1U |
|||
#define USB_OTG_MODE_DRD 2U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL Device Speed
|
|||
* @{ |
|||
*/ |
|||
#define USBD_HS_SPEED 0U |
|||
#define USBD_HSINFS_SPEED 1U |
|||
#define USBH_HS_SPEED 0U |
|||
#define USBD_FS_SPEED 2U |
|||
#define USBH_FSLS_SPEED 1U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_Core_Speed USB Low Layer Core Speed
|
|||
* @{ |
|||
*/ |
|||
#define USB_OTG_SPEED_HIGH 0U |
|||
#define USB_OTG_SPEED_HIGH_IN_FULL 1U |
|||
#define USB_OTG_SPEED_FULL 3U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_Core_PHY USB Low Layer Core PHY
|
|||
* @{ |
|||
*/ |
|||
#define USB_OTG_ULPI_PHY 1U |
|||
#define USB_OTG_EMBEDDED_PHY 2U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_Turnaround_Timeout Turnaround Timeout Value
|
|||
* @{ |
|||
*/ |
|||
#ifndef USBD_HS_TRDT_VALUE |
|||
#define USBD_HS_TRDT_VALUE 9U |
|||
#endif /* USBD_HS_TRDT_VALUE */ |
|||
#ifndef USBD_FS_TRDT_VALUE |
|||
#define USBD_FS_TRDT_VALUE 5U |
|||
#define USBD_DEFAULT_TRDT_VALUE 9U |
|||
#endif /* USBD_HS_TRDT_VALUE */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_Core_MPS USB Low Layer Core MPS
|
|||
* @{ |
|||
*/ |
|||
#define USB_OTG_HS_MAX_PACKET_SIZE 512U |
|||
#define USB_OTG_FS_MAX_PACKET_SIZE 64U |
|||
#define USB_OTG_MAX_EP0_SIZE 64U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_Core_PHY_Frequency USB Low Layer Core PHY Frequency
|
|||
* @{ |
|||
*/ |
|||
#define DSTS_ENUMSPD_HS_PHY_30MHZ_OR_60MHZ (0U << 1) |
|||
#define DSTS_ENUMSPD_FS_PHY_30MHZ_OR_60MHZ (1U << 1) |
|||
#define DSTS_ENUMSPD_FS_PHY_48MHZ (3U << 1) |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_CORE_Frame_Interval USB Low Layer Core Frame Interval
|
|||
* @{ |
|||
*/ |
|||
#define DCFG_FRAME_INTERVAL_80 0U |
|||
#define DCFG_FRAME_INTERVAL_85 1U |
|||
#define DCFG_FRAME_INTERVAL_90 2U |
|||
#define DCFG_FRAME_INTERVAL_95 3U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_EP0_MPS USB Low Layer EP0 MPS
|
|||
* @{ |
|||
*/ |
|||
#define EP_MPS_64 0U |
|||
#define EP_MPS_32 1U |
|||
#define EP_MPS_16 2U |
|||
#define EP_MPS_8 3U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_EP_Speed USB Low Layer EP Speed
|
|||
* @{ |
|||
*/ |
|||
#define EP_SPEED_LOW 0U |
|||
#define EP_SPEED_FULL 1U |
|||
#define EP_SPEED_HIGH 2U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_EP_Type USB Low Layer EP Type
|
|||
* @{ |
|||
*/ |
|||
#define EP_TYPE_CTRL 0U |
|||
#define EP_TYPE_ISOC 1U |
|||
#define EP_TYPE_BULK 2U |
|||
#define EP_TYPE_INTR 3U |
|||
#define EP_TYPE_MSK 3U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_STS_Defines USB Low Layer STS Defines
|
|||
* @{ |
|||
*/ |
|||
#define STS_GOUT_NAK 1U |
|||
#define STS_DATA_UPDT 2U |
|||
#define STS_XFER_COMP 3U |
|||
#define STS_SETUP_COMP 4U |
|||
#define STS_SETUP_UPDT 6U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_HCFG_SPEED_Defines USB Low Layer HCFG Speed Defines
|
|||
* @{ |
|||
*/ |
|||
#define HCFG_30_60_MHZ 0U |
|||
#define HCFG_48_MHZ 1U |
|||
#define HCFG_6_MHZ 2U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_LL_HPRT0_PRTSPD_SPEED_Defines USB Low Layer HPRT0 PRTSPD Speed Defines
|
|||
* @{ |
|||
*/ |
|||
#define HPRT0_PRTSPD_HIGH_SPEED 0U |
|||
#define HPRT0_PRTSPD_FULL_SPEED 1U |
|||
#define HPRT0_PRTSPD_LOW_SPEED 2U |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#define HCCHAR_CTRL 0U |
|||
#define HCCHAR_ISOC 1U |
|||
#define HCCHAR_BULK 2U |
|||
#define HCCHAR_INTR 3U |
|||
|
|||
#define HC_PID_DATA0 0U |
|||
#define HC_PID_DATA2 1U |
|||
#define HC_PID_DATA1 2U |
|||
#define HC_PID_SETUP 3U |
|||
|
|||
#define GRXSTS_PKTSTS_IN 2U |
|||
#define GRXSTS_PKTSTS_IN_XFER_COMP 3U |
|||
#define GRXSTS_PKTSTS_DATA_TOGGLE_ERR 5U |
|||
#define GRXSTS_PKTSTS_CH_HALTED 7U |
|||
|
|||
#define TEST_J 1U |
|||
#define TEST_K 2U |
|||
#define TEST_SE0_NAK 3U |
|||
#define TEST_PACKET 4U |
|||
#define TEST_FORCE_EN 5U |
|||
|
|||
#define USBx_PCGCCTL *(__IO uint32_t *)((uint32_t)USBx_BASE + USB_OTG_PCGCCTL_BASE) |
|||
#define USBx_HPRT0 *(__IO uint32_t *)((uint32_t)USBx_BASE + USB_OTG_HOST_PORT_BASE) |
|||
|
|||
#define USBx_DEVICE ((USB_OTG_DeviceTypeDef *)(USBx_BASE + USB_OTG_DEVICE_BASE)) |
|||
#define USBx_INEP(i) ((USB_OTG_INEndpointTypeDef *)(USBx_BASE\ |
|||
+ USB_OTG_IN_ENDPOINT_BASE + ((i) * USB_OTG_EP_REG_SIZE))) |
|||
|
|||
#define USBx_OUTEP(i) ((USB_OTG_OUTEndpointTypeDef *)(USBx_BASE\ |
|||
+ USB_OTG_OUT_ENDPOINT_BASE + ((i) * USB_OTG_EP_REG_SIZE))) |
|||
|
|||
#define USBx_DFIFO(i) *(__IO uint32_t *)(USBx_BASE + USB_OTG_FIFO_BASE + ((i) * USB_OTG_FIFO_SIZE)) |
|||
|
|||
#define USBx_HOST ((USB_OTG_HostTypeDef *)(USBx_BASE + USB_OTG_HOST_BASE)) |
|||
#define USBx_HC(i) ((USB_OTG_HostChannelTypeDef *)(USBx_BASE\ |
|||
+ USB_OTG_HOST_CHANNEL_BASE\ |
|||
+ ((i) * USB_OTG_HOST_CHANNEL_SIZE))) |
|||
|
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
#define EP_ADDR_MSK 0xFU |
|||
|
|||
#ifndef USE_USB_DOUBLE_BUFFER |
|||
#define USE_USB_DOUBLE_BUFFER 1U |
|||
#endif /* USE_USB_DOUBLE_BUFFER */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* Exported macro ------------------------------------------------------------*/ |
|||
/** @defgroup USB_LL_Exported_Macros USB Low Layer Exported Macros
|
|||
* @{ |
|||
*/ |
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
#define USB_MASK_INTERRUPT(__INSTANCE__, __INTERRUPT__) ((__INSTANCE__)->GINTMSK &= ~(__INTERRUPT__)) |
|||
#define USB_UNMASK_INTERRUPT(__INSTANCE__, __INTERRUPT__) ((__INSTANCE__)->GINTMSK |= (__INTERRUPT__)) |
|||
|
|||
#define CLEAR_IN_EP_INTR(__EPNUM__, __INTERRUPT__) (USBx_INEP(__EPNUM__)->DIEPINT = (__INTERRUPT__)) |
|||
#define CLEAR_OUT_EP_INTR(__EPNUM__, __INTERRUPT__) (USBx_OUTEP(__EPNUM__)->DOEPINT = (__INTERRUPT__)) |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* Exported functions --------------------------------------------------------*/ |
|||
/** @addtogroup USB_LL_Exported_Functions USB Low Layer Exported Functions
|
|||
* @{ |
|||
*/ |
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
HAL_StatusTypeDef USB_CoreInit(USB_OTG_GlobalTypeDef *USBx, USB_OTG_CfgTypeDef cfg); |
|||
HAL_StatusTypeDef USB_DevInit(USB_OTG_GlobalTypeDef *USBx, USB_OTG_CfgTypeDef cfg); |
|||
HAL_StatusTypeDef USB_EnableGlobalInt(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_DisableGlobalInt(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_SetTurnaroundTime(USB_OTG_GlobalTypeDef *USBx, uint32_t hclk, uint8_t speed); |
|||
HAL_StatusTypeDef USB_SetCurrentMode(USB_OTG_GlobalTypeDef *USBx, USB_OTG_ModeTypeDef mode); |
|||
HAL_StatusTypeDef USB_SetDevSpeed(USB_OTG_GlobalTypeDef *USBx, uint8_t speed); |
|||
HAL_StatusTypeDef USB_FlushRxFifo(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_FlushTxFifo(USB_OTG_GlobalTypeDef *USBx, uint32_t num); |
|||
HAL_StatusTypeDef USB_ActivateEndpoint(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); |
|||
HAL_StatusTypeDef USB_DeactivateEndpoint(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); |
|||
HAL_StatusTypeDef USB_ActivateDedicatedEndpoint(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); |
|||
HAL_StatusTypeDef USB_DeactivateDedicatedEndpoint(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); |
|||
HAL_StatusTypeDef USB_EPStartXfer(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep, uint8_t dma); |
|||
HAL_StatusTypeDef USB_EP0StartXfer(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep, uint8_t dma); |
|||
HAL_StatusTypeDef USB_WritePacket(USB_OTG_GlobalTypeDef *USBx, uint8_t *src, |
|||
uint8_t ch_ep_num, uint16_t len, uint8_t dma); |
|||
|
|||
void *USB_ReadPacket(USB_OTG_GlobalTypeDef *USBx, uint8_t *dest, uint16_t len); |
|||
HAL_StatusTypeDef USB_EPSetStall(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); |
|||
HAL_StatusTypeDef USB_EPClearStall(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); |
|||
HAL_StatusTypeDef USB_EPStopXfer(USB_OTG_GlobalTypeDef *USBx, USB_OTG_EPTypeDef *ep); |
|||
HAL_StatusTypeDef USB_SetDevAddress(USB_OTG_GlobalTypeDef *USBx, uint8_t address); |
|||
HAL_StatusTypeDef USB_DevConnect(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_DevDisconnect(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_StopDevice(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_ActivateSetup(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_EP0_OutStart(USB_OTG_GlobalTypeDef *USBx, uint8_t dma, uint8_t *psetup); |
|||
uint8_t USB_GetDevSpeed(USB_OTG_GlobalTypeDef *USBx); |
|||
uint32_t USB_GetMode(USB_OTG_GlobalTypeDef *USBx); |
|||
uint32_t USB_ReadInterrupts(USB_OTG_GlobalTypeDef *USBx); |
|||
uint32_t USB_ReadDevAllOutEpInterrupt(USB_OTG_GlobalTypeDef *USBx); |
|||
uint32_t USB_ReadDevOutEPInterrupt(USB_OTG_GlobalTypeDef *USBx, uint8_t epnum); |
|||
uint32_t USB_ReadDevAllInEpInterrupt(USB_OTG_GlobalTypeDef *USBx); |
|||
uint32_t USB_ReadDevInEPInterrupt(USB_OTG_GlobalTypeDef *USBx, uint8_t epnum); |
|||
void USB_ClearInterrupts(USB_OTG_GlobalTypeDef *USBx, uint32_t interrupt); |
|||
|
|||
HAL_StatusTypeDef USB_HostInit(USB_OTG_GlobalTypeDef *USBx, USB_OTG_CfgTypeDef cfg); |
|||
HAL_StatusTypeDef USB_InitFSLSPClkSel(USB_OTG_GlobalTypeDef *USBx, uint8_t freq); |
|||
HAL_StatusTypeDef USB_ResetPort(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_DriveVbus(USB_OTG_GlobalTypeDef *USBx, uint8_t state); |
|||
uint32_t USB_GetHostSpeed(USB_OTG_GlobalTypeDef *USBx); |
|||
uint32_t USB_GetCurrentFrame(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_HC_Init(USB_OTG_GlobalTypeDef *USBx, uint8_t ch_num, |
|||
uint8_t epnum, uint8_t dev_address, uint8_t speed, |
|||
uint8_t ep_type, uint16_t mps); |
|||
HAL_StatusTypeDef USB_HC_StartXfer(USB_OTG_GlobalTypeDef *USBx, |
|||
USB_OTG_HCTypeDef *hc, uint8_t dma); |
|||
|
|||
uint32_t USB_HC_ReadInterrupt(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_HC_Halt(USB_OTG_GlobalTypeDef *USBx, uint8_t hc_num); |
|||
HAL_StatusTypeDef USB_DoPing(USB_OTG_GlobalTypeDef *USBx, uint8_t ch_num); |
|||
HAL_StatusTypeDef USB_StopHost(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_ActivateRemoteWakeup(USB_OTG_GlobalTypeDef *USBx); |
|||
HAL_StatusTypeDef USB_DeActivateRemoteWakeup(USB_OTG_GlobalTypeDef *USBx); |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif /* __cplusplus */ |
|||
|
|||
|
|||
#endif /* STM32F4xx_LL_USB_H */ |
File diff suppressed because it is too large
@ -0,0 +1,341 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file stm32f4xx_hal_pcd_ex.c |
|||
* @author MCD Application Team |
|||
* @brief PCD Extended HAL module driver. |
|||
* This file provides firmware functions to manage the following |
|||
* functionalities of the USB Peripheral Controller: |
|||
* + Extended features functions |
|||
* |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2016 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "stm32f4xx_hal.h" |
|||
|
|||
/** @addtogroup STM32F4xx_HAL_Driver
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup PCDEx PCDEx
|
|||
* @brief PCD Extended HAL module driver |
|||
* @{ |
|||
*/ |
|||
|
|||
#ifdef HAL_PCD_MODULE_ENABLED |
|||
|
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
/* Private types -------------------------------------------------------------*/ |
|||
/* Private variables ---------------------------------------------------------*/ |
|||
/* Private constants ---------------------------------------------------------*/ |
|||
/* Private macros ------------------------------------------------------------*/ |
|||
/* Private functions ---------------------------------------------------------*/ |
|||
/* Exported functions --------------------------------------------------------*/ |
|||
|
|||
/** @defgroup PCDEx_Exported_Functions PCDEx Exported Functions
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup PCDEx_Exported_Functions_Group1 Peripheral Control functions
|
|||
* @brief PCDEx control functions |
|||
* |
|||
@verbatim |
|||
=============================================================================== |
|||
##### Extended features functions ##### |
|||
=============================================================================== |
|||
[..] This section provides functions allowing to: |
|||
(+) Update FIFO configuration |
|||
|
|||
@endverbatim |
|||
* @{ |
|||
*/ |
|||
#if defined (USB_OTG_FS) || defined (USB_OTG_HS) |
|||
/**
|
|||
* @brief Set Tx FIFO |
|||
* @param hpcd PCD handle |
|||
* @param fifo The number of Tx fifo |
|||
* @param size Fifo size |
|||
* @retval HAL status |
|||
*/ |
|||
HAL_StatusTypeDef HAL_PCDEx_SetTxFiFo(PCD_HandleTypeDef *hpcd, uint8_t fifo, uint16_t size) |
|||
{ |
|||
uint8_t i; |
|||
uint32_t Tx_Offset; |
|||
|
|||
/* TXn min size = 16 words. (n : Transmit FIFO index)
|
|||
When a TxFIFO is not used, the Configuration should be as follows: |
|||
case 1 : n > m and Txn is not used (n,m : Transmit FIFO indexes) |
|||
--> Txm can use the space allocated for Txn. |
|||
case2 : n < m and Txn is not used (n,m : Transmit FIFO indexes) |
|||
--> Txn should be configured with the minimum space of 16 words |
|||
The FIFO is used optimally when used TxFIFOs are allocated in the top |
|||
of the FIFO.Ex: use EP1 and EP2 as IN instead of EP1 and EP3 as IN ones. |
|||
When DMA is used 3n * FIFO locations should be reserved for internal DMA registers */ |
|||
|
|||
Tx_Offset = hpcd->Instance->GRXFSIZ; |
|||
|
|||
if (fifo == 0U) |
|||
{ |
|||
hpcd->Instance->DIEPTXF0_HNPTXFSIZ = ((uint32_t)size << 16) | Tx_Offset; |
|||
} |
|||
else |
|||
{ |
|||
Tx_Offset += (hpcd->Instance->DIEPTXF0_HNPTXFSIZ) >> 16; |
|||
for (i = 0U; i < (fifo - 1U); i++) |
|||
{ |
|||
Tx_Offset += (hpcd->Instance->DIEPTXF[i] >> 16); |
|||
} |
|||
|
|||
/* Multiply Tx_Size by 2 to get higher performance */ |
|||
hpcd->Instance->DIEPTXF[fifo - 1U] = ((uint32_t)size << 16) | Tx_Offset; |
|||
} |
|||
|
|||
return HAL_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Set Rx FIFO |
|||
* @param hpcd PCD handle |
|||
* @param size Size of Rx fifo |
|||
* @retval HAL status |
|||
*/ |
|||
HAL_StatusTypeDef HAL_PCDEx_SetRxFiFo(PCD_HandleTypeDef *hpcd, uint16_t size) |
|||
{ |
|||
hpcd->Instance->GRXFSIZ = size; |
|||
|
|||
return HAL_OK; |
|||
} |
|||
#if defined(STM32F446xx) || defined(STM32F469xx) || defined(STM32F479xx) || defined(STM32F412Zx) || defined(STM32F412Vx) || defined(STM32F412Rx) || defined(STM32F412Cx) || defined(STM32F413xx) || defined(STM32F423xx) |
|||
/**
|
|||
* @brief Activate LPM feature. |
|||
* @param hpcd PCD handle |
|||
* @retval HAL status |
|||
*/ |
|||
HAL_StatusTypeDef HAL_PCDEx_ActivateLPM(PCD_HandleTypeDef *hpcd) |
|||
{ |
|||
USB_OTG_GlobalTypeDef *USBx = hpcd->Instance; |
|||
|
|||
hpcd->lpm_active = 1U; |
|||
hpcd->LPM_State = LPM_L0; |
|||
USBx->GINTMSK |= USB_OTG_GINTMSK_LPMINTM; |
|||
USBx->GLPMCFG |= (USB_OTG_GLPMCFG_LPMEN | USB_OTG_GLPMCFG_LPMACK | USB_OTG_GLPMCFG_ENBESL); |
|||
|
|||
return HAL_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Deactivate LPM feature. |
|||
* @param hpcd PCD handle |
|||
* @retval HAL status |
|||
*/ |
|||
HAL_StatusTypeDef HAL_PCDEx_DeActivateLPM(PCD_HandleTypeDef *hpcd) |
|||
{ |
|||
USB_OTG_GlobalTypeDef *USBx = hpcd->Instance; |
|||
|
|||
hpcd->lpm_active = 0U; |
|||
USBx->GINTMSK &= ~USB_OTG_GINTMSK_LPMINTM; |
|||
USBx->GLPMCFG &= ~(USB_OTG_GLPMCFG_LPMEN | USB_OTG_GLPMCFG_LPMACK | USB_OTG_GLPMCFG_ENBESL); |
|||
|
|||
return HAL_OK; |
|||
} |
|||
#endif /* defined(STM32F446xx) || defined(STM32F469xx) || defined(STM32F479xx) || defined(STM32F412Zx) || defined(STM32F412Vx) || defined(STM32F412Rx) || defined(STM32F412Cx) || defined(STM32F413xx) || defined(STM32F423xx) */ |
|||
#if defined(STM32F412Zx) || defined(STM32F412Vx) || defined(STM32F412Rx) || defined(STM32F412Cx) || defined(STM32F413xx) || defined(STM32F423xx) |
|||
/**
|
|||
* @brief Handle BatteryCharging Process. |
|||
* @param hpcd PCD handle |
|||
* @retval HAL status |
|||
*/ |
|||
void HAL_PCDEx_BCD_VBUSDetect(PCD_HandleTypeDef *hpcd) |
|||
{ |
|||
USB_OTG_GlobalTypeDef *USBx = hpcd->Instance; |
|||
uint32_t tickstart = HAL_GetTick(); |
|||
|
|||
/* Enable DCD : Data Contact Detect */ |
|||
USBx->GCCFG |= USB_OTG_GCCFG_DCDEN; |
|||
|
|||
/* Wait for Min DCD Timeout */ |
|||
HAL_Delay(300U); |
|||
|
|||
/* Check Detect flag */ |
|||
if ((USBx->GCCFG & USB_OTG_GCCFG_DCDET) == USB_OTG_GCCFG_DCDET) |
|||
{ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
hpcd->BCDCallback(hpcd, PCD_BCD_CONTACT_DETECTION); |
|||
#else |
|||
HAL_PCDEx_BCD_Callback(hpcd, PCD_BCD_CONTACT_DETECTION); |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
} |
|||
|
|||
/* Primary detection: checks if connected to Standard Downstream Port
|
|||
(without charging capability) */ |
|||
USBx->GCCFG &= ~ USB_OTG_GCCFG_DCDEN; |
|||
HAL_Delay(50U); |
|||
USBx->GCCFG |= USB_OTG_GCCFG_PDEN; |
|||
HAL_Delay(50U); |
|||
|
|||
if ((USBx->GCCFG & USB_OTG_GCCFG_PDET) == 0U) |
|||
{ |
|||
/* Case of Standard Downstream Port */ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
hpcd->BCDCallback(hpcd, PCD_BCD_STD_DOWNSTREAM_PORT); |
|||
#else |
|||
HAL_PCDEx_BCD_Callback(hpcd, PCD_BCD_STD_DOWNSTREAM_PORT); |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
} |
|||
else |
|||
{ |
|||
/* start secondary detection to check connection to Charging Downstream
|
|||
Port or Dedicated Charging Port */ |
|||
USBx->GCCFG &= ~ USB_OTG_GCCFG_PDEN; |
|||
HAL_Delay(50U); |
|||
USBx->GCCFG |= USB_OTG_GCCFG_SDEN; |
|||
HAL_Delay(50U); |
|||
|
|||
if ((USBx->GCCFG & USB_OTG_GCCFG_SDET) == USB_OTG_GCCFG_SDET) |
|||
{ |
|||
/* case Dedicated Charging Port */ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
hpcd->BCDCallback(hpcd, PCD_BCD_DEDICATED_CHARGING_PORT); |
|||
#else |
|||
HAL_PCDEx_BCD_Callback(hpcd, PCD_BCD_DEDICATED_CHARGING_PORT); |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
} |
|||
else |
|||
{ |
|||
/* case Charging Downstream Port */ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
hpcd->BCDCallback(hpcd, PCD_BCD_CHARGING_DOWNSTREAM_PORT); |
|||
#else |
|||
HAL_PCDEx_BCD_Callback(hpcd, PCD_BCD_CHARGING_DOWNSTREAM_PORT); |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
} |
|||
} |
|||
|
|||
/* Battery Charging capability discovery finished */ |
|||
(void)HAL_PCDEx_DeActivateBCD(hpcd); |
|||
|
|||
/* Check for the Timeout, else start USB Device */ |
|||
if ((HAL_GetTick() - tickstart) > 1000U) |
|||
{ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
hpcd->BCDCallback(hpcd, PCD_BCD_ERROR); |
|||
#else |
|||
HAL_PCDEx_BCD_Callback(hpcd, PCD_BCD_ERROR); |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
} |
|||
else |
|||
{ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
hpcd->BCDCallback(hpcd, PCD_BCD_DISCOVERY_COMPLETED); |
|||
#else |
|||
HAL_PCDEx_BCD_Callback(hpcd, PCD_BCD_DISCOVERY_COMPLETED); |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
} |
|||
} |
|||
|
|||
/**
|
|||
* @brief Activate BatteryCharging feature. |
|||
* @param hpcd PCD handle |
|||
* @retval HAL status |
|||
*/ |
|||
HAL_StatusTypeDef HAL_PCDEx_ActivateBCD(PCD_HandleTypeDef *hpcd) |
|||
{ |
|||
USB_OTG_GlobalTypeDef *USBx = hpcd->Instance; |
|||
|
|||
USBx->GCCFG &= ~(USB_OTG_GCCFG_PDEN); |
|||
USBx->GCCFG &= ~(USB_OTG_GCCFG_SDEN); |
|||
|
|||
/* Power Down USB transceiver */ |
|||
USBx->GCCFG &= ~(USB_OTG_GCCFG_PWRDWN); |
|||
|
|||
/* Enable Battery charging */ |
|||
USBx->GCCFG |= USB_OTG_GCCFG_BCDEN; |
|||
|
|||
hpcd->battery_charging_active = 1U; |
|||
|
|||
return HAL_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Deactivate BatteryCharging feature. |
|||
* @param hpcd PCD handle |
|||
* @retval HAL status |
|||
*/ |
|||
HAL_StatusTypeDef HAL_PCDEx_DeActivateBCD(PCD_HandleTypeDef *hpcd) |
|||
{ |
|||
USB_OTG_GlobalTypeDef *USBx = hpcd->Instance; |
|||
|
|||
USBx->GCCFG &= ~(USB_OTG_GCCFG_SDEN); |
|||
USBx->GCCFG &= ~(USB_OTG_GCCFG_PDEN); |
|||
|
|||
/* Disable Battery charging */ |
|||
USBx->GCCFG &= ~(USB_OTG_GCCFG_BCDEN); |
|||
|
|||
hpcd->battery_charging_active = 0U; |
|||
|
|||
return HAL_OK; |
|||
} |
|||
#endif /* defined(STM32F412Zx) || defined(STM32F412Vx) || defined(STM32F412Rx) || defined(STM32F412Cx) || defined(STM32F413xx) || defined(STM32F423xx) */ |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
|
|||
/**
|
|||
* @brief Send LPM message to user layer callback. |
|||
* @param hpcd PCD handle |
|||
* @param msg LPM message |
|||
* @retval HAL status |
|||
*/ |
|||
__weak void HAL_PCDEx_LPM_Callback(PCD_HandleTypeDef *hpcd, PCD_LPM_MsgTypeDef msg) |
|||
{ |
|||
/* Prevent unused argument(s) compilation warning */ |
|||
UNUSED(hpcd); |
|||
UNUSED(msg); |
|||
|
|||
/* NOTE : This function should not be modified, when the callback is needed,
|
|||
the HAL_PCDEx_LPM_Callback could be implemented in the user file |
|||
*/ |
|||
} |
|||
|
|||
/**
|
|||
* @brief Send BatteryCharging message to user layer callback. |
|||
* @param hpcd PCD handle |
|||
* @param msg LPM message |
|||
* @retval HAL status |
|||
*/ |
|||
__weak void HAL_PCDEx_BCD_Callback(PCD_HandleTypeDef *hpcd, PCD_BCD_MsgTypeDef msg) |
|||
{ |
|||
/* Prevent unused argument(s) compilation warning */ |
|||
UNUSED(hpcd); |
|||
UNUSED(msg); |
|||
|
|||
/* NOTE : This function should not be modified, when the callback is needed,
|
|||
the HAL_PCDEx_BCD_Callback could be implemented in the user file |
|||
*/ |
|||
} |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
#endif /* defined (USB_OTG_FS) || defined (USB_OTG_HS) */ |
|||
#endif /* HAL_PCD_MODULE_ENABLED */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
File diff suppressed because it is too large
@ -0,0 +1,179 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file usbd_cdc.h |
|||
* @author MCD Application Team |
|||
* @brief header file for the usbd_cdc.c file. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2015 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __USB_CDC_H |
|||
#define __USB_CDC_H |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_ioreq.h" |
|||
|
|||
/** @addtogroup STM32_USB_DEVICE_LIBRARY
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup usbd_cdc
|
|||
* @brief This file is the Header file for usbd_cdc.c |
|||
* @{ |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup usbd_cdc_Exported_Defines
|
|||
* @{ |
|||
*/ |
|||
#ifndef CDC_IN_EP |
|||
#define CDC_IN_EP 0x81U /* EP1 for data IN */ |
|||
#endif /* CDC_IN_EP */ |
|||
#ifndef CDC_OUT_EP |
|||
#define CDC_OUT_EP 0x01U /* EP1 for data OUT */ |
|||
#endif /* CDC_OUT_EP */ |
|||
#ifndef CDC_CMD_EP |
|||
#define CDC_CMD_EP 0x82U /* EP2 for CDC commands */ |
|||
#endif /* CDC_CMD_EP */ |
|||
|
|||
#ifndef CDC_HS_BINTERVAL |
|||
#define CDC_HS_BINTERVAL 0x10U |
|||
#endif /* CDC_HS_BINTERVAL */ |
|||
|
|||
#ifndef CDC_FS_BINTERVAL |
|||
#define CDC_FS_BINTERVAL 0x10U |
|||
#endif /* CDC_FS_BINTERVAL */ |
|||
|
|||
/* CDC Endpoints parameters: you can fine tune these values depending on the needed baudrates and performance. */ |
|||
#define CDC_DATA_HS_MAX_PACKET_SIZE 512U /* Endpoint IN & OUT Packet size */ |
|||
#define CDC_DATA_FS_MAX_PACKET_SIZE 64U /* Endpoint IN & OUT Packet size */ |
|||
#define CDC_CMD_PACKET_SIZE 8U /* Control Endpoint Packet size */ |
|||
|
|||
#define USB_CDC_CONFIG_DESC_SIZ 67U |
|||
#define CDC_DATA_HS_IN_PACKET_SIZE CDC_DATA_HS_MAX_PACKET_SIZE |
|||
#define CDC_DATA_HS_OUT_PACKET_SIZE CDC_DATA_HS_MAX_PACKET_SIZE |
|||
|
|||
#define CDC_DATA_FS_IN_PACKET_SIZE CDC_DATA_FS_MAX_PACKET_SIZE |
|||
#define CDC_DATA_FS_OUT_PACKET_SIZE CDC_DATA_FS_MAX_PACKET_SIZE |
|||
|
|||
#define CDC_REQ_MAX_DATA_SIZE 0x7U |
|||
/*---------------------------------------------------------------------*/ |
|||
/* CDC definitions */ |
|||
/*---------------------------------------------------------------------*/ |
|||
#define CDC_SEND_ENCAPSULATED_COMMAND 0x00U |
|||
#define CDC_GET_ENCAPSULATED_RESPONSE 0x01U |
|||
#define CDC_SET_COMM_FEATURE 0x02U |
|||
#define CDC_GET_COMM_FEATURE 0x03U |
|||
#define CDC_CLEAR_COMM_FEATURE 0x04U |
|||
#define CDC_SET_LINE_CODING 0x20U |
|||
#define CDC_GET_LINE_CODING 0x21U |
|||
#define CDC_SET_CONTROL_LINE_STATE 0x22U |
|||
#define CDC_SEND_BREAK 0x23U |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_CORE_Exported_TypesDefinitions
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
typedef struct |
|||
{ |
|||
uint32_t bitrate; |
|||
uint8_t format; |
|||
uint8_t paritytype; |
|||
uint8_t datatype; |
|||
} USBD_CDC_LineCodingTypeDef; |
|||
|
|||
typedef struct _USBD_CDC_Itf |
|||
{ |
|||
int8_t (* Init)(void); |
|||
int8_t (* DeInit)(void); |
|||
int8_t (* Control)(uint8_t cmd, uint8_t *pbuf, uint16_t length); |
|||
int8_t (* Receive)(uint8_t *Buf, uint32_t *Len); |
|||
int8_t (* TransmitCplt)(uint8_t *Buf, uint32_t *Len, uint8_t epnum); |
|||
} USBD_CDC_ItfTypeDef; |
|||
|
|||
|
|||
typedef struct |
|||
{ |
|||
uint32_t data[CDC_DATA_HS_MAX_PACKET_SIZE / 4U]; /* Force 32-bit alignment */ |
|||
uint8_t CmdOpCode; |
|||
uint8_t CmdLength; |
|||
uint8_t *RxBuffer; |
|||
uint8_t *TxBuffer; |
|||
uint32_t RxLength; |
|||
uint32_t TxLength; |
|||
|
|||
__IO uint32_t TxState; |
|||
__IO uint32_t RxState; |
|||
} USBD_CDC_HandleTypeDef; |
|||
|
|||
|
|||
|
|||
/** @defgroup USBD_CORE_Exported_Macros
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CORE_Exported_Variables
|
|||
* @{ |
|||
*/ |
|||
|
|||
extern USBD_ClassTypeDef USBD_CDC; |
|||
#define USBD_CDC_CLASS &USBD_CDC |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USB_CORE_Exported_Functions
|
|||
* @{ |
|||
*/ |
|||
uint8_t USBD_CDC_RegisterInterface(USBD_HandleTypeDef *pdev, |
|||
USBD_CDC_ItfTypeDef *fops); |
|||
|
|||
uint8_t USBD_CDC_SetTxBuffer(USBD_HandleTypeDef *pdev, uint8_t *pbuff, |
|||
uint32_t length); |
|||
|
|||
uint8_t USBD_CDC_SetRxBuffer(USBD_HandleTypeDef *pdev, uint8_t *pbuff); |
|||
uint8_t USBD_CDC_ReceivePacket(USBD_HandleTypeDef *pdev); |
|||
uint8_t USBD_CDC_TransmitPacket(USBD_HandleTypeDef *pdev); |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* __USB_CDC_H */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
@ -0,0 +1,875 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file usbd_cdc.c |
|||
* @author MCD Application Team |
|||
* @brief This file provides the high layer firmware functions to manage the |
|||
* following functionalities of the USB CDC Class: |
|||
* - Initialization and Configuration of high and low layer |
|||
* - Enumeration as CDC Device (and enumeration for each implemented memory interface) |
|||
* - OUT/IN data transfer |
|||
* - Command IN transfer (class requests management) |
|||
* - Error management |
|||
* |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2015 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
* @verbatim |
|||
* |
|||
* =================================================================== |
|||
* CDC Class Driver Description |
|||
* =================================================================== |
|||
* This driver manages the "Universal Serial Bus Class Definitions for Communications Devices |
|||
* Revision 1.2 November 16, 2007" and the sub-protocol specification of "Universal Serial Bus |
|||
* Communications Class Subclass Specification for PSTN Devices Revision 1.2 February 9, 2007" |
|||
* This driver implements the following aspects of the specification: |
|||
* - Device descriptor management |
|||
* - Configuration descriptor management |
|||
* - Enumeration as CDC device with 2 data endpoints (IN and OUT) and 1 command endpoint (IN) |
|||
* - Requests management (as described in section 6.2 in specification) |
|||
* - Abstract Control Model compliant |
|||
* - Union Functional collection (using 1 IN endpoint for control) |
|||
* - Data interface class |
|||
* |
|||
* These aspects may be enriched or modified for a specific user application. |
|||
* |
|||
* This driver doesn't implement the following aspects of the specification |
|||
* (but it is possible to manage these features with some modifications on this driver): |
|||
* - Any class-specific aspect relative to communication classes should be managed by user application. |
|||
* - All communication classes other than PSTN are not managed |
|||
* |
|||
* @endverbatim |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* BSPDependencies
|
|||
- "stm32xxxxx_{eval}{discovery}{nucleo_144}.c" |
|||
- "stm32xxxxx_{eval}{discovery}_io.c" |
|||
EndBSPDependencies */ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_cdc.h" |
|||
#include "usbd_ctlreq.h" |
|||
|
|||
|
|||
/** @addtogroup STM32_USB_DEVICE_LIBRARY
|
|||
* @{ |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_CDC
|
|||
* @brief usbd core module |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_Private_TypesDefinitions
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_CDC_Private_Defines
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_CDC_Private_Macros
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_CDC_Private_FunctionPrototypes
|
|||
* @{ |
|||
*/ |
|||
|
|||
static uint8_t USBD_CDC_Init(USBD_HandleTypeDef *pdev, uint8_t cfgidx); |
|||
static uint8_t USBD_CDC_DeInit(USBD_HandleTypeDef *pdev, uint8_t cfgidx); |
|||
static uint8_t USBD_CDC_Setup(USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req); |
|||
static uint8_t USBD_CDC_DataIn(USBD_HandleTypeDef *pdev, uint8_t epnum); |
|||
static uint8_t USBD_CDC_DataOut(USBD_HandleTypeDef *pdev, uint8_t epnum); |
|||
static uint8_t USBD_CDC_EP0_RxReady(USBD_HandleTypeDef *pdev); |
|||
#ifndef USE_USBD_COMPOSITE |
|||
static uint8_t *USBD_CDC_GetFSCfgDesc(uint16_t *length); |
|||
static uint8_t *USBD_CDC_GetHSCfgDesc(uint16_t *length); |
|||
static uint8_t *USBD_CDC_GetOtherSpeedCfgDesc(uint16_t *length); |
|||
static uint8_t *USBD_CDC_GetOtherSpeedCfgDesc(uint16_t *length); |
|||
uint8_t *USBD_CDC_GetDeviceQualifierDescriptor(uint16_t *length); |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
|
|||
#ifndef USE_USBD_COMPOSITE |
|||
/* USB Standard Device Descriptor */ |
|||
__ALIGN_BEGIN static uint8_t USBD_CDC_DeviceQualifierDesc[USB_LEN_DEV_QUALIFIER_DESC] __ALIGN_END = |
|||
{ |
|||
USB_LEN_DEV_QUALIFIER_DESC, |
|||
USB_DESC_TYPE_DEVICE_QUALIFIER, |
|||
0x00, |
|||
0x02, |
|||
0x00, |
|||
0x00, |
|||
0x00, |
|||
0x40, |
|||
0x01, |
|||
0x00, |
|||
}; |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_Private_Variables
|
|||
* @{ |
|||
*/ |
|||
|
|||
|
|||
/* CDC interface class callbacks structure */ |
|||
USBD_ClassTypeDef USBD_CDC = |
|||
{ |
|||
USBD_CDC_Init, |
|||
USBD_CDC_DeInit, |
|||
USBD_CDC_Setup, |
|||
NULL, /* EP0_TxSent */ |
|||
USBD_CDC_EP0_RxReady, |
|||
USBD_CDC_DataIn, |
|||
USBD_CDC_DataOut, |
|||
NULL, |
|||
NULL, |
|||
NULL, |
|||
#ifdef USE_USBD_COMPOSITE |
|||
NULL, |
|||
NULL, |
|||
NULL, |
|||
NULL, |
|||
#else |
|||
USBD_CDC_GetHSCfgDesc, |
|||
USBD_CDC_GetFSCfgDesc, |
|||
USBD_CDC_GetOtherSpeedCfgDesc, |
|||
USBD_CDC_GetDeviceQualifierDescriptor, |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
}; |
|||
|
|||
#ifndef USE_USBD_COMPOSITE |
|||
/* USB CDC device Configuration Descriptor */ |
|||
__ALIGN_BEGIN static uint8_t USBD_CDC_CfgDesc[USB_CDC_CONFIG_DESC_SIZ] __ALIGN_END = |
|||
{ |
|||
/* Configuration Descriptor */ |
|||
0x09, /* bLength: Configuration Descriptor size */ |
|||
USB_DESC_TYPE_CONFIGURATION, /* bDescriptorType: Configuration */ |
|||
USB_CDC_CONFIG_DESC_SIZ, /* wTotalLength */ |
|||
0x00, |
|||
0x02, /* bNumInterfaces: 2 interfaces */ |
|||
0x01, /* bConfigurationValue: Configuration value */ |
|||
0x00, /* iConfiguration: Index of string descriptor
|
|||
describing the configuration */ |
|||
#if (USBD_SELF_POWERED == 1U) |
|||
0xC0, /* bmAttributes: Bus Powered according to user configuration */ |
|||
#else |
|||
0x80, /* bmAttributes: Bus Powered according to user configuration */ |
|||
#endif /* USBD_SELF_POWERED */ |
|||
USBD_MAX_POWER, /* MaxPower (mA) */ |
|||
|
|||
/*---------------------------------------------------------------------------*/ |
|||
|
|||
/* Interface Descriptor */ |
|||
0x09, /* bLength: Interface Descriptor size */ |
|||
USB_DESC_TYPE_INTERFACE, /* bDescriptorType: Interface */ |
|||
/* Interface descriptor type */ |
|||
0x00, /* bInterfaceNumber: Number of Interface */ |
|||
0x00, /* bAlternateSetting: Alternate setting */ |
|||
0x01, /* bNumEndpoints: One endpoint used */ |
|||
0x02, /* bInterfaceClass: Communication Interface Class */ |
|||
0x02, /* bInterfaceSubClass: Abstract Control Model */ |
|||
0x01, /* bInterfaceProtocol: Common AT commands */ |
|||
0x00, /* iInterface */ |
|||
|
|||
/* Header Functional Descriptor */ |
|||
0x05, /* bLength: Endpoint Descriptor size */ |
|||
0x24, /* bDescriptorType: CS_INTERFACE */ |
|||
0x00, /* bDescriptorSubtype: Header Func Desc */ |
|||
0x10, /* bcdCDC: spec release number */ |
|||
0x01, |
|||
|
|||
/* Call Management Functional Descriptor */ |
|||
0x05, /* bFunctionLength */ |
|||
0x24, /* bDescriptorType: CS_INTERFACE */ |
|||
0x01, /* bDescriptorSubtype: Call Management Func Desc */ |
|||
0x00, /* bmCapabilities: D0+D1 */ |
|||
0x01, /* bDataInterface */ |
|||
|
|||
/* ACM Functional Descriptor */ |
|||
0x04, /* bFunctionLength */ |
|||
0x24, /* bDescriptorType: CS_INTERFACE */ |
|||
0x02, /* bDescriptorSubtype: Abstract Control Management desc */ |
|||
0x02, /* bmCapabilities */ |
|||
|
|||
/* Union Functional Descriptor */ |
|||
0x05, /* bFunctionLength */ |
|||
0x24, /* bDescriptorType: CS_INTERFACE */ |
|||
0x06, /* bDescriptorSubtype: Union func desc */ |
|||
0x00, /* bMasterInterface: Communication class interface */ |
|||
0x01, /* bSlaveInterface0: Data Class Interface */ |
|||
|
|||
/* Endpoint 2 Descriptor */ |
|||
0x07, /* bLength: Endpoint Descriptor size */ |
|||
USB_DESC_TYPE_ENDPOINT, /* bDescriptorType: Endpoint */ |
|||
CDC_CMD_EP, /* bEndpointAddress */ |
|||
0x03, /* bmAttributes: Interrupt */ |
|||
LOBYTE(CDC_CMD_PACKET_SIZE), /* wMaxPacketSize */ |
|||
HIBYTE(CDC_CMD_PACKET_SIZE), |
|||
CDC_FS_BINTERVAL, /* bInterval */ |
|||
/*---------------------------------------------------------------------------*/ |
|||
|
|||
/* Data class interface descriptor */ |
|||
0x09, /* bLength: Endpoint Descriptor size */ |
|||
USB_DESC_TYPE_INTERFACE, /* bDescriptorType: */ |
|||
0x01, /* bInterfaceNumber: Number of Interface */ |
|||
0x00, /* bAlternateSetting: Alternate setting */ |
|||
0x02, /* bNumEndpoints: Two endpoints used */ |
|||
0x0A, /* bInterfaceClass: CDC */ |
|||
0x00, /* bInterfaceSubClass */ |
|||
0x00, /* bInterfaceProtocol */ |
|||
0x00, /* iInterface */ |
|||
|
|||
/* Endpoint OUT Descriptor */ |
|||
0x07, /* bLength: Endpoint Descriptor size */ |
|||
USB_DESC_TYPE_ENDPOINT, /* bDescriptorType: Endpoint */ |
|||
CDC_OUT_EP, /* bEndpointAddress */ |
|||
0x02, /* bmAttributes: Bulk */ |
|||
LOBYTE(CDC_DATA_FS_MAX_PACKET_SIZE), /* wMaxPacketSize */ |
|||
HIBYTE(CDC_DATA_FS_MAX_PACKET_SIZE), |
|||
0x00, /* bInterval */ |
|||
|
|||
/* Endpoint IN Descriptor */ |
|||
0x07, /* bLength: Endpoint Descriptor size */ |
|||
USB_DESC_TYPE_ENDPOINT, /* bDescriptorType: Endpoint */ |
|||
CDC_IN_EP, /* bEndpointAddress */ |
|||
0x02, /* bmAttributes: Bulk */ |
|||
LOBYTE(CDC_DATA_FS_MAX_PACKET_SIZE), /* wMaxPacketSize */ |
|||
HIBYTE(CDC_DATA_FS_MAX_PACKET_SIZE), |
|||
0x00 /* bInterval */ |
|||
}; |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
|
|||
static uint8_t CDCInEpAdd = CDC_IN_EP; |
|||
static uint8_t CDCOutEpAdd = CDC_OUT_EP; |
|||
static uint8_t CDCCmdEpAdd = CDC_CMD_EP; |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_Private_Functions
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_Init |
|||
* Initialize the CDC interface |
|||
* @param pdev: device instance |
|||
* @param cfgidx: Configuration index |
|||
* @retval status |
|||
*/ |
|||
static uint8_t USBD_CDC_Init(USBD_HandleTypeDef *pdev, uint8_t cfgidx) |
|||
{ |
|||
UNUSED(cfgidx); |
|||
USBD_CDC_HandleTypeDef *hcdc; |
|||
|
|||
hcdc = (USBD_CDC_HandleTypeDef *)USBD_malloc(sizeof(USBD_CDC_HandleTypeDef)); |
|||
|
|||
if (hcdc == NULL) |
|||
{ |
|||
pdev->pClassDataCmsit[pdev->classId] = NULL; |
|||
return (uint8_t)USBD_EMEM; |
|||
} |
|||
|
|||
(void)USBD_memset(hcdc, 0, sizeof(USBD_CDC_HandleTypeDef)); |
|||
|
|||
pdev->pClassDataCmsit[pdev->classId] = (void *)hcdc; |
|||
pdev->pClassData = pdev->pClassDataCmsit[pdev->classId]; |
|||
|
|||
#ifdef USE_USBD_COMPOSITE |
|||
/* Get the Endpoints addresses allocated for this class instance */ |
|||
CDCInEpAdd = USBD_CoreGetEPAdd(pdev, USBD_EP_IN, USBD_EP_TYPE_BULK); |
|||
CDCOutEpAdd = USBD_CoreGetEPAdd(pdev, USBD_EP_OUT, USBD_EP_TYPE_BULK); |
|||
CDCCmdEpAdd = USBD_CoreGetEPAdd(pdev, USBD_EP_IN, USBD_EP_TYPE_INTR); |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
|
|||
if (pdev->dev_speed == USBD_SPEED_HIGH) |
|||
{ |
|||
/* Open EP IN */ |
|||
(void)USBD_LL_OpenEP(pdev, CDCInEpAdd, USBD_EP_TYPE_BULK, |
|||
CDC_DATA_HS_IN_PACKET_SIZE); |
|||
|
|||
pdev->ep_in[CDCInEpAdd & 0xFU].is_used = 1U; |
|||
|
|||
/* Open EP OUT */ |
|||
(void)USBD_LL_OpenEP(pdev, CDCOutEpAdd, USBD_EP_TYPE_BULK, |
|||
CDC_DATA_HS_OUT_PACKET_SIZE); |
|||
|
|||
pdev->ep_out[CDCOutEpAdd & 0xFU].is_used = 1U; |
|||
|
|||
/* Set bInterval for CDC CMD Endpoint */ |
|||
pdev->ep_in[CDCCmdEpAdd & 0xFU].bInterval = CDC_HS_BINTERVAL; |
|||
} |
|||
else |
|||
{ |
|||
/* Open EP IN */ |
|||
(void)USBD_LL_OpenEP(pdev, CDCInEpAdd, USBD_EP_TYPE_BULK, |
|||
CDC_DATA_FS_IN_PACKET_SIZE); |
|||
|
|||
pdev->ep_in[CDCInEpAdd & 0xFU].is_used = 1U; |
|||
|
|||
/* Open EP OUT */ |
|||
(void)USBD_LL_OpenEP(pdev, CDCOutEpAdd, USBD_EP_TYPE_BULK, |
|||
CDC_DATA_FS_OUT_PACKET_SIZE); |
|||
|
|||
pdev->ep_out[CDCOutEpAdd & 0xFU].is_used = 1U; |
|||
|
|||
/* Set bInterval for CMD Endpoint */ |
|||
pdev->ep_in[CDCCmdEpAdd & 0xFU].bInterval = CDC_FS_BINTERVAL; |
|||
} |
|||
|
|||
/* Open Command IN EP */ |
|||
(void)USBD_LL_OpenEP(pdev, CDCCmdEpAdd, USBD_EP_TYPE_INTR, CDC_CMD_PACKET_SIZE); |
|||
pdev->ep_in[CDCCmdEpAdd & 0xFU].is_used = 1U; |
|||
|
|||
hcdc->RxBuffer = NULL; |
|||
|
|||
/* Init physical Interface components */ |
|||
((USBD_CDC_ItfTypeDef *)pdev->pUserData[pdev->classId])->Init(); |
|||
|
|||
/* Init Xfer states */ |
|||
hcdc->TxState = 0U; |
|||
hcdc->RxState = 0U; |
|||
|
|||
if (hcdc->RxBuffer == NULL) |
|||
{ |
|||
return (uint8_t)USBD_EMEM; |
|||
} |
|||
|
|||
if (pdev->dev_speed == USBD_SPEED_HIGH) |
|||
{ |
|||
/* Prepare Out endpoint to receive next packet */ |
|||
(void)USBD_LL_PrepareReceive(pdev, CDCOutEpAdd, hcdc->RxBuffer, |
|||
CDC_DATA_HS_OUT_PACKET_SIZE); |
|||
} |
|||
else |
|||
{ |
|||
/* Prepare Out endpoint to receive next packet */ |
|||
(void)USBD_LL_PrepareReceive(pdev, CDCOutEpAdd, hcdc->RxBuffer, |
|||
CDC_DATA_FS_OUT_PACKET_SIZE); |
|||
} |
|||
|
|||
return (uint8_t)USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_Init |
|||
* DeInitialize the CDC layer |
|||
* @param pdev: device instance |
|||
* @param cfgidx: Configuration index |
|||
* @retval status |
|||
*/ |
|||
static uint8_t USBD_CDC_DeInit(USBD_HandleTypeDef *pdev, uint8_t cfgidx) |
|||
{ |
|||
UNUSED(cfgidx); |
|||
|
|||
|
|||
#ifdef USE_USBD_COMPOSITE |
|||
/* Get the Endpoints addresses allocated for this CDC class instance */ |
|||
CDCInEpAdd = USBD_CoreGetEPAdd(pdev, USBD_EP_IN, USBD_EP_TYPE_BULK); |
|||
CDCOutEpAdd = USBD_CoreGetEPAdd(pdev, USBD_EP_OUT, USBD_EP_TYPE_BULK); |
|||
CDCCmdEpAdd = USBD_CoreGetEPAdd(pdev, USBD_EP_IN, USBD_EP_TYPE_INTR); |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
|
|||
/* Close EP IN */ |
|||
(void)USBD_LL_CloseEP(pdev, CDCInEpAdd); |
|||
pdev->ep_in[CDCInEpAdd & 0xFU].is_used = 0U; |
|||
|
|||
/* Close EP OUT */ |
|||
(void)USBD_LL_CloseEP(pdev, CDCOutEpAdd); |
|||
pdev->ep_out[CDCOutEpAdd & 0xFU].is_used = 0U; |
|||
|
|||
/* Close Command IN EP */ |
|||
(void)USBD_LL_CloseEP(pdev, CDCCmdEpAdd); |
|||
pdev->ep_in[CDCCmdEpAdd & 0xFU].is_used = 0U; |
|||
pdev->ep_in[CDCCmdEpAdd & 0xFU].bInterval = 0U; |
|||
|
|||
/* DeInit physical Interface components */ |
|||
if (pdev->pClassDataCmsit[pdev->classId] != NULL) |
|||
{ |
|||
((USBD_CDC_ItfTypeDef *)pdev->pUserData[pdev->classId])->DeInit(); |
|||
(void)USBD_free(pdev->pClassDataCmsit[pdev->classId]); |
|||
pdev->pClassDataCmsit[pdev->classId] = NULL; |
|||
pdev->pClassData = NULL; |
|||
} |
|||
|
|||
return (uint8_t)USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_Setup |
|||
* Handle the CDC specific requests |
|||
* @param pdev: instance |
|||
* @param req: usb requests |
|||
* @retval status |
|||
*/ |
|||
static uint8_t USBD_CDC_Setup(USBD_HandleTypeDef *pdev, |
|||
USBD_SetupReqTypedef *req) |
|||
{ |
|||
USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef *)pdev->pClassDataCmsit[pdev->classId]; |
|||
uint16_t len; |
|||
uint8_t ifalt = 0U; |
|||
uint16_t status_info = 0U; |
|||
USBD_StatusTypeDef ret = USBD_OK; |
|||
|
|||
if (hcdc == NULL) |
|||
{ |
|||
return (uint8_t)USBD_FAIL; |
|||
} |
|||
|
|||
switch (req->bmRequest & USB_REQ_TYPE_MASK) |
|||
{ |
|||
case USB_REQ_TYPE_CLASS: |
|||
if (req->wLength != 0U) |
|||
{ |
|||
if ((req->bmRequest & 0x80U) != 0U) |
|||
{ |
|||
((USBD_CDC_ItfTypeDef *)pdev->pUserData[pdev->classId])->Control(req->bRequest, |
|||
(uint8_t *)hcdc->data, |
|||
req->wLength); |
|||
|
|||
len = MIN(CDC_REQ_MAX_DATA_SIZE, req->wLength); |
|||
(void)USBD_CtlSendData(pdev, (uint8_t *)hcdc->data, len); |
|||
} |
|||
else |
|||
{ |
|||
hcdc->CmdOpCode = req->bRequest; |
|||
hcdc->CmdLength = (uint8_t)MIN(req->wLength, USB_MAX_EP0_SIZE); |
|||
|
|||
(void)USBD_CtlPrepareRx(pdev, (uint8_t *)hcdc->data, hcdc->CmdLength); |
|||
} |
|||
} |
|||
else |
|||
{ |
|||
((USBD_CDC_ItfTypeDef *)pdev->pUserData[pdev->classId])->Control(req->bRequest, |
|||
(uint8_t *)req, 0U); |
|||
} |
|||
break; |
|||
|
|||
case USB_REQ_TYPE_STANDARD: |
|||
switch (req->bRequest) |
|||
{ |
|||
case USB_REQ_GET_STATUS: |
|||
if (pdev->dev_state == USBD_STATE_CONFIGURED) |
|||
{ |
|||
(void)USBD_CtlSendData(pdev, (uint8_t *)&status_info, 2U); |
|||
} |
|||
else |
|||
{ |
|||
USBD_CtlError(pdev, req); |
|||
ret = USBD_FAIL; |
|||
} |
|||
break; |
|||
|
|||
case USB_REQ_GET_INTERFACE: |
|||
if (pdev->dev_state == USBD_STATE_CONFIGURED) |
|||
{ |
|||
(void)USBD_CtlSendData(pdev, &ifalt, 1U); |
|||
} |
|||
else |
|||
{ |
|||
USBD_CtlError(pdev, req); |
|||
ret = USBD_FAIL; |
|||
} |
|||
break; |
|||
|
|||
case USB_REQ_SET_INTERFACE: |
|||
if (pdev->dev_state != USBD_STATE_CONFIGURED) |
|||
{ |
|||
USBD_CtlError(pdev, req); |
|||
ret = USBD_FAIL; |
|||
} |
|||
break; |
|||
|
|||
case USB_REQ_CLEAR_FEATURE: |
|||
break; |
|||
|
|||
default: |
|||
USBD_CtlError(pdev, req); |
|||
ret = USBD_FAIL; |
|||
break; |
|||
} |
|||
break; |
|||
|
|||
default: |
|||
USBD_CtlError(pdev, req); |
|||
ret = USBD_FAIL; |
|||
break; |
|||
} |
|||
|
|||
return (uint8_t)ret; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_DataIn |
|||
* Data sent on non-control IN endpoint |
|||
* @param pdev: device instance |
|||
* @param epnum: endpoint number |
|||
* @retval status |
|||
*/ |
|||
static uint8_t USBD_CDC_DataIn(USBD_HandleTypeDef *pdev, uint8_t epnum) |
|||
{ |
|||
USBD_CDC_HandleTypeDef *hcdc; |
|||
PCD_HandleTypeDef *hpcd = (PCD_HandleTypeDef *)pdev->pData; |
|||
|
|||
if (pdev->pClassDataCmsit[pdev->classId] == NULL) |
|||
{ |
|||
return (uint8_t)USBD_FAIL; |
|||
} |
|||
|
|||
hcdc = (USBD_CDC_HandleTypeDef *)pdev->pClassDataCmsit[pdev->classId]; |
|||
|
|||
if ((pdev->ep_in[epnum & 0xFU].total_length > 0U) && |
|||
((pdev->ep_in[epnum & 0xFU].total_length % hpcd->IN_ep[epnum & 0xFU].maxpacket) == 0U)) |
|||
{ |
|||
/* Update the packet total length */ |
|||
pdev->ep_in[epnum & 0xFU].total_length = 0U; |
|||
|
|||
/* Send ZLP */ |
|||
(void)USBD_LL_Transmit(pdev, epnum, NULL, 0U); |
|||
} |
|||
else |
|||
{ |
|||
hcdc->TxState = 0U; |
|||
|
|||
if (((USBD_CDC_ItfTypeDef *)pdev->pUserData[pdev->classId])->TransmitCplt != NULL) |
|||
{ |
|||
((USBD_CDC_ItfTypeDef *)pdev->pUserData[pdev->classId])->TransmitCplt(hcdc->TxBuffer, &hcdc->TxLength, epnum); |
|||
} |
|||
} |
|||
|
|||
return (uint8_t)USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_DataOut |
|||
* Data received on non-control Out endpoint |
|||
* @param pdev: device instance |
|||
* @param epnum: endpoint number |
|||
* @retval status |
|||
*/ |
|||
static uint8_t USBD_CDC_DataOut(USBD_HandleTypeDef *pdev, uint8_t epnum) |
|||
{ |
|||
USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef *)pdev->pClassDataCmsit[pdev->classId]; |
|||
|
|||
if (pdev->pClassDataCmsit[pdev->classId] == NULL) |
|||
{ |
|||
return (uint8_t)USBD_FAIL; |
|||
} |
|||
|
|||
/* Get the received data length */ |
|||
hcdc->RxLength = USBD_LL_GetRxDataSize(pdev, epnum); |
|||
|
|||
/* USB data will be immediately processed, this allow next USB traffic being
|
|||
NAKed till the end of the application Xfer */ |
|||
|
|||
((USBD_CDC_ItfTypeDef *)pdev->pUserData[pdev->classId])->Receive(hcdc->RxBuffer, &hcdc->RxLength); |
|||
|
|||
return (uint8_t)USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_EP0_RxReady |
|||
* Handle EP0 Rx Ready event |
|||
* @param pdev: device instance |
|||
* @retval status |
|||
*/ |
|||
static uint8_t USBD_CDC_EP0_RxReady(USBD_HandleTypeDef *pdev) |
|||
{ |
|||
USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef *)pdev->pClassDataCmsit[pdev->classId]; |
|||
|
|||
if (hcdc == NULL) |
|||
{ |
|||
return (uint8_t)USBD_FAIL; |
|||
} |
|||
|
|||
if ((pdev->pUserData[pdev->classId] != NULL) && (hcdc->CmdOpCode != 0xFFU)) |
|||
{ |
|||
((USBD_CDC_ItfTypeDef *)pdev->pUserData[pdev->classId])->Control(hcdc->CmdOpCode, |
|||
(uint8_t *)hcdc->data, |
|||
(uint16_t)hcdc->CmdLength); |
|||
hcdc->CmdOpCode = 0xFFU; |
|||
} |
|||
|
|||
return (uint8_t)USBD_OK; |
|||
} |
|||
#ifndef USE_USBD_COMPOSITE |
|||
/**
|
|||
* @brief USBD_CDC_GetFSCfgDesc |
|||
* Return configuration descriptor |
|||
* @param length : pointer data length |
|||
* @retval pointer to descriptor buffer |
|||
*/ |
|||
static uint8_t *USBD_CDC_GetFSCfgDesc(uint16_t *length) |
|||
{ |
|||
USBD_EpDescTypeDef *pEpCmdDesc = USBD_GetEpDesc(USBD_CDC_CfgDesc, CDC_CMD_EP); |
|||
USBD_EpDescTypeDef *pEpOutDesc = USBD_GetEpDesc(USBD_CDC_CfgDesc, CDC_OUT_EP); |
|||
USBD_EpDescTypeDef *pEpInDesc = USBD_GetEpDesc(USBD_CDC_CfgDesc, CDC_IN_EP); |
|||
|
|||
if (pEpCmdDesc != NULL) |
|||
{ |
|||
pEpCmdDesc->bInterval = CDC_FS_BINTERVAL; |
|||
} |
|||
|
|||
if (pEpOutDesc != NULL) |
|||
{ |
|||
pEpOutDesc->wMaxPacketSize = CDC_DATA_FS_MAX_PACKET_SIZE; |
|||
} |
|||
|
|||
if (pEpInDesc != NULL) |
|||
{ |
|||
pEpInDesc->wMaxPacketSize = CDC_DATA_FS_MAX_PACKET_SIZE; |
|||
} |
|||
|
|||
*length = (uint16_t)sizeof(USBD_CDC_CfgDesc); |
|||
return USBD_CDC_CfgDesc; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_GetHSCfgDesc |
|||
* Return configuration descriptor |
|||
* @param length : pointer data length |
|||
* @retval pointer to descriptor buffer |
|||
*/ |
|||
static uint8_t *USBD_CDC_GetHSCfgDesc(uint16_t *length) |
|||
{ |
|||
USBD_EpDescTypeDef *pEpCmdDesc = USBD_GetEpDesc(USBD_CDC_CfgDesc, CDC_CMD_EP); |
|||
USBD_EpDescTypeDef *pEpOutDesc = USBD_GetEpDesc(USBD_CDC_CfgDesc, CDC_OUT_EP); |
|||
USBD_EpDescTypeDef *pEpInDesc = USBD_GetEpDesc(USBD_CDC_CfgDesc, CDC_IN_EP); |
|||
|
|||
if (pEpCmdDesc != NULL) |
|||
{ |
|||
pEpCmdDesc->bInterval = CDC_HS_BINTERVAL; |
|||
} |
|||
|
|||
if (pEpOutDesc != NULL) |
|||
{ |
|||
pEpOutDesc->wMaxPacketSize = CDC_DATA_HS_MAX_PACKET_SIZE; |
|||
} |
|||
|
|||
if (pEpInDesc != NULL) |
|||
{ |
|||
pEpInDesc->wMaxPacketSize = CDC_DATA_HS_MAX_PACKET_SIZE; |
|||
} |
|||
|
|||
*length = (uint16_t)sizeof(USBD_CDC_CfgDesc); |
|||
return USBD_CDC_CfgDesc; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_GetOtherSpeedCfgDesc |
|||
* Return configuration descriptor |
|||
* @param length : pointer data length |
|||
* @retval pointer to descriptor buffer |
|||
*/ |
|||
static uint8_t *USBD_CDC_GetOtherSpeedCfgDesc(uint16_t *length) |
|||
{ |
|||
USBD_EpDescTypeDef *pEpCmdDesc = USBD_GetEpDesc(USBD_CDC_CfgDesc, CDC_CMD_EP); |
|||
USBD_EpDescTypeDef *pEpOutDesc = USBD_GetEpDesc(USBD_CDC_CfgDesc, CDC_OUT_EP); |
|||
USBD_EpDescTypeDef *pEpInDesc = USBD_GetEpDesc(USBD_CDC_CfgDesc, CDC_IN_EP); |
|||
|
|||
if (pEpCmdDesc != NULL) |
|||
{ |
|||
pEpCmdDesc->bInterval = CDC_FS_BINTERVAL; |
|||
} |
|||
|
|||
if (pEpOutDesc != NULL) |
|||
{ |
|||
pEpOutDesc->wMaxPacketSize = CDC_DATA_FS_MAX_PACKET_SIZE; |
|||
} |
|||
|
|||
if (pEpInDesc != NULL) |
|||
{ |
|||
pEpInDesc->wMaxPacketSize = CDC_DATA_FS_MAX_PACKET_SIZE; |
|||
} |
|||
|
|||
*length = (uint16_t)sizeof(USBD_CDC_CfgDesc); |
|||
return USBD_CDC_CfgDesc; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_GetDeviceQualifierDescriptor |
|||
* return Device Qualifier descriptor |
|||
* @param length : pointer data length |
|||
* @retval pointer to descriptor buffer |
|||
*/ |
|||
uint8_t *USBD_CDC_GetDeviceQualifierDescriptor(uint16_t *length) |
|||
{ |
|||
*length = (uint16_t)sizeof(USBD_CDC_DeviceQualifierDesc); |
|||
|
|||
return USBD_CDC_DeviceQualifierDesc; |
|||
} |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
/**
|
|||
* @brief USBD_CDC_RegisterInterface |
|||
* @param pdev: device instance |
|||
* @param fops: CD Interface callback |
|||
* @retval status |
|||
*/ |
|||
uint8_t USBD_CDC_RegisterInterface(USBD_HandleTypeDef *pdev, |
|||
USBD_CDC_ItfTypeDef *fops) |
|||
{ |
|||
if (fops == NULL) |
|||
{ |
|||
return (uint8_t)USBD_FAIL; |
|||
} |
|||
|
|||
pdev->pUserData[pdev->classId] = fops; |
|||
|
|||
return (uint8_t)USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_SetTxBuffer |
|||
* @param pdev: device instance |
|||
* @param pbuff: Tx Buffer |
|||
* @param length: Tx Buffer length |
|||
* @retval status |
|||
*/ |
|||
uint8_t USBD_CDC_SetTxBuffer(USBD_HandleTypeDef *pdev, |
|||
uint8_t *pbuff, uint32_t length) |
|||
{ |
|||
USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef *)pdev->pClassDataCmsit[pdev->classId]; |
|||
|
|||
if (hcdc == NULL) |
|||
{ |
|||
return (uint8_t)USBD_FAIL; |
|||
} |
|||
|
|||
hcdc->TxBuffer = pbuff; |
|||
hcdc->TxLength = length; |
|||
|
|||
return (uint8_t)USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_SetRxBuffer |
|||
* @param pdev: device instance |
|||
* @param pbuff: Rx Buffer |
|||
* @retval status |
|||
*/ |
|||
uint8_t USBD_CDC_SetRxBuffer(USBD_HandleTypeDef *pdev, uint8_t *pbuff) |
|||
{ |
|||
USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef *)pdev->pClassDataCmsit[pdev->classId]; |
|||
|
|||
if (hcdc == NULL) |
|||
{ |
|||
return (uint8_t)USBD_FAIL; |
|||
} |
|||
|
|||
hcdc->RxBuffer = pbuff; |
|||
|
|||
return (uint8_t)USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_TransmitPacket |
|||
* Transmit packet on IN endpoint |
|||
* @param pdev: device instance |
|||
* @retval status |
|||
*/ |
|||
uint8_t USBD_CDC_TransmitPacket(USBD_HandleTypeDef *pdev) |
|||
{ |
|||
USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef *)pdev->pClassDataCmsit[pdev->classId]; |
|||
USBD_StatusTypeDef ret = USBD_BUSY; |
|||
|
|||
#ifdef USE_USBD_COMPOSITE |
|||
/* Get the Endpoints addresses allocated for this class instance */ |
|||
CDCInEpAdd = USBD_CoreGetEPAdd(pdev, USBD_EP_IN, USBD_EP_TYPE_BULK); |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
if (pdev->pClassDataCmsit[pdev->classId] == NULL) |
|||
{ |
|||
return (uint8_t)USBD_FAIL; |
|||
} |
|||
|
|||
if (hcdc->TxState == 0U) |
|||
{ |
|||
/* Tx Transfer in progress */ |
|||
hcdc->TxState = 1U; |
|||
|
|||
/* Update the packet total length */ |
|||
pdev->ep_in[CDCInEpAdd & 0xFU].total_length = hcdc->TxLength; |
|||
|
|||
/* Transmit next packet */ |
|||
(void)USBD_LL_Transmit(pdev, CDCInEpAdd, hcdc->TxBuffer, hcdc->TxLength); |
|||
|
|||
ret = USBD_OK; |
|||
} |
|||
|
|||
return (uint8_t)ret; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CDC_ReceivePacket |
|||
* prepare OUT Endpoint for reception |
|||
* @param pdev: device instance |
|||
* @retval status |
|||
*/ |
|||
uint8_t USBD_CDC_ReceivePacket(USBD_HandleTypeDef *pdev) |
|||
{ |
|||
USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef *)pdev->pClassDataCmsit[pdev->classId]; |
|||
|
|||
#ifdef USE_USBD_COMPOSITE |
|||
/* Get the Endpoints addresses allocated for this class instance */ |
|||
CDCOutEpAdd = USBD_CoreGetEPAdd(pdev, USBD_EP_OUT, USBD_EP_TYPE_BULK); |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
|
|||
if (pdev->pClassDataCmsit[pdev->classId] == NULL) |
|||
{ |
|||
return (uint8_t)USBD_FAIL; |
|||
} |
|||
|
|||
if (pdev->dev_speed == USBD_SPEED_HIGH) |
|||
{ |
|||
/* Prepare Out endpoint to receive next packet */ |
|||
(void)USBD_LL_PrepareReceive(pdev, CDCOutEpAdd, hcdc->RxBuffer, |
|||
CDC_DATA_HS_OUT_PACKET_SIZE); |
|||
} |
|||
else |
|||
{ |
|||
/* Prepare Out endpoint to receive next packet */ |
|||
(void)USBD_LL_PrepareReceive(pdev, CDCOutEpAdd, hcdc->RxBuffer, |
|||
CDC_DATA_FS_OUT_PACKET_SIZE); |
|||
} |
|||
|
|||
return (uint8_t)USBD_OK; |
|||
} |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
@ -0,0 +1,172 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file usbd_core.h |
|||
* @author MCD Application Team |
|||
* @brief Header file for usbd_core.c file |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2015 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __USBD_CORE_H |
|||
#define __USBD_CORE_H |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_conf.h" |
|||
#include "usbd_def.h" |
|||
#include "usbd_ioreq.h" |
|||
#include "usbd_ctlreq.h" |
|||
|
|||
/** @addtogroup STM32_USB_DEVICE_LIBRARY
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CORE
|
|||
* @brief This file is the Header file for usbd_core.c file |
|||
* @{ |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_CORE_Exported_Defines
|
|||
* @{ |
|||
*/ |
|||
#ifndef USBD_DEBUG_LEVEL |
|||
#define USBD_DEBUG_LEVEL 0U |
|||
#endif /* USBD_DEBUG_LEVEL */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_CORE_Exported_TypesDefinitions
|
|||
* @{ |
|||
*/ |
|||
|
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
|
|||
/** @defgroup USBD_CORE_Exported_Macros
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CORE_Exported_Variables
|
|||
* @{ |
|||
*/ |
|||
#define USBD_SOF USBD_LL_SOF |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CORE_Exported_FunctionsPrototype
|
|||
* @{ |
|||
*/ |
|||
USBD_StatusTypeDef USBD_Init(USBD_HandleTypeDef *pdev, USBD_DescriptorsTypeDef *pdesc, uint8_t id); |
|||
USBD_StatusTypeDef USBD_DeInit(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_Start(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_Stop(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_RegisterClass(USBD_HandleTypeDef *pdev, USBD_ClassTypeDef *pclass); |
|||
|
|||
#ifdef USE_USBD_COMPOSITE |
|||
USBD_StatusTypeDef USBD_RegisterClassComposite(USBD_HandleTypeDef *pdev, USBD_ClassTypeDef *pclass, |
|||
USBD_CompositeClassTypeDef classtype, uint8_t *EpAddr); |
|||
|
|||
USBD_StatusTypeDef USBD_UnRegisterClassComposite(USBD_HandleTypeDef *pdev); |
|||
uint8_t USBD_CoreGetEPAdd(USBD_HandleTypeDef *pdev, uint8_t ep_dir, uint8_t ep_type); |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
|
|||
uint8_t USBD_CoreFindIF(USBD_HandleTypeDef *pdev, uint8_t index); |
|||
uint8_t USBD_CoreFindEP(USBD_HandleTypeDef *pdev, uint8_t index); |
|||
|
|||
USBD_StatusTypeDef USBD_RunTestMode(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_SetClassConfig(USBD_HandleTypeDef *pdev, uint8_t cfgidx); |
|||
USBD_StatusTypeDef USBD_ClrClassConfig(USBD_HandleTypeDef *pdev, uint8_t cfgidx); |
|||
|
|||
USBD_StatusTypeDef USBD_LL_SetupStage(USBD_HandleTypeDef *pdev, uint8_t *psetup); |
|||
USBD_StatusTypeDef USBD_LL_DataOutStage(USBD_HandleTypeDef *pdev, uint8_t epnum, uint8_t *pdata); |
|||
USBD_StatusTypeDef USBD_LL_DataInStage(USBD_HandleTypeDef *pdev, uint8_t epnum, uint8_t *pdata); |
|||
|
|||
USBD_StatusTypeDef USBD_LL_Reset(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_LL_SetSpeed(USBD_HandleTypeDef *pdev, USBD_SpeedTypeDef speed); |
|||
USBD_StatusTypeDef USBD_LL_Suspend(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_LL_Resume(USBD_HandleTypeDef *pdev); |
|||
|
|||
USBD_StatusTypeDef USBD_LL_SOF(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_LL_IsoINIncomplete(USBD_HandleTypeDef *pdev, uint8_t epnum); |
|||
USBD_StatusTypeDef USBD_LL_IsoOUTIncomplete(USBD_HandleTypeDef *pdev, uint8_t epnum); |
|||
|
|||
USBD_StatusTypeDef USBD_LL_DevConnected(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_LL_DevDisconnected(USBD_HandleTypeDef *pdev); |
|||
|
|||
/* USBD Low Level Driver */ |
|||
USBD_StatusTypeDef USBD_LL_Init(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_LL_DeInit(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_LL_Start(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_LL_Stop(USBD_HandleTypeDef *pdev); |
|||
|
|||
USBD_StatusTypeDef USBD_LL_OpenEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr, |
|||
uint8_t ep_type, uint16_t ep_mps); |
|||
|
|||
USBD_StatusTypeDef USBD_LL_CloseEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr); |
|||
USBD_StatusTypeDef USBD_LL_FlushEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr); |
|||
USBD_StatusTypeDef USBD_LL_StallEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr); |
|||
USBD_StatusTypeDef USBD_LL_ClearStallEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr); |
|||
USBD_StatusTypeDef USBD_LL_SetUSBAddress(USBD_HandleTypeDef *pdev, uint8_t dev_addr); |
|||
|
|||
USBD_StatusTypeDef USBD_LL_Transmit(USBD_HandleTypeDef *pdev, uint8_t ep_addr, |
|||
uint8_t *pbuf, uint32_t size); |
|||
|
|||
USBD_StatusTypeDef USBD_LL_PrepareReceive(USBD_HandleTypeDef *pdev, uint8_t ep_addr, |
|||
uint8_t *pbuf, uint32_t size); |
|||
|
|||
#ifdef USBD_HS_TESTMODE_ENABLE |
|||
USBD_StatusTypeDef USBD_LL_SetTestMode(USBD_HandleTypeDef *pdev, uint8_t testmode); |
|||
#endif /* USBD_HS_TESTMODE_ENABLE */ |
|||
|
|||
uint8_t USBD_LL_IsStallEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr); |
|||
uint32_t USBD_LL_GetRxDataSize(USBD_HandleTypeDef *pdev, uint8_t ep_addr); |
|||
|
|||
void USBD_LL_Delay(uint32_t Delay); |
|||
|
|||
void *USBD_GetEpDesc(uint8_t *pConfDesc, uint8_t EpAddr); |
|||
USBD_DescHeaderTypeDef *USBD_GetNextDesc(uint8_t *pbuf, uint16_t *ptr); |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* __USBD_CORE_H */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
@ -0,0 +1,101 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file usbd_req.h |
|||
* @author MCD Application Team |
|||
* @brief Header file for the usbd_req.c file |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2015 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __USB_REQUEST_H |
|||
#define __USB_REQUEST_H |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_def.h" |
|||
|
|||
|
|||
/** @addtogroup STM32_USB_DEVICE_LIBRARY
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_REQ
|
|||
* @brief header file for the usbd_req.c file |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_REQ_Exported_Defines
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_REQ_Exported_Types
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
|
|||
/** @defgroup USBD_REQ_Exported_Macros
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_REQ_Exported_Variables
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_REQ_Exported_FunctionsPrototype
|
|||
* @{ |
|||
*/ |
|||
|
|||
USBD_StatusTypeDef USBD_StdDevReq(USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req); |
|||
USBD_StatusTypeDef USBD_StdItfReq(USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req); |
|||
USBD_StatusTypeDef USBD_StdEPReq(USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req); |
|||
|
|||
void USBD_CtlError(USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req); |
|||
void USBD_ParseSetupRequest(USBD_SetupReqTypedef *req, uint8_t *pdata); |
|||
void USBD_GetString(uint8_t *desc, uint8_t *unicode, uint16_t *len); |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* __USB_REQUEST_H */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
@ -0,0 +1,514 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file usbd_def.h |
|||
* @author MCD Application Team |
|||
* @brief General defines for the usb device library |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2015 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __USBD_DEF_H |
|||
#define __USBD_DEF_H |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_conf.h" |
|||
|
|||
/** @addtogroup STM32_USBD_DEVICE_LIBRARY
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USB_DEF
|
|||
* @brief general defines for the usb device library file |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USB_DEF_Exported_Defines
|
|||
* @{ |
|||
*/ |
|||
|
|||
#ifndef NULL |
|||
#define NULL 0U |
|||
#endif /* NULL */ |
|||
|
|||
#ifndef USBD_MAX_NUM_INTERFACES |
|||
#define USBD_MAX_NUM_INTERFACES 1U |
|||
#endif /* USBD_MAX_NUM_CONFIGURATION */ |
|||
|
|||
#ifndef USBD_MAX_NUM_CONFIGURATION |
|||
#define USBD_MAX_NUM_CONFIGURATION 1U |
|||
#endif /* USBD_MAX_NUM_CONFIGURATION */ |
|||
|
|||
#ifdef USE_USBD_COMPOSITE |
|||
#ifndef USBD_MAX_SUPPORTED_CLASS |
|||
#define USBD_MAX_SUPPORTED_CLASS 4U |
|||
#endif /* USBD_MAX_SUPPORTED_CLASS */ |
|||
#else |
|||
#ifndef USBD_MAX_SUPPORTED_CLASS |
|||
#define USBD_MAX_SUPPORTED_CLASS 1U |
|||
#endif /* USBD_MAX_SUPPORTED_CLASS */ |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
|
|||
#ifndef USBD_MAX_CLASS_ENDPOINTS |
|||
#define USBD_MAX_CLASS_ENDPOINTS 5U |
|||
#endif /* USBD_MAX_CLASS_ENDPOINTS */ |
|||
|
|||
#ifndef USBD_MAX_CLASS_INTERFACES |
|||
#define USBD_MAX_CLASS_INTERFACES 5U |
|||
#endif /* USBD_MAX_CLASS_INTERFACES */ |
|||
|
|||
#ifndef USBD_LPM_ENABLED |
|||
#define USBD_LPM_ENABLED 0U |
|||
#endif /* USBD_LPM_ENABLED */ |
|||
|
|||
#ifndef USBD_SELF_POWERED |
|||
#define USBD_SELF_POWERED 1U |
|||
#endif /*USBD_SELF_POWERED */ |
|||
|
|||
#ifndef USBD_MAX_POWER |
|||
#define USBD_MAX_POWER 0x32U /* 100 mA */ |
|||
#endif /* USBD_MAX_POWER */ |
|||
|
|||
#ifndef USBD_SUPPORT_USER_STRING_DESC |
|||
#define USBD_SUPPORT_USER_STRING_DESC 0U |
|||
#endif /* USBD_SUPPORT_USER_STRING_DESC */ |
|||
|
|||
#ifndef USBD_CLASS_USER_STRING_DESC |
|||
#define USBD_CLASS_USER_STRING_DESC 0U |
|||
#endif /* USBD_CLASS_USER_STRING_DESC */ |
|||
|
|||
#define USB_LEN_DEV_QUALIFIER_DESC 0x0AU |
|||
#define USB_LEN_DEV_DESC 0x12U |
|||
#define USB_LEN_CFG_DESC 0x09U |
|||
#define USB_LEN_IF_DESC 0x09U |
|||
#define USB_LEN_EP_DESC 0x07U |
|||
#define USB_LEN_OTG_DESC 0x03U |
|||
#define USB_LEN_LANGID_STR_DESC 0x04U |
|||
#define USB_LEN_OTHER_SPEED_DESC_SIZ 0x09U |
|||
|
|||
#define USBD_IDX_LANGID_STR 0x00U |
|||
#define USBD_IDX_MFC_STR 0x01U |
|||
#define USBD_IDX_PRODUCT_STR 0x02U |
|||
#define USBD_IDX_SERIAL_STR 0x03U |
|||
#define USBD_IDX_CONFIG_STR 0x04U |
|||
#define USBD_IDX_INTERFACE_STR 0x05U |
|||
|
|||
#define USB_REQ_TYPE_STANDARD 0x00U |
|||
#define USB_REQ_TYPE_CLASS 0x20U |
|||
#define USB_REQ_TYPE_VENDOR 0x40U |
|||
#define USB_REQ_TYPE_MASK 0x60U |
|||
|
|||
#define USB_REQ_RECIPIENT_DEVICE 0x00U |
|||
#define USB_REQ_RECIPIENT_INTERFACE 0x01U |
|||
#define USB_REQ_RECIPIENT_ENDPOINT 0x02U |
|||
#define USB_REQ_RECIPIENT_MASK 0x03U |
|||
|
|||
#define USB_REQ_GET_STATUS 0x00U |
|||
#define USB_REQ_CLEAR_FEATURE 0x01U |
|||
#define USB_REQ_SET_FEATURE 0x03U |
|||
#define USB_REQ_SET_ADDRESS 0x05U |
|||
#define USB_REQ_GET_DESCRIPTOR 0x06U |
|||
#define USB_REQ_SET_DESCRIPTOR 0x07U |
|||
#define USB_REQ_GET_CONFIGURATION 0x08U |
|||
#define USB_REQ_SET_CONFIGURATION 0x09U |
|||
#define USB_REQ_GET_INTERFACE 0x0AU |
|||
#define USB_REQ_SET_INTERFACE 0x0BU |
|||
#define USB_REQ_SYNCH_FRAME 0x0CU |
|||
|
|||
#define USB_DESC_TYPE_DEVICE 0x01U |
|||
#define USB_DESC_TYPE_CONFIGURATION 0x02U |
|||
#define USB_DESC_TYPE_STRING 0x03U |
|||
#define USB_DESC_TYPE_INTERFACE 0x04U |
|||
#define USB_DESC_TYPE_ENDPOINT 0x05U |
|||
#define USB_DESC_TYPE_DEVICE_QUALIFIER 0x06U |
|||
#define USB_DESC_TYPE_OTHER_SPEED_CONFIGURATION 0x07U |
|||
#define USB_DESC_TYPE_IAD 0x0BU |
|||
#define USB_DESC_TYPE_BOS 0x0FU |
|||
|
|||
#define USB_CONFIG_REMOTE_WAKEUP 0x02U |
|||
#define USB_CONFIG_SELF_POWERED 0x01U |
|||
|
|||
#define USB_FEATURE_EP_HALT 0x00U |
|||
#define USB_FEATURE_REMOTE_WAKEUP 0x01U |
|||
#define USB_FEATURE_TEST_MODE 0x02U |
|||
|
|||
#define USB_DEVICE_CAPABITY_TYPE 0x10U |
|||
|
|||
#define USB_CONF_DESC_SIZE 0x09U |
|||
#define USB_IF_DESC_SIZE 0x09U |
|||
#define USB_EP_DESC_SIZE 0x07U |
|||
#define USB_IAD_DESC_SIZE 0x08U |
|||
|
|||
#define USB_HS_MAX_PACKET_SIZE 512U |
|||
#define USB_FS_MAX_PACKET_SIZE 64U |
|||
#define USB_MAX_EP0_SIZE 64U |
|||
|
|||
/* Device Status */ |
|||
#define USBD_STATE_DEFAULT 0x01U |
|||
#define USBD_STATE_ADDRESSED 0x02U |
|||
#define USBD_STATE_CONFIGURED 0x03U |
|||
#define USBD_STATE_SUSPENDED 0x04U |
|||
|
|||
|
|||
/* EP0 State */ |
|||
#define USBD_EP0_IDLE 0x00U |
|||
#define USBD_EP0_SETUP 0x01U |
|||
#define USBD_EP0_DATA_IN 0x02U |
|||
#define USBD_EP0_DATA_OUT 0x03U |
|||
#define USBD_EP0_STATUS_IN 0x04U |
|||
#define USBD_EP0_STATUS_OUT 0x05U |
|||
#define USBD_EP0_STALL 0x06U |
|||
|
|||
#define USBD_EP_TYPE_CTRL 0x00U |
|||
#define USBD_EP_TYPE_ISOC 0x01U |
|||
#define USBD_EP_TYPE_BULK 0x02U |
|||
#define USBD_EP_TYPE_INTR 0x03U |
|||
|
|||
#ifdef USE_USBD_COMPOSITE |
|||
#define USBD_EP_IN 0x80U |
|||
#define USBD_EP_OUT 0x00U |
|||
#define USBD_FUNC_DESCRIPTOR_TYPE 0x24U |
|||
#define USBD_DESC_SUBTYPE_ACM 0x0FU |
|||
#define USBD_DESC_ECM_BCD_LOW 0x00U |
|||
#define USBD_DESC_ECM_BCD_HIGH 0x10U |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_DEF_Exported_TypesDefinitions
|
|||
* @{ |
|||
*/ |
|||
|
|||
typedef struct usb_setup_req |
|||
{ |
|||
uint8_t bmRequest; |
|||
uint8_t bRequest; |
|||
uint16_t wValue; |
|||
uint16_t wIndex; |
|||
uint16_t wLength; |
|||
} USBD_SetupReqTypedef; |
|||
|
|||
typedef struct |
|||
{ |
|||
uint8_t bLength; |
|||
uint8_t bDescriptorType; |
|||
uint16_t wTotalLength; |
|||
uint8_t bNumInterfaces; |
|||
uint8_t bConfigurationValue; |
|||
uint8_t iConfiguration; |
|||
uint8_t bmAttributes; |
|||
uint8_t bMaxPower; |
|||
} __PACKED USBD_ConfigDescTypeDef; |
|||
|
|||
typedef struct |
|||
{ |
|||
uint8_t bLength; |
|||
uint8_t bDescriptorType; |
|||
uint16_t wTotalLength; |
|||
uint8_t bNumDeviceCaps; |
|||
} USBD_BosDescTypeDef; |
|||
|
|||
typedef struct |
|||
{ |
|||
uint8_t bLength; |
|||
uint8_t bDescriptorType; |
|||
uint8_t bEndpointAddress; |
|||
uint8_t bmAttributes; |
|||
uint16_t wMaxPacketSize; |
|||
uint8_t bInterval; |
|||
} __PACKED USBD_EpDescTypeDef; |
|||
|
|||
typedef struct |
|||
{ |
|||
uint8_t bLength; |
|||
uint8_t bDescriptorType; |
|||
uint8_t bDescriptorSubType; |
|||
} USBD_DescHeaderTypeDef; |
|||
|
|||
struct _USBD_HandleTypeDef; |
|||
|
|||
typedef struct _Device_cb |
|||
{ |
|||
uint8_t (*Init)(struct _USBD_HandleTypeDef *pdev, uint8_t cfgidx); |
|||
uint8_t (*DeInit)(struct _USBD_HandleTypeDef *pdev, uint8_t cfgidx); |
|||
/* Control Endpoints*/ |
|||
uint8_t (*Setup)(struct _USBD_HandleTypeDef *pdev, USBD_SetupReqTypedef *req); |
|||
uint8_t (*EP0_TxSent)(struct _USBD_HandleTypeDef *pdev); |
|||
uint8_t (*EP0_RxReady)(struct _USBD_HandleTypeDef *pdev); |
|||
/* Class Specific Endpoints*/ |
|||
uint8_t (*DataIn)(struct _USBD_HandleTypeDef *pdev, uint8_t epnum); |
|||
uint8_t (*DataOut)(struct _USBD_HandleTypeDef *pdev, uint8_t epnum); |
|||
uint8_t (*SOF)(struct _USBD_HandleTypeDef *pdev); |
|||
uint8_t (*IsoINIncomplete)(struct _USBD_HandleTypeDef *pdev, uint8_t epnum); |
|||
uint8_t (*IsoOUTIncomplete)(struct _USBD_HandleTypeDef *pdev, uint8_t epnum); |
|||
|
|||
uint8_t *(*GetHSConfigDescriptor)(uint16_t *length); |
|||
uint8_t *(*GetFSConfigDescriptor)(uint16_t *length); |
|||
uint8_t *(*GetOtherSpeedConfigDescriptor)(uint16_t *length); |
|||
uint8_t *(*GetDeviceQualifierDescriptor)(uint16_t *length); |
|||
#if (USBD_SUPPORT_USER_STRING_DESC == 1U) |
|||
uint8_t *(*GetUsrStrDescriptor)(struct _USBD_HandleTypeDef *pdev, uint8_t index, uint16_t *length); |
|||
#endif /* USBD_SUPPORT_USER_STRING_DESC */ |
|||
|
|||
} USBD_ClassTypeDef; |
|||
|
|||
/* Following USB Device Speed */ |
|||
typedef enum |
|||
{ |
|||
USBD_SPEED_HIGH = 0U, |
|||
USBD_SPEED_FULL = 1U, |
|||
USBD_SPEED_LOW = 2U, |
|||
} USBD_SpeedTypeDef; |
|||
|
|||
/* Following USB Device status */ |
|||
typedef enum |
|||
{ |
|||
USBD_OK = 0U, |
|||
USBD_BUSY, |
|||
USBD_EMEM, |
|||
USBD_FAIL, |
|||
} USBD_StatusTypeDef; |
|||
|
|||
/* USB Device descriptors structure */ |
|||
typedef struct |
|||
{ |
|||
uint8_t *(*GetDeviceDescriptor)(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t *(*GetLangIDStrDescriptor)(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t *(*GetManufacturerStrDescriptor)(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t *(*GetProductStrDescriptor)(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t *(*GetSerialStrDescriptor)(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t *(*GetConfigurationStrDescriptor)(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t *(*GetInterfaceStrDescriptor)(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
#if (USBD_CLASS_USER_STRING_DESC == 1) |
|||
uint8_t *(*GetUserStrDescriptor)(USBD_SpeedTypeDef speed, uint8_t idx, uint16_t *length); |
|||
#endif /* USBD_CLASS_USER_STRING_DESC */ |
|||
#if ((USBD_LPM_ENABLED == 1U) || (USBD_CLASS_BOS_ENABLED == 1)) |
|||
uint8_t *(*GetBOSDescriptor)(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
#endif /* (USBD_LPM_ENABLED == 1U) || (USBD_CLASS_BOS_ENABLED == 1) */ |
|||
} USBD_DescriptorsTypeDef; |
|||
|
|||
/* USB Device handle structure */ |
|||
typedef struct |
|||
{ |
|||
uint32_t status; |
|||
uint32_t total_length; |
|||
uint32_t rem_length; |
|||
uint32_t maxpacket; |
|||
uint16_t is_used; |
|||
uint16_t bInterval; |
|||
} USBD_EndpointTypeDef; |
|||
|
|||
#ifdef USE_USBD_COMPOSITE |
|||
typedef enum |
|||
{ |
|||
CLASS_TYPE_NONE = 0, |
|||
CLASS_TYPE_HID = 1, |
|||
CLASS_TYPE_CDC = 2, |
|||
CLASS_TYPE_MSC = 3, |
|||
CLASS_TYPE_DFU = 4, |
|||
CLASS_TYPE_CHID = 5, |
|||
CLASS_TYPE_AUDIO = 6, |
|||
CLASS_TYPE_ECM = 7, |
|||
CLASS_TYPE_RNDIS = 8, |
|||
CLASS_TYPE_MTP = 9, |
|||
CLASS_TYPE_VIDEO = 10, |
|||
CLASS_TYPE_PRINTER = 11, |
|||
CLASS_TYPE_CCID = 12, |
|||
} USBD_CompositeClassTypeDef; |
|||
|
|||
|
|||
/* USB Device handle structure */ |
|||
typedef struct |
|||
{ |
|||
uint8_t add; |
|||
uint8_t type; |
|||
uint8_t size; |
|||
uint8_t is_used; |
|||
} USBD_EPTypeDef; |
|||
|
|||
/* USB Device handle structure */ |
|||
typedef struct |
|||
{ |
|||
USBD_CompositeClassTypeDef ClassType; |
|||
uint32_t ClassId; |
|||
uint32_t Active; |
|||
uint32_t NumEps; |
|||
USBD_EPTypeDef Eps[USBD_MAX_CLASS_ENDPOINTS]; |
|||
uint8_t *EpAdd; |
|||
uint32_t NumIf; |
|||
uint8_t Ifs[USBD_MAX_CLASS_INTERFACES]; |
|||
uint32_t CurrPcktSze; |
|||
} USBD_CompositeElementTypeDef; |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
|
|||
/* USB Device handle structure */ |
|||
typedef struct _USBD_HandleTypeDef |
|||
{ |
|||
uint8_t id; |
|||
uint32_t dev_config; |
|||
uint32_t dev_default_config; |
|||
uint32_t dev_config_status; |
|||
USBD_SpeedTypeDef dev_speed; |
|||
USBD_EndpointTypeDef ep_in[16]; |
|||
USBD_EndpointTypeDef ep_out[16]; |
|||
__IO uint32_t ep0_state; |
|||
uint32_t ep0_data_len; |
|||
__IO uint8_t dev_state; |
|||
__IO uint8_t dev_old_state; |
|||
uint8_t dev_address; |
|||
uint8_t dev_connection_status; |
|||
uint8_t dev_test_mode; |
|||
uint32_t dev_remote_wakeup; |
|||
uint8_t ConfIdx; |
|||
|
|||
USBD_SetupReqTypedef request; |
|||
USBD_DescriptorsTypeDef *pDesc; |
|||
USBD_ClassTypeDef *pClass[USBD_MAX_SUPPORTED_CLASS]; |
|||
void *pClassData; |
|||
void *pClassDataCmsit[USBD_MAX_SUPPORTED_CLASS]; |
|||
void *pUserData[USBD_MAX_SUPPORTED_CLASS]; |
|||
void *pData; |
|||
void *pBosDesc; |
|||
void *pConfDesc; |
|||
uint32_t classId; |
|||
uint32_t NumClasses; |
|||
#ifdef USE_USBD_COMPOSITE |
|||
USBD_CompositeElementTypeDef tclasslist[USBD_MAX_SUPPORTED_CLASS]; |
|||
#endif /* USE_USBD_COMPOSITE */ |
|||
} USBD_HandleTypeDef; |
|||
|
|||
/* USB Device endpoint direction */ |
|||
typedef enum |
|||
{ |
|||
OUT = 0x00, |
|||
IN = 0x80, |
|||
} USBD_EPDirectionTypeDef; |
|||
|
|||
typedef enum |
|||
{ |
|||
NETWORK_CONNECTION = 0x00, |
|||
RESPONSE_AVAILABLE = 0x01, |
|||
CONNECTION_SPEED_CHANGE = 0x2A |
|||
} USBD_CDC_NotifCodeTypeDef; |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
|
|||
/** @defgroup USBD_DEF_Exported_Macros
|
|||
* @{ |
|||
*/ |
|||
__STATIC_INLINE uint16_t SWAPBYTE(uint8_t *addr) |
|||
{ |
|||
uint16_t _SwapVal, _Byte1, _Byte2; |
|||
uint8_t *_pbuff = addr; |
|||
|
|||
_Byte1 = *(uint8_t *)_pbuff; |
|||
_pbuff++; |
|||
_Byte2 = *(uint8_t *)_pbuff; |
|||
|
|||
_SwapVal = (_Byte2 << 8) | _Byte1; |
|||
|
|||
return _SwapVal; |
|||
} |
|||
|
|||
#ifndef LOBYTE |
|||
#define LOBYTE(x) ((uint8_t)((x) & 0x00FFU)) |
|||
#endif /* LOBYTE */ |
|||
|
|||
#ifndef HIBYTE |
|||
#define HIBYTE(x) ((uint8_t)(((x) & 0xFF00U) >> 8U)) |
|||
#endif /* HIBYTE */ |
|||
|
|||
#ifndef MIN |
|||
#define MIN(a, b) (((a) < (b)) ? (a) : (b)) |
|||
#endif /* MIN */ |
|||
|
|||
#ifndef MAX |
|||
#define MAX(a, b) (((a) > (b)) ? (a) : (b)) |
|||
#endif /* MAX */ |
|||
|
|||
#if defined ( __GNUC__ ) |
|||
#ifndef __weak |
|||
#define __weak __attribute__((weak)) |
|||
#endif /* __weak */ |
|||
#ifndef __packed |
|||
#define __packed __attribute__((__packed__)) |
|||
#endif /* __packed */ |
|||
#endif /* __GNUC__ */ |
|||
|
|||
|
|||
/* In HS mode and when the DMA is used, all variables and data structures dealing
|
|||
with the DMA during the transaction process should be 4-bytes aligned */ |
|||
|
|||
#if defined ( __GNUC__ ) && !defined (__CC_ARM) /* GNU Compiler */ |
|||
#ifndef __ALIGN_END |
|||
#define __ALIGN_END __attribute__ ((aligned (4U))) |
|||
#endif /* __ALIGN_END */ |
|||
#ifndef __ALIGN_BEGIN |
|||
#define __ALIGN_BEGIN |
|||
#endif /* __ALIGN_BEGIN */ |
|||
#else |
|||
#ifndef __ALIGN_END |
|||
#define __ALIGN_END |
|||
#endif /* __ALIGN_END */ |
|||
#ifndef __ALIGN_BEGIN |
|||
#if defined (__CC_ARM) /* ARM Compiler */ |
|||
#define __ALIGN_BEGIN __align(4U) |
|||
#elif defined (__ICCARM__) /* IAR Compiler */ |
|||
#define __ALIGN_BEGIN |
|||
#endif /* __CC_ARM */ |
|||
#endif /* __ALIGN_BEGIN */ |
|||
#endif /* __GNUC__ */ |
|||
|
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DEF_Exported_Variables
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DEF_Exported_FunctionsPrototype
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* __USBD_DEF_H */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
@ -0,0 +1,113 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file usbd_ioreq.h |
|||
* @author MCD Application Team |
|||
* @brief Header file for the usbd_ioreq.c file |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2015 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __USBD_IOREQ_H |
|||
#define __USBD_IOREQ_H |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_def.h" |
|||
#include "usbd_core.h" |
|||
|
|||
/** @addtogroup STM32_USB_DEVICE_LIBRARY
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_IOREQ
|
|||
* @brief header file for the usbd_ioreq.c file |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_IOREQ_Exported_Defines
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_IOREQ_Exported_Types
|
|||
* @{ |
|||
*/ |
|||
|
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
|
|||
/** @defgroup USBD_IOREQ_Exported_Macros
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_IOREQ_Exported_Variables
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_IOREQ_Exported_FunctionsPrototype
|
|||
* @{ |
|||
*/ |
|||
|
|||
USBD_StatusTypeDef USBD_CtlSendData(USBD_HandleTypeDef *pdev, |
|||
uint8_t *pbuf, uint32_t len); |
|||
|
|||
USBD_StatusTypeDef USBD_CtlContinueSendData(USBD_HandleTypeDef *pdev, |
|||
uint8_t *pbuf, uint32_t len); |
|||
|
|||
USBD_StatusTypeDef USBD_CtlPrepareRx(USBD_HandleTypeDef *pdev, |
|||
uint8_t *pbuf, uint32_t len); |
|||
|
|||
USBD_StatusTypeDef USBD_CtlContinueRx(USBD_HandleTypeDef *pdev, |
|||
uint8_t *pbuf, uint32_t len); |
|||
|
|||
USBD_StatusTypeDef USBD_CtlSendStatus(USBD_HandleTypeDef *pdev); |
|||
USBD_StatusTypeDef USBD_CtlReceiveStatus(USBD_HandleTypeDef *pdev); |
|||
|
|||
uint32_t USBD_GetRxCount(USBD_HandleTypeDef *pdev, uint8_t ep_addr); |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* __USBD_IOREQ_H */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
File diff suppressed because it is too large
File diff suppressed because it is too large
@ -0,0 +1,224 @@ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file usbd_ioreq.c |
|||
* @author MCD Application Team |
|||
* @brief This file provides the IO requests APIs for control endpoints. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2015 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_ioreq.h" |
|||
|
|||
/** @addtogroup STM32_USB_DEVICE_LIBRARY
|
|||
* @{ |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_IOREQ
|
|||
* @brief control I/O requests module |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_IOREQ_Private_TypesDefinitions
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_IOREQ_Private_Defines
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_IOREQ_Private_Macros
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_IOREQ_Private_Variables
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_IOREQ_Private_FunctionPrototypes
|
|||
* @{ |
|||
*/ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/** @defgroup USBD_IOREQ_Private_Functions
|
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @brief USBD_CtlSendData |
|||
* send data on the ctl pipe |
|||
* @param pdev: device instance |
|||
* @param buff: pointer to data buffer |
|||
* @param len: length of data to be sent |
|||
* @retval status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_CtlSendData(USBD_HandleTypeDef *pdev, |
|||
uint8_t *pbuf, uint32_t len) |
|||
{ |
|||
/* Set EP0 State */ |
|||
pdev->ep0_state = USBD_EP0_DATA_IN; |
|||
pdev->ep_in[0].total_length = len; |
|||
|
|||
#ifdef USBD_AVOID_PACKET_SPLIT_MPS |
|||
pdev->ep_in[0].rem_length = 0U; |
|||
#else |
|||
pdev->ep_in[0].rem_length = len; |
|||
#endif /* USBD_AVOID_PACKET_SPLIT_MPS */ |
|||
|
|||
/* Start the transfer */ |
|||
(void)USBD_LL_Transmit(pdev, 0x00U, pbuf, len); |
|||
|
|||
return USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CtlContinueSendData |
|||
* continue sending data on the ctl pipe |
|||
* @param pdev: device instance |
|||
* @param buff: pointer to data buffer |
|||
* @param len: length of data to be sent |
|||
* @retval status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_CtlContinueSendData(USBD_HandleTypeDef *pdev, |
|||
uint8_t *pbuf, uint32_t len) |
|||
{ |
|||
/* Start the next transfer */ |
|||
(void)USBD_LL_Transmit(pdev, 0x00U, pbuf, len); |
|||
|
|||
return USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CtlPrepareRx |
|||
* receive data on the ctl pipe |
|||
* @param pdev: device instance |
|||
* @param buff: pointer to data buffer |
|||
* @param len: length of data to be received |
|||
* @retval status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_CtlPrepareRx(USBD_HandleTypeDef *pdev, |
|||
uint8_t *pbuf, uint32_t len) |
|||
{ |
|||
/* Set EP0 State */ |
|||
pdev->ep0_state = USBD_EP0_DATA_OUT; |
|||
pdev->ep_out[0].total_length = len; |
|||
|
|||
#ifdef USBD_AVOID_PACKET_SPLIT_MPS |
|||
pdev->ep_out[0].rem_length = 0U; |
|||
#else |
|||
pdev->ep_out[0].rem_length = len; |
|||
#endif /* USBD_AVOID_PACKET_SPLIT_MPS */ |
|||
|
|||
/* Start the transfer */ |
|||
(void)USBD_LL_PrepareReceive(pdev, 0U, pbuf, len); |
|||
|
|||
return USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CtlContinueRx |
|||
* continue receive data on the ctl pipe |
|||
* @param pdev: device instance |
|||
* @param buff: pointer to data buffer |
|||
* @param len: length of data to be received |
|||
* @retval status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_CtlContinueRx(USBD_HandleTypeDef *pdev, |
|||
uint8_t *pbuf, uint32_t len) |
|||
{ |
|||
(void)USBD_LL_PrepareReceive(pdev, 0U, pbuf, len); |
|||
|
|||
return USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CtlSendStatus |
|||
* send zero lzngth packet on the ctl pipe |
|||
* @param pdev: device instance |
|||
* @retval status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_CtlSendStatus(USBD_HandleTypeDef *pdev) |
|||
{ |
|||
/* Set EP0 State */ |
|||
pdev->ep0_state = USBD_EP0_STATUS_IN; |
|||
|
|||
/* Start the transfer */ |
|||
(void)USBD_LL_Transmit(pdev, 0x00U, NULL, 0U); |
|||
|
|||
return USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_CtlReceiveStatus |
|||
* receive zero lzngth packet on the ctl pipe |
|||
* @param pdev: device instance |
|||
* @retval status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_CtlReceiveStatus(USBD_HandleTypeDef *pdev) |
|||
{ |
|||
/* Set EP0 State */ |
|||
pdev->ep0_state = USBD_EP0_STATUS_OUT; |
|||
|
|||
/* Start the transfer */ |
|||
(void)USBD_LL_PrepareReceive(pdev, 0U, NULL, 0U); |
|||
|
|||
return USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief USBD_GetRxCount |
|||
* returns the received data length |
|||
* @param pdev: device instance |
|||
* @param ep_addr: endpoint address |
|||
* @retval Rx Data blength |
|||
*/ |
|||
uint32_t USBD_GetRxCount(USBD_HandleTypeDef *pdev, uint8_t ep_addr) |
|||
{ |
|||
return USBD_LL_GetRxDataSize(pdev, ep_addr); |
|||
} |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
@ -0,0 +1,86 @@ |
|||
This software component is provided to you as part of a software package and |
|||
applicable license terms are in the Package_license file. If you received this |
|||
software component outside of a package or without applicable license terms, |
|||
the terms of the SLA0044 license shall apply and are fully reproduced below: |
|||
|
|||
SLA0044 Rev5/February 2018 |
|||
|
|||
Software license agreement |
|||
|
|||
ULTIMATE LIBERTY SOFTWARE LICENSE AGREEMENT |
|||
|
|||
BY INSTALLING, COPYING, DOWNLOADING, ACCESSING OR OTHERWISE USING THIS SOFTWARE |
|||
OR ANY PART THEREOF (AND THE RELATED DOCUMENTATION) FROM STMICROELECTRONICS |
|||
INTERNATIONAL N.V, SWISS BRANCH AND/OR ITS AFFILIATED COMPANIES |
|||
(STMICROELECTRONICS), THE RECIPIENT, ON BEHALF OF HIMSELF OR HERSELF, OR ON |
|||
BEHALF OF ANY ENTITY BY WHICH SUCH RECIPIENT IS EMPLOYED AND/OR ENGAGED AGREES |
|||
TO BE BOUND BY THIS SOFTWARE LICENSE AGREEMENT. |
|||
|
|||
Under STMicroelectronics’ intellectual property rights, the redistribution, |
|||
reproduction and use in source and binary forms of the software or any part |
|||
thereof, with or without modification, are permitted provided that the following |
|||
conditions are met: |
|||
|
|||
1. Redistribution of source code (modified or not) must retain any copyright |
|||
notice, this list of conditions and the disclaimer set forth below as items 10 |
|||
and 11. |
|||
|
|||
2. Redistributions in binary form, except as embedded into microcontroller or |
|||
microprocessor device manufactured by or for STMicroelectronics or a software |
|||
update for such device, must reproduce any copyright notice provided with the |
|||
binary code, this list of conditions, and the disclaimer set forth below as |
|||
items 10 and 11, in documentation and/or other materials provided with the |
|||
distribution. |
|||
|
|||
3. Neither the name of STMicroelectronics nor the names of other contributors to |
|||
this software may be used to endorse or promote products derived from this |
|||
software or part thereof without specific written permission. |
|||
|
|||
4. This software or any part thereof, including modifications and/or derivative |
|||
works of this software, must be used and execute solely and exclusively on or in |
|||
combination with a microcontroller or microprocessor device manufactured by or |
|||
for STMicroelectronics. |
|||
|
|||
5. No use, reproduction or redistribution of this software partially or totally |
|||
may be done in any manner that would subject this software to any Open Source |
|||
Terms. “Open Source Terms” shall mean any open source license which requires as |
|||
part of distribution of software that the source code of such software is |
|||
distributed therewith or otherwise made available, or open source license that |
|||
substantially complies with the Open Source definition specified at |
|||
www.opensource.org and any other comparable open source license such as for |
|||
example GNU General Public License (GPL), Eclipse Public License (EPL), Apache |
|||
Software License, BSD license or MIT license. |
|||
|
|||
6. STMicroelectronics has no obligation to provide any maintenance, support or |
|||
updates for the software. |
|||
|
|||
7. The software is and will remain the exclusive property of STMicroelectronics |
|||
and its licensors. The recipient will not take any action that jeopardizes |
|||
STMicroelectronics and its licensors' proprietary rights or acquire any rights |
|||
in the software, except the limited rights specified hereunder. |
|||
|
|||
8. The recipient shall comply with all applicable laws and regulations affecting |
|||
the use of the software or any part thereof including any applicable export |
|||
control law or regulation. |
|||
|
|||
9. Redistribution and use of this software or any part thereof other than as |
|||
permitted under this license is void and will automatically terminate your |
|||
rights under this license. |
|||
|
|||
10. THIS SOFTWARE IS PROVIDED BY STMICROELECTRONICS AND CONTRIBUTORS "AS IS" AND |
|||
ANY EXPRESS, IMPLIED OR STATUTORY WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE |
|||
IMPLIED WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND |
|||
NON-INFRINGEMENT OF THIRD PARTY INTELLECTUAL PROPERTY RIGHTS, WHICH ARE |
|||
DISCLAIMED TO THE FULLEST EXTENT PERMITTED BY LAW. IN NO EVENT SHALL |
|||
STMICROELECTRONICS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, |
|||
INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT |
|||
LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR |
|||
PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF |
|||
LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE |
|||
OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF |
|||
ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. |
|||
|
|||
11. EXCEPT AS EXPRESSLY PERMITTED HEREUNDER, NO LICENSE OR OTHER RIGHTS, WHETHER |
|||
EXPRESS OR IMPLIED, ARE GRANTED UNDER ANY PATENT OR OTHER INTELLECTUAL PROPERTY |
|||
RIGHTS OF STMICROELECTRONICS OR ANY THIRD PARTY. |
|||
|
@ -0,0 +1,100 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file : usb_device.c |
|||
* @version : v1.0_Cube |
|||
* @brief : This file implements the USB Device |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
|
|||
#include "usb_device.h" |
|||
#include "usbd_core.h" |
|||
#include "usbd_desc.h" |
|||
#include "usbd_cdc.h" |
|||
#include "usbd_cdc_if.h" |
|||
|
|||
/* USER CODE BEGIN Includes */ |
|||
|
|||
/* USER CODE END Includes */ |
|||
|
|||
/* USER CODE BEGIN PV */ |
|||
/* Private variables ---------------------------------------------------------*/ |
|||
|
|||
/* USER CODE END PV */ |
|||
|
|||
/* USER CODE BEGIN PFP */ |
|||
/* Private function prototypes -----------------------------------------------*/ |
|||
|
|||
/* USER CODE END PFP */ |
|||
|
|||
/* USB Device Core handle declaration. */ |
|||
USBD_HandleTypeDef hUsbDeviceFS; |
|||
|
|||
/*
|
|||
* -- Insert your variables declaration here -- |
|||
*/ |
|||
/* USER CODE BEGIN 0 */ |
|||
|
|||
/* USER CODE END 0 */ |
|||
|
|||
/*
|
|||
* -- Insert your external function declaration here -- |
|||
*/ |
|||
/* USER CODE BEGIN 1 */ |
|||
|
|||
/* USER CODE END 1 */ |
|||
|
|||
/**
|
|||
* Init USB device Library, add supported class and start the library |
|||
* @retval None |
|||
*/ |
|||
void MX_USB_DEVICE_Init(void) |
|||
{ |
|||
/* USER CODE BEGIN USB_DEVICE_Init_PreTreatment */ |
|||
|
|||
/* USER CODE END USB_DEVICE_Init_PreTreatment */ |
|||
|
|||
/* Init Device Library, add supported class and start the library. */ |
|||
if (USBD_Init(&hUsbDeviceFS, &FS_Desc, DEVICE_FS) != USBD_OK) |
|||
{ |
|||
Error_Handler(); |
|||
} |
|||
if (USBD_RegisterClass(&hUsbDeviceFS, &USBD_CDC) != USBD_OK) |
|||
{ |
|||
Error_Handler(); |
|||
} |
|||
if (USBD_CDC_RegisterInterface(&hUsbDeviceFS, &USBD_Interface_fops_FS) != USBD_OK) |
|||
{ |
|||
Error_Handler(); |
|||
} |
|||
if (USBD_Start(&hUsbDeviceFS) != USBD_OK) |
|||
{ |
|||
Error_Handler(); |
|||
} |
|||
|
|||
/* USER CODE BEGIN USB_DEVICE_Init_PostTreatment */ |
|||
|
|||
/* USER CODE END USB_DEVICE_Init_PostTreatment */ |
|||
} |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
@ -0,0 +1,102 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file : usb_device.h |
|||
* @version : v1.0_Cube |
|||
* @brief : Header for usb_device.c file. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __USB_DEVICE__H__ |
|||
#define __USB_DEVICE__H__ |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "stm32f4xx.h" |
|||
#include "stm32f4xx_hal.h" |
|||
#include "usbd_def.h" |
|||
|
|||
/* USER CODE BEGIN INCLUDE */ |
|||
|
|||
/* USER CODE END INCLUDE */ |
|||
|
|||
/** @addtogroup USBD_OTG_DRIVER
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DEVICE USBD_DEVICE
|
|||
* @brief Device file for Usb otg low level driver. |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DEVICE_Exported_Variables USBD_DEVICE_Exported_Variables
|
|||
* @brief Public variables. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* Private variables ---------------------------------------------------------*/ |
|||
/* USER CODE BEGIN PV */ |
|||
|
|||
/* USER CODE END PV */ |
|||
|
|||
/* Private function prototypes -----------------------------------------------*/ |
|||
/* USER CODE BEGIN PFP */ |
|||
|
|||
/* USER CODE END PFP */ |
|||
|
|||
/*
|
|||
* -- Insert your variables declaration here -- |
|||
*/ |
|||
/* USER CODE BEGIN VARIABLES */ |
|||
|
|||
/* USER CODE END VARIABLES */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DEVICE_Exported_FunctionsPrototype USBD_DEVICE_Exported_FunctionsPrototype
|
|||
* @brief Declaration of public functions for Usb device. |
|||
* @{ |
|||
*/ |
|||
|
|||
/** USB Device initialization function. */ |
|||
void MX_USB_DEVICE_Init(void); |
|||
|
|||
/*
|
|||
* -- Insert functions declaration here -- |
|||
*/ |
|||
/* USER CODE BEGIN FD */ |
|||
|
|||
/* USER CODE END FD */ |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* __USB_DEVICE__H__ */ |
@ -0,0 +1,328 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file : usbd_cdc_if.c |
|||
* @version : v1.0_Cube |
|||
* @brief : Usb device for Virtual Com Port. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_cdc_if.h" |
|||
|
|||
/* USER CODE BEGIN INCLUDE */ |
|||
|
|||
/* USER CODE END INCLUDE */ |
|||
|
|||
/* Private typedef -----------------------------------------------------------*/ |
|||
/* Private define ------------------------------------------------------------*/ |
|||
/* Private macro -------------------------------------------------------------*/ |
|||
|
|||
/* USER CODE BEGIN PV */ |
|||
/* Private variables ---------------------------------------------------------*/ |
|||
|
|||
/* USER CODE END PV */ |
|||
|
|||
/** @addtogroup STM32_USB_OTG_DEVICE_LIBRARY
|
|||
* @brief Usb device library. |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @addtogroup USBD_CDC_IF
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Private_TypesDefinitions USBD_CDC_IF_Private_TypesDefinitions
|
|||
* @brief Private types. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN PRIVATE_TYPES */ |
|||
|
|||
/* USER CODE END PRIVATE_TYPES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Private_Defines USBD_CDC_IF_Private_Defines
|
|||
* @brief Private defines. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN PRIVATE_DEFINES */ |
|||
/* USER CODE END PRIVATE_DEFINES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Private_Macros USBD_CDC_IF_Private_Macros
|
|||
* @brief Private macros. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN PRIVATE_MACRO */ |
|||
|
|||
/* USER CODE END PRIVATE_MACRO */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Private_Variables USBD_CDC_IF_Private_Variables
|
|||
* @brief Private variables. |
|||
* @{ |
|||
*/ |
|||
/* Create buffer for reception and transmission */ |
|||
/* It's up to user to redefine and/or remove those define */ |
|||
/** Received data over USB are stored in this buffer */ |
|||
uint8_t UserRxBufferFS[APP_RX_DATA_SIZE]; |
|||
|
|||
/** Data to send over USB CDC are stored in this buffer */ |
|||
uint8_t UserTxBufferFS[APP_TX_DATA_SIZE]; |
|||
|
|||
/* USER CODE BEGIN PRIVATE_VARIABLES */ |
|||
|
|||
/* USER CODE END PRIVATE_VARIABLES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Exported_Variables USBD_CDC_IF_Exported_Variables
|
|||
* @brief Public variables. |
|||
* @{ |
|||
*/ |
|||
|
|||
extern USBD_HandleTypeDef hUsbDeviceFS; |
|||
|
|||
/* USER CODE BEGIN EXPORTED_VARIABLES */ |
|||
|
|||
/* USER CODE END EXPORTED_VARIABLES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Private_FunctionPrototypes USBD_CDC_IF_Private_FunctionPrototypes
|
|||
* @brief Private functions declaration. |
|||
* @{ |
|||
*/ |
|||
|
|||
static int8_t CDC_Init_FS(void); |
|||
static int8_t CDC_DeInit_FS(void); |
|||
static int8_t CDC_Control_FS(uint8_t cmd, uint8_t* pbuf, uint16_t length); |
|||
static int8_t CDC_Receive_FS(uint8_t* pbuf, uint32_t *Len); |
|||
static int8_t CDC_TransmitCplt_FS(uint8_t *pbuf, uint32_t *Len, uint8_t epnum); |
|||
|
|||
/* USER CODE BEGIN PRIVATE_FUNCTIONS_DECLARATION */ |
|||
|
|||
/* USER CODE END PRIVATE_FUNCTIONS_DECLARATION */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
USBD_CDC_ItfTypeDef USBD_Interface_fops_FS = |
|||
{ |
|||
CDC_Init_FS, |
|||
CDC_DeInit_FS, |
|||
CDC_Control_FS, |
|||
CDC_Receive_FS, |
|||
CDC_TransmitCplt_FS |
|||
}; |
|||
|
|||
/* Private functions ---------------------------------------------------------*/ |
|||
/**
|
|||
* @brief Initializes the CDC media low layer over the FS USB IP |
|||
* @retval USBD_OK if all operations are OK else USBD_FAIL |
|||
*/ |
|||
static int8_t CDC_Init_FS(void) |
|||
{ |
|||
/* USER CODE BEGIN 3 */ |
|||
/* Set Application Buffers */ |
|||
USBD_CDC_SetTxBuffer(&hUsbDeviceFS, UserTxBufferFS, 0); |
|||
USBD_CDC_SetRxBuffer(&hUsbDeviceFS, UserRxBufferFS); |
|||
return (USBD_OK); |
|||
/* USER CODE END 3 */ |
|||
} |
|||
|
|||
/**
|
|||
* @brief DeInitializes the CDC media low layer |
|||
* @retval USBD_OK if all operations are OK else USBD_FAIL |
|||
*/ |
|||
static int8_t CDC_DeInit_FS(void) |
|||
{ |
|||
/* USER CODE BEGIN 4 */ |
|||
return (USBD_OK); |
|||
/* USER CODE END 4 */ |
|||
} |
|||
|
|||
/**
|
|||
* @brief Manage the CDC class requests |
|||
* @param cmd: Command code |
|||
* @param pbuf: Buffer containing command data (request parameters) |
|||
* @param length: Number of data to be sent (in bytes) |
|||
* @retval Result of the operation: USBD_OK if all operations are OK else USBD_FAIL |
|||
*/ |
|||
static int8_t CDC_Control_FS(uint8_t cmd, uint8_t* pbuf, uint16_t length) |
|||
{ |
|||
/* USER CODE BEGIN 5 */ |
|||
switch(cmd) |
|||
{ |
|||
case CDC_SEND_ENCAPSULATED_COMMAND: |
|||
|
|||
break; |
|||
|
|||
case CDC_GET_ENCAPSULATED_RESPONSE: |
|||
|
|||
break; |
|||
|
|||
case CDC_SET_COMM_FEATURE: |
|||
|
|||
break; |
|||
|
|||
case CDC_GET_COMM_FEATURE: |
|||
|
|||
break; |
|||
|
|||
case CDC_CLEAR_COMM_FEATURE: |
|||
|
|||
break; |
|||
|
|||
/*******************************************************************************/ |
|||
/* Line Coding Structure */ |
|||
/*-----------------------------------------------------------------------------*/ |
|||
/* Offset | Field | Size | Value | Description */ |
|||
/* 0 | dwDTERate | 4 | Number |Data terminal rate, in bits per second*/ |
|||
/* 4 | bCharFormat | 1 | Number | Stop bits */ |
|||
/* 0 - 1 Stop bit */ |
|||
/* 1 - 1.5 Stop bits */ |
|||
/* 2 - 2 Stop bits */ |
|||
/* 5 | bParityType | 1 | Number | Parity */ |
|||
/* 0 - None */ |
|||
/* 1 - Odd */ |
|||
/* 2 - Even */ |
|||
/* 3 - Mark */ |
|||
/* 4 - Space */ |
|||
/* 6 | bDataBits | 1 | Number Data bits (5, 6, 7, 8 or 16). */ |
|||
/*******************************************************************************/ |
|||
case CDC_SET_LINE_CODING: |
|||
|
|||
break; |
|||
|
|||
case CDC_GET_LINE_CODING: |
|||
|
|||
break; |
|||
|
|||
case CDC_SET_CONTROL_LINE_STATE: |
|||
|
|||
break; |
|||
|
|||
case CDC_SEND_BREAK: |
|||
|
|||
break; |
|||
|
|||
default: |
|||
break; |
|||
} |
|||
|
|||
return (USBD_OK); |
|||
/* USER CODE END 5 */ |
|||
} |
|||
|
|||
/**
|
|||
* @brief Data received over USB OUT endpoint are sent over CDC interface |
|||
* through this function. |
|||
* |
|||
* @note |
|||
* This function will issue a NAK packet on any OUT packet received on |
|||
* USB endpoint until exiting this function. If you exit this function |
|||
* before transfer is complete on CDC interface (ie. using DMA controller) |
|||
* it will result in receiving more data while previous ones are still |
|||
* not sent. |
|||
* |
|||
* @param Buf: Buffer of data to be received |
|||
* @param Len: Number of data received (in bytes) |
|||
* @retval Result of the operation: USBD_OK if all operations are OK else USBD_FAIL |
|||
*/ |
|||
static int8_t CDC_Receive_FS(uint8_t* Buf, uint32_t *Len) |
|||
{ |
|||
/* USER CODE BEGIN 6 */ |
|||
USBD_CDC_SetRxBuffer(&hUsbDeviceFS, &Buf[0]); |
|||
USBD_CDC_ReceivePacket(&hUsbDeviceFS); |
|||
return (USBD_OK); |
|||
/* USER CODE END 6 */ |
|||
} |
|||
|
|||
/**
|
|||
* @brief CDC_Transmit_FS |
|||
* Data to send over USB IN endpoint are sent over CDC interface |
|||
* through this function. |
|||
* @note |
|||
* |
|||
* |
|||
* @param Buf: Buffer of data to be sent |
|||
* @param Len: Number of data to be sent (in bytes) |
|||
* @retval USBD_OK if all operations are OK else USBD_FAIL or USBD_BUSY |
|||
*/ |
|||
uint8_t CDC_Transmit_FS(uint8_t* Buf, uint16_t Len) |
|||
{ |
|||
uint8_t result = USBD_OK; |
|||
/* USER CODE BEGIN 7 */ |
|||
USBD_CDC_HandleTypeDef *hcdc = (USBD_CDC_HandleTypeDef*)hUsbDeviceFS.pClassData; |
|||
if (hcdc->TxState != 0){ |
|||
return USBD_BUSY; |
|||
} |
|||
USBD_CDC_SetTxBuffer(&hUsbDeviceFS, Buf, Len); |
|||
result = USBD_CDC_TransmitPacket(&hUsbDeviceFS); |
|||
/* USER CODE END 7 */ |
|||
return result; |
|||
} |
|||
|
|||
/**
|
|||
* @brief CDC_TransmitCplt_FS |
|||
* Data transmitted callback |
|||
* |
|||
* @note |
|||
* This function is IN transfer complete callback used to inform user that |
|||
* the submitted Data is successfully sent over USB. |
|||
* |
|||
* @param Buf: Buffer of data to be received |
|||
* @param Len: Number of data received (in bytes) |
|||
* @retval Result of the operation: USBD_OK if all operations are OK else USBD_FAIL |
|||
*/ |
|||
static int8_t CDC_TransmitCplt_FS(uint8_t *Buf, uint32_t *Len, uint8_t epnum) |
|||
{ |
|||
uint8_t result = USBD_OK; |
|||
/* USER CODE BEGIN 13 */ |
|||
UNUSED(Buf); |
|||
UNUSED(Len); |
|||
UNUSED(epnum); |
|||
/* USER CODE END 13 */ |
|||
return result; |
|||
} |
|||
|
|||
/* USER CODE BEGIN PRIVATE_FUNCTIONS_IMPLEMENTATION */ |
|||
|
|||
/* USER CODE END PRIVATE_FUNCTIONS_IMPLEMENTATION */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
@ -0,0 +1,131 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file : usbd_cdc_if.h |
|||
* @version : v1.0_Cube |
|||
* @brief : Header for usbd_cdc_if.c file. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __USBD_CDC_IF_H__ |
|||
#define __USBD_CDC_IF_H__ |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_cdc.h" |
|||
|
|||
/* USER CODE BEGIN INCLUDE */ |
|||
|
|||
/* USER CODE END INCLUDE */ |
|||
|
|||
/** @addtogroup STM32_USB_OTG_DEVICE_LIBRARY
|
|||
* @brief For Usb device. |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF USBD_CDC_IF
|
|||
* @brief Usb VCP device module |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Exported_Defines USBD_CDC_IF_Exported_Defines
|
|||
* @brief Defines. |
|||
* @{ |
|||
*/ |
|||
/* Define size for the receive and transmit buffer over CDC */ |
|||
#define APP_RX_DATA_SIZE 2048 |
|||
#define APP_TX_DATA_SIZE 2048 |
|||
/* USER CODE BEGIN EXPORTED_DEFINES */ |
|||
|
|||
/* USER CODE END EXPORTED_DEFINES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Exported_Types USBD_CDC_IF_Exported_Types
|
|||
* @brief Types. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN EXPORTED_TYPES */ |
|||
|
|||
/* USER CODE END EXPORTED_TYPES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Exported_Macros USBD_CDC_IF_Exported_Macros
|
|||
* @brief Aliases. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN EXPORTED_MACRO */ |
|||
|
|||
/* USER CODE END EXPORTED_MACRO */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Exported_Variables USBD_CDC_IF_Exported_Variables
|
|||
* @brief Public variables. |
|||
* @{ |
|||
*/ |
|||
|
|||
/** CDC Interface callback. */ |
|||
extern USBD_CDC_ItfTypeDef USBD_Interface_fops_FS; |
|||
|
|||
/* USER CODE BEGIN EXPORTED_VARIABLES */ |
|||
|
|||
/* USER CODE END EXPORTED_VARIABLES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CDC_IF_Exported_FunctionsPrototype USBD_CDC_IF_Exported_FunctionsPrototype
|
|||
* @brief Public functions declaration. |
|||
* @{ |
|||
*/ |
|||
|
|||
uint8_t CDC_Transmit_FS(uint8_t* Buf, uint16_t Len); |
|||
|
|||
/* USER CODE BEGIN EXPORTED_FUNCTIONS */ |
|||
|
|||
/* USER CODE END EXPORTED_FUNCTIONS */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* __USBD_CDC_IF_H__ */ |
|||
|
@ -0,0 +1,445 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file : App/usbd_desc.c |
|||
* @version : v1.0_Cube |
|||
* @brief : This file implements the USB device descriptors. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_core.h" |
|||
#include "usbd_desc.h" |
|||
#include "usbd_conf.h" |
|||
|
|||
/* USER CODE BEGIN INCLUDE */ |
|||
|
|||
/* USER CODE END INCLUDE */ |
|||
|
|||
/* Private typedef -----------------------------------------------------------*/ |
|||
/* Private define ------------------------------------------------------------*/ |
|||
/* Private macro -------------------------------------------------------------*/ |
|||
|
|||
/* USER CODE BEGIN PV */ |
|||
/* Private variables ---------------------------------------------------------*/ |
|||
|
|||
/* USER CODE END PV */ |
|||
|
|||
/** @addtogroup STM32_USB_OTG_DEVICE_LIBRARY
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @addtogroup USBD_DESC
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Private_TypesDefinitions USBD_DESC_Private_TypesDefinitions
|
|||
* @brief Private types. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN PRIVATE_TYPES */ |
|||
|
|||
/* USER CODE END PRIVATE_TYPES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Private_Defines USBD_DESC_Private_Defines
|
|||
* @brief Private defines. |
|||
* @{ |
|||
*/ |
|||
|
|||
#define USBD_VID 1155 |
|||
#define USBD_LANGID_STRING 1033 |
|||
#define USBD_MANUFACTURER_STRING "Heck" |
|||
#define USBD_PID_FS 22336 |
|||
#define USBD_PRODUCT_STRING_FS "STM32 HeckPort" |
|||
#define USBD_CONFIGURATION_STRING_FS "CDC Config" |
|||
#define USBD_INTERFACE_STRING_FS "CDC Interface" |
|||
|
|||
#define USB_SIZ_BOS_DESC 0x0C |
|||
|
|||
/* USER CODE BEGIN PRIVATE_DEFINES */ |
|||
|
|||
/* USER CODE END PRIVATE_DEFINES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN 0 */ |
|||
|
|||
/* USER CODE END 0 */ |
|||
|
|||
/** @defgroup USBD_DESC_Private_Macros USBD_DESC_Private_Macros
|
|||
* @brief Private macros. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN PRIVATE_MACRO */ |
|||
|
|||
/* USER CODE END PRIVATE_MACRO */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Private_FunctionPrototypes USBD_DESC_Private_FunctionPrototypes
|
|||
* @brief Private functions declaration. |
|||
* @{ |
|||
*/ |
|||
|
|||
static void Get_SerialNum(void); |
|||
static void IntToUnicode(uint32_t value, uint8_t * pbuf, uint8_t len); |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Private_FunctionPrototypes USBD_DESC_Private_FunctionPrototypes
|
|||
* @brief Private functions declaration for FS. |
|||
* @{ |
|||
*/ |
|||
|
|||
uint8_t * USBD_FS_DeviceDescriptor(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t * USBD_FS_LangIDStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t * USBD_FS_ManufacturerStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t * USBD_FS_ProductStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t * USBD_FS_SerialStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t * USBD_FS_ConfigStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
uint8_t * USBD_FS_InterfaceStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
#if (USBD_LPM_ENABLED == 1) |
|||
uint8_t * USBD_FS_USR_BOSDescriptor(USBD_SpeedTypeDef speed, uint16_t *length); |
|||
#endif /* (USBD_LPM_ENABLED == 1) */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Private_Variables USBD_DESC_Private_Variables
|
|||
* @brief Private variables. |
|||
* @{ |
|||
*/ |
|||
|
|||
USBD_DescriptorsTypeDef FS_Desc = |
|||
{ |
|||
USBD_FS_DeviceDescriptor |
|||
, USBD_FS_LangIDStrDescriptor |
|||
, USBD_FS_ManufacturerStrDescriptor |
|||
, USBD_FS_ProductStrDescriptor |
|||
, USBD_FS_SerialStrDescriptor |
|||
, USBD_FS_ConfigStrDescriptor |
|||
, USBD_FS_InterfaceStrDescriptor |
|||
#if (USBD_LPM_ENABLED == 1) |
|||
, USBD_FS_USR_BOSDescriptor |
|||
#endif /* (USBD_LPM_ENABLED == 1) */ |
|||
}; |
|||
|
|||
#if defined ( __ICCARM__ ) /* IAR Compiler */ |
|||
#pragma data_alignment=4 |
|||
#endif /* defined ( __ICCARM__ ) */ |
|||
/** USB standard device descriptor. */ |
|||
__ALIGN_BEGIN uint8_t USBD_FS_DeviceDesc[USB_LEN_DEV_DESC] __ALIGN_END = |
|||
{ |
|||
0x12, /*bLength */ |
|||
USB_DESC_TYPE_DEVICE, /*bDescriptorType*/ |
|||
#if (USBD_LPM_ENABLED == 1) |
|||
0x01, /*bcdUSB */ /* changed to USB version 2.01
|
|||
in order to support LPM L1 suspend |
|||
resume test of USBCV3.0*/ |
|||
#else |
|||
0x00, /*bcdUSB */ |
|||
#endif /* (USBD_LPM_ENABLED == 1) */ |
|||
0x02, |
|||
0x02, /*bDeviceClass*/ |
|||
0x02, /*bDeviceSubClass*/ |
|||
0x00, /*bDeviceProtocol*/ |
|||
USB_MAX_EP0_SIZE, /*bMaxPacketSize*/ |
|||
LOBYTE(USBD_VID), /*idVendor*/ |
|||
HIBYTE(USBD_VID), /*idVendor*/ |
|||
LOBYTE(USBD_PID_FS), /*idProduct*/ |
|||
HIBYTE(USBD_PID_FS), /*idProduct*/ |
|||
0x00, /*bcdDevice rel. 2.00*/ |
|||
0x02, |
|||
USBD_IDX_MFC_STR, /*Index of manufacturer string*/ |
|||
USBD_IDX_PRODUCT_STR, /*Index of product string*/ |
|||
USBD_IDX_SERIAL_STR, /*Index of serial number string*/ |
|||
USBD_MAX_NUM_CONFIGURATION /*bNumConfigurations*/ |
|||
}; |
|||
|
|||
/* USB_DeviceDescriptor */ |
|||
/** BOS descriptor. */ |
|||
#if (USBD_LPM_ENABLED == 1) |
|||
#if defined ( __ICCARM__ ) /* IAR Compiler */ |
|||
#pragma data_alignment=4 |
|||
#endif /* defined ( __ICCARM__ ) */ |
|||
__ALIGN_BEGIN uint8_t USBD_FS_BOSDesc[USB_SIZ_BOS_DESC] __ALIGN_END = |
|||
{ |
|||
0x5, |
|||
USB_DESC_TYPE_BOS, |
|||
0xC, |
|||
0x0, |
|||
0x1, /* 1 device capability*/ |
|||
/* device capability*/ |
|||
0x7, |
|||
USB_DEVICE_CAPABITY_TYPE, |
|||
0x2, |
|||
0x2, /* LPM capability bit set*/ |
|||
0x0, |
|||
0x0, |
|||
0x0 |
|||
}; |
|||
#endif /* (USBD_LPM_ENABLED == 1) */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Private_Variables USBD_DESC_Private_Variables
|
|||
* @brief Private variables. |
|||
* @{ |
|||
*/ |
|||
|
|||
#if defined ( __ICCARM__ ) /* IAR Compiler */ |
|||
#pragma data_alignment=4 |
|||
#endif /* defined ( __ICCARM__ ) */ |
|||
|
|||
/** USB lang identifier descriptor. */ |
|||
__ALIGN_BEGIN uint8_t USBD_LangIDDesc[USB_LEN_LANGID_STR_DESC] __ALIGN_END = |
|||
{ |
|||
USB_LEN_LANGID_STR_DESC, |
|||
USB_DESC_TYPE_STRING, |
|||
LOBYTE(USBD_LANGID_STRING), |
|||
HIBYTE(USBD_LANGID_STRING) |
|||
}; |
|||
|
|||
#if defined ( __ICCARM__ ) /* IAR Compiler */ |
|||
#pragma data_alignment=4 |
|||
#endif /* defined ( __ICCARM__ ) */ |
|||
/* Internal string descriptor. */ |
|||
__ALIGN_BEGIN uint8_t USBD_StrDesc[USBD_MAX_STR_DESC_SIZ] __ALIGN_END; |
|||
|
|||
#if defined ( __ICCARM__ ) /*!< IAR Compiler */ |
|||
#pragma data_alignment=4 |
|||
#endif |
|||
__ALIGN_BEGIN uint8_t USBD_StringSerial[USB_SIZ_STRING_SERIAL] __ALIGN_END = { |
|||
USB_SIZ_STRING_SERIAL, |
|||
USB_DESC_TYPE_STRING, |
|||
}; |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Private_Functions USBD_DESC_Private_Functions
|
|||
* @brief Private functions. |
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @brief Return the device descriptor |
|||
* @param speed : Current device speed |
|||
* @param length : Pointer to data length variable |
|||
* @retval Pointer to descriptor buffer |
|||
*/ |
|||
uint8_t * USBD_FS_DeviceDescriptor(USBD_SpeedTypeDef speed, uint16_t *length) |
|||
{ |
|||
UNUSED(speed); |
|||
*length = sizeof(USBD_FS_DeviceDesc); |
|||
return USBD_FS_DeviceDesc; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Return the LangID string descriptor |
|||
* @param speed : Current device speed |
|||
* @param length : Pointer to data length variable |
|||
* @retval Pointer to descriptor buffer |
|||
*/ |
|||
uint8_t * USBD_FS_LangIDStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length) |
|||
{ |
|||
UNUSED(speed); |
|||
*length = sizeof(USBD_LangIDDesc); |
|||
return USBD_LangIDDesc; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Return the product string descriptor |
|||
* @param speed : Current device speed |
|||
* @param length : Pointer to data length variable |
|||
* @retval Pointer to descriptor buffer |
|||
*/ |
|||
uint8_t * USBD_FS_ProductStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length) |
|||
{ |
|||
if(speed == 0) |
|||
{ |
|||
USBD_GetString((uint8_t *)USBD_PRODUCT_STRING_FS, USBD_StrDesc, length); |
|||
} |
|||
else |
|||
{ |
|||
USBD_GetString((uint8_t *)USBD_PRODUCT_STRING_FS, USBD_StrDesc, length); |
|||
} |
|||
return USBD_StrDesc; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Return the manufacturer string descriptor |
|||
* @param speed : Current device speed |
|||
* @param length : Pointer to data length variable |
|||
* @retval Pointer to descriptor buffer |
|||
*/ |
|||
uint8_t * USBD_FS_ManufacturerStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length) |
|||
{ |
|||
UNUSED(speed); |
|||
USBD_GetString((uint8_t *)USBD_MANUFACTURER_STRING, USBD_StrDesc, length); |
|||
return USBD_StrDesc; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Return the serial number string descriptor |
|||
* @param speed : Current device speed |
|||
* @param length : Pointer to data length variable |
|||
* @retval Pointer to descriptor buffer |
|||
*/ |
|||
uint8_t * USBD_FS_SerialStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length) |
|||
{ |
|||
UNUSED(speed); |
|||
*length = USB_SIZ_STRING_SERIAL; |
|||
|
|||
/* Update the serial number string descriptor with the data from the unique
|
|||
* ID */ |
|||
Get_SerialNum(); |
|||
/* USER CODE BEGIN USBD_FS_SerialStrDescriptor */ |
|||
|
|||
/* USER CODE END USBD_FS_SerialStrDescriptor */ |
|||
return (uint8_t *) USBD_StringSerial; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Return the configuration string descriptor |
|||
* @param speed : Current device speed |
|||
* @param length : Pointer to data length variable |
|||
* @retval Pointer to descriptor buffer |
|||
*/ |
|||
uint8_t * USBD_FS_ConfigStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length) |
|||
{ |
|||
if(speed == USBD_SPEED_HIGH) |
|||
{ |
|||
USBD_GetString((uint8_t *)USBD_CONFIGURATION_STRING_FS, USBD_StrDesc, length); |
|||
} |
|||
else |
|||
{ |
|||
USBD_GetString((uint8_t *)USBD_CONFIGURATION_STRING_FS, USBD_StrDesc, length); |
|||
} |
|||
return USBD_StrDesc; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Return the interface string descriptor |
|||
* @param speed : Current device speed |
|||
* @param length : Pointer to data length variable |
|||
* @retval Pointer to descriptor buffer |
|||
*/ |
|||
uint8_t * USBD_FS_InterfaceStrDescriptor(USBD_SpeedTypeDef speed, uint16_t *length) |
|||
{ |
|||
if(speed == 0) |
|||
{ |
|||
USBD_GetString((uint8_t *)USBD_INTERFACE_STRING_FS, USBD_StrDesc, length); |
|||
} |
|||
else |
|||
{ |
|||
USBD_GetString((uint8_t *)USBD_INTERFACE_STRING_FS, USBD_StrDesc, length); |
|||
} |
|||
return USBD_StrDesc; |
|||
} |
|||
|
|||
#if (USBD_LPM_ENABLED == 1) |
|||
/**
|
|||
* @brief Return the BOS descriptor |
|||
* @param speed : Current device speed |
|||
* @param length : Pointer to data length variable |
|||
* @retval Pointer to descriptor buffer |
|||
*/ |
|||
uint8_t * USBD_FS_USR_BOSDescriptor(USBD_SpeedTypeDef speed, uint16_t *length) |
|||
{ |
|||
UNUSED(speed); |
|||
*length = sizeof(USBD_FS_BOSDesc); |
|||
return (uint8_t*)USBD_FS_BOSDesc; |
|||
} |
|||
#endif /* (USBD_LPM_ENABLED == 1) */ |
|||
|
|||
/**
|
|||
* @brief Create the serial number string descriptor |
|||
* @param None |
|||
* @retval None |
|||
*/ |
|||
static void Get_SerialNum(void) |
|||
{ |
|||
uint32_t deviceserial0; |
|||
uint32_t deviceserial1; |
|||
uint32_t deviceserial2; |
|||
|
|||
deviceserial0 = *(uint32_t *) DEVICE_ID1; |
|||
deviceserial1 = *(uint32_t *) DEVICE_ID2; |
|||
deviceserial2 = *(uint32_t *) DEVICE_ID3; |
|||
|
|||
deviceserial0 += deviceserial2; |
|||
|
|||
if (deviceserial0 != 0) |
|||
{ |
|||
IntToUnicode(deviceserial0, &USBD_StringSerial[2], 8); |
|||
IntToUnicode(deviceserial1, &USBD_StringSerial[18], 4); |
|||
} |
|||
} |
|||
|
|||
/**
|
|||
* @brief Convert Hex 32Bits value into char |
|||
* @param value: value to convert |
|||
* @param pbuf: pointer to the buffer |
|||
* @param len: buffer length |
|||
* @retval None |
|||
*/ |
|||
static void IntToUnicode(uint32_t value, uint8_t * pbuf, uint8_t len) |
|||
{ |
|||
uint8_t idx = 0; |
|||
|
|||
for (idx = 0; idx < len; idx++) |
|||
{ |
|||
if (((value >> 28)) < 0xA) |
|||
{ |
|||
pbuf[2 * idx] = (value >> 28) + '0'; |
|||
} |
|||
else |
|||
{ |
|||
pbuf[2 * idx] = (value >> 28) + 'A' - 10; |
|||
} |
|||
|
|||
value = value << 4; |
|||
|
|||
pbuf[2 * idx + 1] = 0; |
|||
} |
|||
} |
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
@ -0,0 +1,143 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file : usbd_desc.c |
|||
* @version : v1.0_Cube |
|||
* @brief : Header for usbd_conf.c file. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __USBD_DESC__C__ |
|||
#define __USBD_DESC__C__ |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "usbd_def.h" |
|||
|
|||
/* USER CODE BEGIN INCLUDE */ |
|||
|
|||
/* USER CODE END INCLUDE */ |
|||
|
|||
/** @addtogroup STM32_USB_OTG_DEVICE_LIBRARY
|
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC USBD_DESC
|
|||
* @brief Usb device descriptors module. |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Exported_Constants USBD_DESC_Exported_Constants
|
|||
* @brief Constants. |
|||
* @{ |
|||
*/ |
|||
#define DEVICE_ID1 (UID_BASE) |
|||
#define DEVICE_ID2 (UID_BASE + 0x4) |
|||
#define DEVICE_ID3 (UID_BASE + 0x8) |
|||
|
|||
#define USB_SIZ_STRING_SERIAL 0x1A |
|||
|
|||
/* USER CODE BEGIN EXPORTED_CONSTANTS */ |
|||
|
|||
/* USER CODE END EXPORTED_CONSTANTS */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Exported_Defines USBD_DESC_Exported_Defines
|
|||
* @brief Defines. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN EXPORTED_DEFINES */ |
|||
|
|||
/* USER CODE END EXPORTED_DEFINES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Exported_TypesDefinitions USBD_DESC_Exported_TypesDefinitions
|
|||
* @brief Types. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN EXPORTED_TYPES */ |
|||
|
|||
/* USER CODE END EXPORTED_TYPES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Exported_Macros USBD_DESC_Exported_Macros
|
|||
* @brief Aliases. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN EXPORTED_MACRO */ |
|||
|
|||
/* USER CODE END EXPORTED_MACRO */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Exported_Variables USBD_DESC_Exported_Variables
|
|||
* @brief Public variables. |
|||
* @{ |
|||
*/ |
|||
|
|||
/** Descriptor for the Usb device. */ |
|||
extern USBD_DescriptorsTypeDef FS_Desc; |
|||
|
|||
/* USER CODE BEGIN EXPORTED_VARIABLES */ |
|||
|
|||
/* USER CODE END EXPORTED_VARIABLES */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_DESC_Exported_FunctionsPrototype USBD_DESC_Exported_FunctionsPrototype
|
|||
* @brief Public functions declaration. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* USER CODE BEGIN EXPORTED_FUNCTIONS */ |
|||
|
|||
/* USER CODE END EXPORTED_FUNCTIONS */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* __USBD_DESC__C__ */ |
|||
|
@ -0,0 +1,679 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file : Target/usbd_conf.c |
|||
* @version : v1.0_Cube |
|||
* @brief : This file implements the board support package for the USB device library |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include "stm32f4xx.h" |
|||
#include "stm32f4xx_hal.h" |
|||
#include "usbd_def.h" |
|||
#include "usbd_core.h" |
|||
|
|||
#include "usbd_cdc.h" |
|||
|
|||
/* USER CODE BEGIN Includes */ |
|||
|
|||
/* USER CODE END Includes */ |
|||
|
|||
/* Private typedef -----------------------------------------------------------*/ |
|||
/* Private define ------------------------------------------------------------*/ |
|||
/* Private macro -------------------------------------------------------------*/ |
|||
|
|||
/* USER CODE BEGIN PV */ |
|||
/* Private variables ---------------------------------------------------------*/ |
|||
|
|||
/* USER CODE END PV */ |
|||
|
|||
PCD_HandleTypeDef hpcd_USB_OTG_FS; |
|||
void Error_Handler(void); |
|||
|
|||
/* External functions --------------------------------------------------------*/ |
|||
void SystemClock_Config(void); |
|||
|
|||
/* USER CODE BEGIN 0 */ |
|||
|
|||
/* USER CODE END 0 */ |
|||
|
|||
/* USER CODE BEGIN PFP */ |
|||
/* Private function prototypes -----------------------------------------------*/ |
|||
USBD_StatusTypeDef USBD_Get_USB_Status(HAL_StatusTypeDef hal_status); |
|||
|
|||
/* USER CODE END PFP */ |
|||
|
|||
/* Private functions ---------------------------------------------------------*/ |
|||
|
|||
/* USER CODE BEGIN 1 */ |
|||
|
|||
/* USER CODE END 1 */ |
|||
|
|||
/*******************************************************************************
|
|||
LL Driver Callbacks (PCD -> USB Device Library) |
|||
*******************************************************************************/ |
|||
/* MSP Init */ |
|||
|
|||
void HAL_PCD_MspInit(PCD_HandleTypeDef* pcdHandle) |
|||
{ |
|||
GPIO_InitTypeDef GPIO_InitStruct = {0}; |
|||
if(pcdHandle->Instance==USB_OTG_FS) |
|||
{ |
|||
/* USER CODE BEGIN USB_OTG_FS_MspInit 0 */ |
|||
|
|||
/* USER CODE END USB_OTG_FS_MspInit 0 */ |
|||
|
|||
__HAL_RCC_GPIOA_CLK_ENABLE(); |
|||
/**USB_OTG_FS GPIO Configuration
|
|||
PA11 ------> USB_OTG_FS_DM |
|||
PA12 ------> USB_OTG_FS_DP |
|||
*/ |
|||
GPIO_InitStruct.Pin = GPIO_PIN_11|GPIO_PIN_12; |
|||
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; |
|||
GPIO_InitStruct.Pull = GPIO_NOPULL; |
|||
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; |
|||
GPIO_InitStruct.Alternate = GPIO_AF10_OTG_FS; |
|||
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct); |
|||
|
|||
/* Peripheral clock enable */ |
|||
__HAL_RCC_USB_OTG_FS_CLK_ENABLE(); |
|||
|
|||
/* Peripheral interrupt init */ |
|||
HAL_NVIC_SetPriority(OTG_FS_IRQn, 0, 0); |
|||
HAL_NVIC_EnableIRQ(OTG_FS_IRQn); |
|||
/* USER CODE BEGIN USB_OTG_FS_MspInit 1 */ |
|||
|
|||
/* USER CODE END USB_OTG_FS_MspInit 1 */ |
|||
} |
|||
} |
|||
|
|||
void HAL_PCD_MspDeInit(PCD_HandleTypeDef* pcdHandle) |
|||
{ |
|||
if(pcdHandle->Instance==USB_OTG_FS) |
|||
{ |
|||
/* USER CODE BEGIN USB_OTG_FS_MspDeInit 0 */ |
|||
|
|||
/* USER CODE END USB_OTG_FS_MspDeInit 0 */ |
|||
/* Peripheral clock disable */ |
|||
__HAL_RCC_USB_OTG_FS_CLK_DISABLE(); |
|||
|
|||
/**USB_OTG_FS GPIO Configuration
|
|||
PA11 ------> USB_OTG_FS_DM |
|||
PA12 ------> USB_OTG_FS_DP |
|||
*/ |
|||
HAL_GPIO_DeInit(GPIOA, GPIO_PIN_11|GPIO_PIN_12); |
|||
|
|||
/* Peripheral interrupt Deinit*/ |
|||
HAL_NVIC_DisableIRQ(OTG_FS_IRQn); |
|||
|
|||
/* USER CODE BEGIN USB_OTG_FS_MspDeInit 1 */ |
|||
|
|||
/* USER CODE END USB_OTG_FS_MspDeInit 1 */ |
|||
} |
|||
} |
|||
|
|||
/**
|
|||
* @brief Setup stage callback |
|||
* @param hpcd: PCD handle |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_SetupStageCallback(PCD_HandleTypeDef *hpcd) |
|||
#else |
|||
void HAL_PCD_SetupStageCallback(PCD_HandleTypeDef *hpcd) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
USBD_LL_SetupStage((USBD_HandleTypeDef*)hpcd->pData, (uint8_t *)hpcd->Setup); |
|||
} |
|||
|
|||
/**
|
|||
* @brief Data Out stage callback. |
|||
* @param hpcd: PCD handle |
|||
* @param epnum: Endpoint number |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_DataOutStageCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum) |
|||
#else |
|||
void HAL_PCD_DataOutStageCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
USBD_LL_DataOutStage((USBD_HandleTypeDef*)hpcd->pData, epnum, hpcd->OUT_ep[epnum].xfer_buff); |
|||
} |
|||
|
|||
/**
|
|||
* @brief Data In stage callback. |
|||
* @param hpcd: PCD handle |
|||
* @param epnum: Endpoint number |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_DataInStageCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum) |
|||
#else |
|||
void HAL_PCD_DataInStageCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
USBD_LL_DataInStage((USBD_HandleTypeDef*)hpcd->pData, epnum, hpcd->IN_ep[epnum].xfer_buff); |
|||
} |
|||
|
|||
/**
|
|||
* @brief SOF callback. |
|||
* @param hpcd: PCD handle |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_SOFCallback(PCD_HandleTypeDef *hpcd) |
|||
#else |
|||
void HAL_PCD_SOFCallback(PCD_HandleTypeDef *hpcd) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
USBD_LL_SOF((USBD_HandleTypeDef*)hpcd->pData); |
|||
} |
|||
|
|||
/**
|
|||
* @brief Reset callback. |
|||
* @param hpcd: PCD handle |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_ResetCallback(PCD_HandleTypeDef *hpcd) |
|||
#else |
|||
void HAL_PCD_ResetCallback(PCD_HandleTypeDef *hpcd) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
USBD_SpeedTypeDef speed = USBD_SPEED_FULL; |
|||
|
|||
if ( hpcd->Init.speed == PCD_SPEED_HIGH) |
|||
{ |
|||
speed = USBD_SPEED_HIGH; |
|||
} |
|||
else if ( hpcd->Init.speed == PCD_SPEED_FULL) |
|||
{ |
|||
speed = USBD_SPEED_FULL; |
|||
} |
|||
else |
|||
{ |
|||
Error_Handler(); |
|||
} |
|||
/* Set Speed. */ |
|||
USBD_LL_SetSpeed((USBD_HandleTypeDef*)hpcd->pData, speed); |
|||
|
|||
/* Reset Device. */ |
|||
USBD_LL_Reset((USBD_HandleTypeDef*)hpcd->pData); |
|||
} |
|||
|
|||
/**
|
|||
* @brief Suspend callback. |
|||
* When Low power mode is enabled the debug cannot be used (IAR, Keil doesn't support it) |
|||
* @param hpcd: PCD handle |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_SuspendCallback(PCD_HandleTypeDef *hpcd) |
|||
#else |
|||
void HAL_PCD_SuspendCallback(PCD_HandleTypeDef *hpcd) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
/* Inform USB library that core enters in suspend Mode. */ |
|||
USBD_LL_Suspend((USBD_HandleTypeDef*)hpcd->pData); |
|||
__HAL_PCD_GATE_PHYCLOCK(hpcd); |
|||
/* Enter in STOP mode. */ |
|||
/* USER CODE BEGIN 2 */ |
|||
if (hpcd->Init.low_power_enable) |
|||
{ |
|||
/* Set SLEEPDEEP bit and SleepOnExit of Cortex System Control Register. */ |
|||
SCB->SCR |= (uint32_t)((uint32_t)(SCB_SCR_SLEEPDEEP_Msk | SCB_SCR_SLEEPONEXIT_Msk)); |
|||
} |
|||
/* USER CODE END 2 */ |
|||
} |
|||
|
|||
/**
|
|||
* @brief Resume callback. |
|||
* When Low power mode is enabled the debug cannot be used (IAR, Keil doesn't support it) |
|||
* @param hpcd: PCD handle |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_ResumeCallback(PCD_HandleTypeDef *hpcd) |
|||
#else |
|||
void HAL_PCD_ResumeCallback(PCD_HandleTypeDef *hpcd) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
/* USER CODE BEGIN 3 */ |
|||
|
|||
/* USER CODE END 3 */ |
|||
USBD_LL_Resume((USBD_HandleTypeDef*)hpcd->pData); |
|||
} |
|||
|
|||
/**
|
|||
* @brief ISOOUTIncomplete callback. |
|||
* @param hpcd: PCD handle |
|||
* @param epnum: Endpoint number |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_ISOOUTIncompleteCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum) |
|||
#else |
|||
void HAL_PCD_ISOOUTIncompleteCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
USBD_LL_IsoOUTIncomplete((USBD_HandleTypeDef*)hpcd->pData, epnum); |
|||
} |
|||
|
|||
/**
|
|||
* @brief ISOINIncomplete callback. |
|||
* @param hpcd: PCD handle |
|||
* @param epnum: Endpoint number |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_ISOINIncompleteCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum) |
|||
#else |
|||
void HAL_PCD_ISOINIncompleteCallback(PCD_HandleTypeDef *hpcd, uint8_t epnum) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
USBD_LL_IsoINIncomplete((USBD_HandleTypeDef*)hpcd->pData, epnum); |
|||
} |
|||
|
|||
/**
|
|||
* @brief Connect callback. |
|||
* @param hpcd: PCD handle |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_ConnectCallback(PCD_HandleTypeDef *hpcd) |
|||
#else |
|||
void HAL_PCD_ConnectCallback(PCD_HandleTypeDef *hpcd) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
USBD_LL_DevConnected((USBD_HandleTypeDef*)hpcd->pData); |
|||
} |
|||
|
|||
/**
|
|||
* @brief Disconnect callback. |
|||
* @param hpcd: PCD handle |
|||
* @retval None |
|||
*/ |
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
static void PCD_DisconnectCallback(PCD_HandleTypeDef *hpcd) |
|||
#else |
|||
void HAL_PCD_DisconnectCallback(PCD_HandleTypeDef *hpcd) |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
{ |
|||
USBD_LL_DevDisconnected((USBD_HandleTypeDef*)hpcd->pData); |
|||
} |
|||
|
|||
/*******************************************************************************
|
|||
LL Driver Interface (USB Device Library --> PCD) |
|||
*******************************************************************************/ |
|||
|
|||
/**
|
|||
* @brief Initializes the low level portion of the device driver. |
|||
* @param pdev: Device handle |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_Init(USBD_HandleTypeDef *pdev) |
|||
{ |
|||
/* Init USB Ip. */ |
|||
if (pdev->id == DEVICE_FS) { |
|||
/* Link the driver to the stack. */ |
|||
hpcd_USB_OTG_FS.pData = pdev; |
|||
pdev->pData = &hpcd_USB_OTG_FS; |
|||
|
|||
hpcd_USB_OTG_FS.Instance = USB_OTG_FS; |
|||
hpcd_USB_OTG_FS.Init.dev_endpoints = 4; |
|||
hpcd_USB_OTG_FS.Init.speed = PCD_SPEED_FULL; |
|||
hpcd_USB_OTG_FS.Init.dma_enable = DISABLE; |
|||
hpcd_USB_OTG_FS.Init.phy_itface = PCD_PHY_EMBEDDED; |
|||
hpcd_USB_OTG_FS.Init.Sof_enable = DISABLE; |
|||
hpcd_USB_OTG_FS.Init.low_power_enable = DISABLE; |
|||
hpcd_USB_OTG_FS.Init.lpm_enable = DISABLE; |
|||
hpcd_USB_OTG_FS.Init.vbus_sensing_enable = DISABLE; |
|||
hpcd_USB_OTG_FS.Init.use_dedicated_ep1 = DISABLE; |
|||
if (HAL_PCD_Init(&hpcd_USB_OTG_FS) != HAL_OK) |
|||
{ |
|||
Error_Handler( ); |
|||
} |
|||
|
|||
#if (USE_HAL_PCD_REGISTER_CALLBACKS == 1U) |
|||
/* Register USB PCD CallBacks */ |
|||
HAL_PCD_RegisterCallback(&hpcd_USB_OTG_FS, HAL_PCD_SOF_CB_ID, PCD_SOFCallback); |
|||
HAL_PCD_RegisterCallback(&hpcd_USB_OTG_FS, HAL_PCD_SETUPSTAGE_CB_ID, PCD_SetupStageCallback); |
|||
HAL_PCD_RegisterCallback(&hpcd_USB_OTG_FS, HAL_PCD_RESET_CB_ID, PCD_ResetCallback); |
|||
HAL_PCD_RegisterCallback(&hpcd_USB_OTG_FS, HAL_PCD_SUSPEND_CB_ID, PCD_SuspendCallback); |
|||
HAL_PCD_RegisterCallback(&hpcd_USB_OTG_FS, HAL_PCD_RESUME_CB_ID, PCD_ResumeCallback); |
|||
HAL_PCD_RegisterCallback(&hpcd_USB_OTG_FS, HAL_PCD_CONNECT_CB_ID, PCD_ConnectCallback); |
|||
HAL_PCD_RegisterCallback(&hpcd_USB_OTG_FS, HAL_PCD_DISCONNECT_CB_ID, PCD_DisconnectCallback); |
|||
|
|||
HAL_PCD_RegisterDataOutStageCallback(&hpcd_USB_OTG_FS, PCD_DataOutStageCallback); |
|||
HAL_PCD_RegisterDataInStageCallback(&hpcd_USB_OTG_FS, PCD_DataInStageCallback); |
|||
HAL_PCD_RegisterIsoOutIncpltCallback(&hpcd_USB_OTG_FS, PCD_ISOOUTIncompleteCallback); |
|||
HAL_PCD_RegisterIsoInIncpltCallback(&hpcd_USB_OTG_FS, PCD_ISOINIncompleteCallback); |
|||
#endif /* USE_HAL_PCD_REGISTER_CALLBACKS */ |
|||
HAL_PCDEx_SetRxFiFo(&hpcd_USB_OTG_FS, 0x80); |
|||
HAL_PCDEx_SetTxFiFo(&hpcd_USB_OTG_FS, 0, 0x40); |
|||
HAL_PCDEx_SetTxFiFo(&hpcd_USB_OTG_FS, 1, 0x80); |
|||
} |
|||
return USBD_OK; |
|||
} |
|||
|
|||
/**
|
|||
* @brief De-Initializes the low level portion of the device driver. |
|||
* @param pdev: Device handle |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_DeInit(USBD_HandleTypeDef *pdev) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_DeInit(pdev->pData); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Starts the low level portion of the device driver. |
|||
* @param pdev: Device handle |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_Start(USBD_HandleTypeDef *pdev) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_Start(pdev->pData); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Stops the low level portion of the device driver. |
|||
* @param pdev: Device handle |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_Stop(USBD_HandleTypeDef *pdev) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_Stop(pdev->pData); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Opens an endpoint of the low level driver. |
|||
* @param pdev: Device handle |
|||
* @param ep_addr: Endpoint number |
|||
* @param ep_type: Endpoint type |
|||
* @param ep_mps: Endpoint max packet size |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_OpenEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr, uint8_t ep_type, uint16_t ep_mps) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_EP_Open(pdev->pData, ep_addr, ep_mps, ep_type); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Closes an endpoint of the low level driver. |
|||
* @param pdev: Device handle |
|||
* @param ep_addr: Endpoint number |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_CloseEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_EP_Close(pdev->pData, ep_addr); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Flushes an endpoint of the Low Level Driver. |
|||
* @param pdev: Device handle |
|||
* @param ep_addr: Endpoint number |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_FlushEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_EP_Flush(pdev->pData, ep_addr); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Sets a Stall condition on an endpoint of the Low Level Driver. |
|||
* @param pdev: Device handle |
|||
* @param ep_addr: Endpoint number |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_StallEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_EP_SetStall(pdev->pData, ep_addr); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Clears a Stall condition on an endpoint of the Low Level Driver. |
|||
* @param pdev: Device handle |
|||
* @param ep_addr: Endpoint number |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_ClearStallEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_EP_ClrStall(pdev->pData, ep_addr); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Returns Stall condition. |
|||
* @param pdev: Device handle |
|||
* @param ep_addr: Endpoint number |
|||
* @retval Stall (1: Yes, 0: No) |
|||
*/ |
|||
uint8_t USBD_LL_IsStallEP(USBD_HandleTypeDef *pdev, uint8_t ep_addr) |
|||
{ |
|||
PCD_HandleTypeDef *hpcd = (PCD_HandleTypeDef*) pdev->pData; |
|||
|
|||
if((ep_addr & 0x80) == 0x80) |
|||
{ |
|||
return hpcd->IN_ep[ep_addr & 0x7F].is_stall; |
|||
} |
|||
else |
|||
{ |
|||
return hpcd->OUT_ep[ep_addr & 0x7F].is_stall; |
|||
} |
|||
} |
|||
|
|||
/**
|
|||
* @brief Assigns a USB address to the device. |
|||
* @param pdev: Device handle |
|||
* @param dev_addr: Device address |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_SetUSBAddress(USBD_HandleTypeDef *pdev, uint8_t dev_addr) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_SetAddress(pdev->pData, dev_addr); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Transmits data over an endpoint. |
|||
* @param pdev: Device handle |
|||
* @param ep_addr: Endpoint number |
|||
* @param pbuf: Pointer to data to be sent |
|||
* @param size: Data size |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_Transmit(USBD_HandleTypeDef *pdev, uint8_t ep_addr, uint8_t *pbuf, uint32_t size) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_EP_Transmit(pdev->pData, ep_addr, pbuf, size); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Prepares an endpoint for reception. |
|||
* @param pdev: Device handle |
|||
* @param ep_addr: Endpoint number |
|||
* @param pbuf: Pointer to data to be received |
|||
* @param size: Data size |
|||
* @retval USBD status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_PrepareReceive(USBD_HandleTypeDef *pdev, uint8_t ep_addr, uint8_t *pbuf, uint32_t size) |
|||
{ |
|||
HAL_StatusTypeDef hal_status = HAL_OK; |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
hal_status = HAL_PCD_EP_Receive(pdev->pData, ep_addr, pbuf, size); |
|||
|
|||
usb_status = USBD_Get_USB_Status(hal_status); |
|||
|
|||
return usb_status; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Returns the last transferred packet size. |
|||
* @param pdev: Device handle |
|||
* @param ep_addr: Endpoint number |
|||
* @retval Received Data Size |
|||
*/ |
|||
uint32_t USBD_LL_GetRxDataSize(USBD_HandleTypeDef *pdev, uint8_t ep_addr) |
|||
{ |
|||
return HAL_PCD_EP_GetRxCount((PCD_HandleTypeDef*) pdev->pData, ep_addr); |
|||
} |
|||
|
|||
#ifdef USBD_HS_TESTMODE_ENABLE |
|||
/**
|
|||
* @brief Set High speed Test mode. |
|||
* @param pdev: Device handle |
|||
* @param testmode: test mode |
|||
* @retval USBD Status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_LL_SetTestMode(USBD_HandleTypeDef *pdev, uint8_t testmode) |
|||
{ |
|||
UNUSED(pdev); |
|||
UNUSED(testmode); |
|||
|
|||
return USBD_OK; |
|||
} |
|||
#endif /* USBD_HS_TESTMODE_ENABLE */ |
|||
|
|||
/**
|
|||
* @brief Static single allocation. |
|||
* @param size: Size of allocated memory |
|||
* @retval None |
|||
*/ |
|||
void *USBD_static_malloc(uint32_t size) |
|||
{ |
|||
static uint32_t mem[(sizeof(USBD_CDC_HandleTypeDef)/4)+1];/* On 32-bit boundary */ |
|||
return mem; |
|||
} |
|||
|
|||
/**
|
|||
* @brief Dummy memory free |
|||
* @param p: Pointer to allocated memory address |
|||
* @retval None |
|||
*/ |
|||
void USBD_static_free(void *p) |
|||
{ |
|||
|
|||
} |
|||
|
|||
/**
|
|||
* @brief Delays routine for the USB Device Library. |
|||
* @param Delay: Delay in ms |
|||
* @retval None |
|||
*/ |
|||
void USBD_LL_Delay(uint32_t Delay) |
|||
{ |
|||
HAL_Delay(Delay); |
|||
} |
|||
|
|||
/**
|
|||
* @brief Returns the USB status depending on the HAL status: |
|||
* @param hal_status: HAL status |
|||
* @retval USB status |
|||
*/ |
|||
USBD_StatusTypeDef USBD_Get_USB_Status(HAL_StatusTypeDef hal_status) |
|||
{ |
|||
USBD_StatusTypeDef usb_status = USBD_OK; |
|||
|
|||
switch (hal_status) |
|||
{ |
|||
case HAL_OK : |
|||
usb_status = USBD_OK; |
|||
break; |
|||
case HAL_ERROR : |
|||
usb_status = USBD_FAIL; |
|||
break; |
|||
case HAL_BUSY : |
|||
usb_status = USBD_BUSY; |
|||
break; |
|||
case HAL_TIMEOUT : |
|||
usb_status = USBD_FAIL; |
|||
break; |
|||
default : |
|||
usb_status = USBD_FAIL; |
|||
break; |
|||
} |
|||
return usb_status; |
|||
} |
@ -0,0 +1,173 @@ |
|||
/* USER CODE BEGIN Header */ |
|||
/**
|
|||
****************************************************************************** |
|||
* @file : usbd_conf.h |
|||
* @version : v1.0_Cube |
|||
* @brief : Header for usbd_conf.c file. |
|||
****************************************************************************** |
|||
* @attention |
|||
* |
|||
* Copyright (c) 2023 STMicroelectronics. |
|||
* All rights reserved. |
|||
* |
|||
* This software is licensed under terms that can be found in the LICENSE file |
|||
* in the root directory of this software component. |
|||
* If no LICENSE file comes with this software, it is provided AS-IS. |
|||
* |
|||
****************************************************************************** |
|||
*/ |
|||
/* USER CODE END Header */ |
|||
|
|||
/* Define to prevent recursive inclusion -------------------------------------*/ |
|||
#ifndef __USBD_CONF__H__ |
|||
#define __USBD_CONF__H__ |
|||
|
|||
#ifdef __cplusplus |
|||
extern "C" { |
|||
#endif |
|||
|
|||
/* Includes ------------------------------------------------------------------*/ |
|||
#include <stdio.h> |
|||
#include <stdlib.h> |
|||
#include <string.h> |
|||
#include "main.h" |
|||
#include "stm32f4xx.h" |
|||
#include "stm32f4xx_hal.h" |
|||
|
|||
/* USER CODE BEGIN INCLUDE */ |
|||
|
|||
/* USER CODE END INCLUDE */ |
|||
|
|||
/** @addtogroup USBD_OTG_DRIVER
|
|||
* @brief Driver for Usb device. |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CONF USBD_CONF
|
|||
* @brief Configuration file for Usb otg low level driver. |
|||
* @{ |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CONF_Exported_Variables USBD_CONF_Exported_Variables
|
|||
* @brief Public variables. |
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CONF_Exported_Defines USBD_CONF_Exported_Defines
|
|||
* @brief Defines for configuration of the Usb device. |
|||
* @{ |
|||
*/ |
|||
|
|||
/*---------- -----------*/ |
|||
#define USBD_MAX_NUM_INTERFACES 1U |
|||
/*---------- -----------*/ |
|||
#define USBD_MAX_NUM_CONFIGURATION 1U |
|||
/*---------- -----------*/ |
|||
#define USBD_MAX_STR_DESC_SIZ 512U |
|||
/*---------- -----------*/ |
|||
#define USBD_DEBUG_LEVEL 0U |
|||
/*---------- -----------*/ |
|||
#define USBD_LPM_ENABLED 0U |
|||
/*---------- -----------*/ |
|||
#define USBD_SELF_POWERED 1U |
|||
|
|||
/****************************************/ |
|||
/* #define for FS and HS identification */ |
|||
#define DEVICE_FS 0 |
|||
#define DEVICE_HS 1 |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CONF_Exported_Macros USBD_CONF_Exported_Macros
|
|||
* @brief Aliases. |
|||
* @{ |
|||
*/ |
|||
/* Memory management macros make sure to use static memory allocation */ |
|||
/** Alias for memory allocation. */ |
|||
|
|||
#define USBD_malloc (void *)USBD_static_malloc |
|||
|
|||
/** Alias for memory release. */ |
|||
#define USBD_free USBD_static_free |
|||
|
|||
/** Alias for memory set. */ |
|||
#define USBD_memset memset |
|||
|
|||
/** Alias for memory copy. */ |
|||
#define USBD_memcpy memcpy |
|||
|
|||
/** Alias for delay. */ |
|||
#define USBD_Delay HAL_Delay |
|||
|
|||
/* DEBUG macros */ |
|||
|
|||
#if (USBD_DEBUG_LEVEL > 0) |
|||
#define USBD_UsrLog(...) printf(__VA_ARGS__);\ |
|||
printf("\n"); |
|||
#else |
|||
#define USBD_UsrLog(...) |
|||
#endif /* (USBD_DEBUG_LEVEL > 0U) */ |
|||
|
|||
#if (USBD_DEBUG_LEVEL > 1) |
|||
|
|||
#define USBD_ErrLog(...) printf("ERROR: ");\ |
|||
printf(__VA_ARGS__);\ |
|||
printf("\n"); |
|||
#else |
|||
#define USBD_ErrLog(...) |
|||
#endif /* (USBD_DEBUG_LEVEL > 1U) */ |
|||
|
|||
#if (USBD_DEBUG_LEVEL > 2) |
|||
#define USBD_DbgLog(...) printf("DEBUG : ");\ |
|||
printf(__VA_ARGS__);\ |
|||
printf("\n"); |
|||
#else |
|||
#define USBD_DbgLog(...) |
|||
#endif /* (USBD_DEBUG_LEVEL > 2U) */ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CONF_Exported_Types USBD_CONF_Exported_Types
|
|||
* @brief Types. |
|||
* @{ |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/** @defgroup USBD_CONF_Exported_FunctionsPrototype USBD_CONF_Exported_FunctionsPrototype
|
|||
* @brief Declaration of public functions for Usb device. |
|||
* @{ |
|||
*/ |
|||
|
|||
/* Exported functions -------------------------------------------------------*/ |
|||
void *USBD_static_malloc(uint32_t size); |
|||
void USBD_static_free(void *p); |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
/**
|
|||
* @} |
|||
*/ |
|||
|
|||
#ifdef __cplusplus |
|||
} |
|||
#endif |
|||
|
|||
#endif /* __USBD_CONF__H__ */ |
|||
|
@ -0,0 +1,77 @@ |
|||
<?xml version="1.0" encoding="UTF-8" standalone="no"?> |
|||
<launchConfiguration type="com.st.stm32cube.ide.mcu.debug.launch.launchConfigurationType"> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.access_port_id" value="0"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.cubeprog_external_loaders" value="[]"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.enable_live_expr" value="true"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.enable_swv" value="false"/> |
|||
<intAttribute key="com.st.stm32cube.ide.mcu.debug.launch.formatVersion" value="2"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.ip_address_local" value="localhost"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.limit_swo_clock.enabled" value="false"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.limit_swo_clock.value" value=""/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.loadList" value="{"fItems":[{"fIsFromMainTab":true,"fPath":"Debug/raw407cxx.elf","fProjectName":"raw407cxx","fPerformBuild":true,"fDownload":true,"fLoadSymbols":true}]}"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.override_start_address_mode" value="default"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.remoteCommand" value="target remote"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.startServer" value="true"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.startuptab.exception.divby0" value="true"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.startuptab.exception.unaligned" value="false"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.startuptab.haltonexception" value="true"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.swd_mode" value="true"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.swv_port" value="61235"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.swv_trace_hclk" value="168000000"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.useRemoteTarget" value="true"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.launch.vector_table" value=""/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.launch.verify_flash_download" value="true"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.cti_allow_halt" value="false"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.cti_signal_halt" value="false"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.enable_logging" value="false"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.enable_max_halt_delay" value="false"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.enable_shared_stlink" value="false"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.frequency" value="0"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.halt_all_on_reset" value="false"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.log_file" value="/Users/heck/src/STM32CubeIDE/workspace1/raw407cxx/Debug/st-link_gdbserver_log.txt"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.low_power_debug" value="enable"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.max_halt_delay" value="2"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.reset_strategy" value="connect_under_reset"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.stlink_check_serial_number" value="false"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.stlink_txt_serial_number" value=""/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.stlink.watchdog_config" value="none"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.debug.stlinkenable_rtos" value="false"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.debug.stlinkrestart_configurations" value="{"fVersion":1,"fItems":[{"fDisplayName":"Reset","fIsSuppressible":false,"fResetAttribute":"Software system reset","fResetStrategies":[{"fDisplayName":"Software system reset","fLaunchAttribute":"system_reset","fGdbCommands":["monitor reset\n"],"fCmdOptions":["-g"]},{"fDisplayName":"Hardware reset","fLaunchAttribute":"hardware_reset","fGdbCommands":["monitor reset hardware\n"],"fCmdOptions":["-g"]},{"fDisplayName":"Core reset","fLaunchAttribute":"core_reset","fGdbCommands":["monitor reset core\n"],"fCmdOptions":["-g"]},{"fDisplayName":"None","fLaunchAttribute":"no_reset","fGdbCommands":[],"fCmdOptions":["-g"]}],"fGdbCommandGroup":{"name":"Additional commands","commands":[]},"fStartApplication":true}]}"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.rtosproxy.enableRtosProxy" value="false"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.rtosproxy.rtosProxyCustomProperties" value=""/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.rtosproxy.rtosProxyDriver" value="threadx"/> |
|||
<booleanAttribute key="com.st.stm32cube.ide.mcu.rtosproxy.rtosProxyDriverAuto" value="false"/> |
|||
<stringAttribute key="com.st.stm32cube.ide.mcu.rtosproxy.rtosProxyDriverPort" value="cortex_m0"/> |
|||
<intAttribute key="com.st.stm32cube.ide.mcu.rtosproxy.rtosProxyPort" value="60000"/> |
|||
<booleanAttribute key="org.eclipse.cdt.debug.gdbjtag.core.doHalt" value="false"/> |
|||
<booleanAttribute key="org.eclipse.cdt.debug.gdbjtag.core.doReset" value="false"/> |
|||
<stringAttribute key="org.eclipse.cdt.debug.gdbjtag.core.initCommands" value=""/> |
|||
<stringAttribute key="org.eclipse.cdt.debug.gdbjtag.core.ipAddress" value="localhost"/> |
|||
<stringAttribute key="org.eclipse.cdt.debug.gdbjtag.core.jtagDeviceId" value="com.st.stm32cube.ide.mcu.debug.stlink"/> |
|||
<stringAttribute key="org.eclipse.cdt.debug.gdbjtag.core.pcRegister" value=""/> |
|||
<intAttribute key="org.eclipse.cdt.debug.gdbjtag.core.portNumber" value="61234"/> |
|||
<stringAttribute key="org.eclipse.cdt.debug.gdbjtag.core.runCommands" value=""/> |
|||
<booleanAttribute key="org.eclipse.cdt.debug.gdbjtag.core.setPcRegister" value="false"/> |
|||
<booleanAttribute key="org.eclipse.cdt.debug.gdbjtag.core.setResume" value="true"/> |
|||
<booleanAttribute key="org.eclipse.cdt.debug.gdbjtag.core.setStopAt" value="true"/> |
|||
<stringAttribute key="org.eclipse.cdt.debug.gdbjtag.core.stopAt" value="main"/> |
|||
<stringAttribute key="org.eclipse.cdt.dsf.gdb.DEBUG_NAME" value="arm-none-eabi-gdb"/> |
|||
<booleanAttribute key="org.eclipse.cdt.dsf.gdb.NON_STOP" value="false"/> |
|||
<booleanAttribute key="org.eclipse.cdt.dsf.gdb.UPDATE_THREADLIST_ON_SUSPEND" value="false"/> |
|||
<intAttribute key="org.eclipse.cdt.launch.ATTR_BUILD_BEFORE_LAUNCH_ATTR" value="2"/> |
|||
<stringAttribute key="org.eclipse.cdt.launch.COREFILE_PATH" value=""/> |
|||
<stringAttribute key="org.eclipse.cdt.launch.DEBUGGER_START_MODE" value="remote"/> |
|||
<booleanAttribute key="org.eclipse.cdt.launch.DEBUGGER_STOP_AT_MAIN" value="true"/> |
|||
<stringAttribute key="org.eclipse.cdt.launch.DEBUGGER_STOP_AT_MAIN_SYMBOL" value="main"/> |
|||
<stringAttribute key="org.eclipse.cdt.launch.PROGRAM_NAME" value="Debug/raw407cxx.elf"/> |
|||
<stringAttribute key="org.eclipse.cdt.launch.PROJECT_ATTR" value="raw407cxx"/> |
|||
<booleanAttribute key="org.eclipse.cdt.launch.PROJECT_BUILD_CONFIG_AUTO_ATTR" value="true"/> |
|||
<stringAttribute key="org.eclipse.cdt.launch.PROJECT_BUILD_CONFIG_ID_ATTR" value="com.st.stm32cube.ide.mcu.gnu.managedbuild.config.exe.debug.1588923769"/> |
|||
<listAttribute key="org.eclipse.debug.core.MAPPED_RESOURCE_PATHS"> |
|||
<listEntry value="/raw407cxx"/> |
|||
</listAttribute> |
|||
<listAttribute key="org.eclipse.debug.core.MAPPED_RESOURCE_TYPES"> |
|||
<listEntry value="4"/> |
|||
</listAttribute> |
|||
<stringAttribute key="process_factory_id" value="com.st.stm32cube.ide.mcu.debug.launch.HardwareDebugProcessFactory"/> |
|||
</launchConfiguration> |
Loading…
Reference in new issue