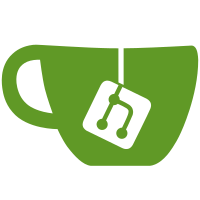
3 changed files with 231 additions and 0 deletions
@ -0,0 +1,67 @@ |
|||
#!/bin/bash |
|||
|
|||
# Just a normal copy program, BUT it never overwrites a file in the destination. |
|||
# if the filename is already taken, the new file will get an incrementing number as postfix added just in fron of the dot before the extension. |
|||
# no extension, its added the end. |
|||
# example: filename.wav already exists, new file will get the name filename_1.wav. |
|||
# |
|||
# usage cp_keep.sh [src file] [dest folder] [prefix newfile] |
|||
|
|||
|
|||
export IFS=$'\n'; |
|||
export src=$1; |
|||
export dst=$2; |
|||
export prefix=$3 |
|||
|
|||
if [ -z $1 ]; then { |
|||
echo "must specify source file"; |
|||
exit; |
|||
} fi |
|||
|
|||
if [ -z $2 ]; then { |
|||
echo "must specify destination directory"; |
|||
exit; |
|||
} fi |
|||
|
|||
|
|||
if [ ! -d $2 ]; then { |
|||
echo "destination must be a directory"; |
|||
exit; |
|||
} fi |
|||
|
|||
if [ -d $1 ]; then { |
|||
echo "$1 is directory. .. quitting."; |
|||
exit; |
|||
} fi |
|||
|
|||
export src_dir=$(dirname $src); |
|||
export src_file=$(basename $src); |
|||
|
|||
|
|||
if [ "$dst" -ef "$src_dir" ]; then { |
|||
echo "source and destiation are same directory... quitting" |
|||
exit; |
|||
} fi |
|||
|
|||
export fname_new=$prefix$src_file; |
|||
|
|||
count=1; |
|||
while [ -e $dst/$fname_new ]; do { #destination filename already taken? |
|||
#add suffix before any dot, if no dot just append |
|||
if [[ "$fname_new" =~ \. ]]; then { |
|||
fname_new=$(echo $fname_new | sed -e "s/\./_$count\./g"); |
|||
} else { |
|||
fname_new=$fname_new"_$count" |
|||
} fi |
|||
# echo "filename already taken, suffix added: $dst/$fname_new" |
|||
count=$(($count+1)); |
|||
} done |
|||
|
|||
if [ "$count" -gt 1 ]; then { |
|||
echo "$dst/$src_file already existed, new name: $fname_new"; |
|||
} fi |
|||
|
|||
export cmd="cp \"$src_dir/$src_file\" \"$dst/$fname_new\""; |
|||
|
|||
#echo $cmd; |
|||
eval $cmd; |
@ -0,0 +1,104 @@ |
|||
#!/usr/bin/perl |
|||
|
|||
my $help = <<'END_HELP'; |
|||
Sampling tool File Renamer |
|||
usage: renamer.pl DIR SEARCHREGEX REPLACEREGEX DOIT |
|||
|
|||
for every regular file in DIR, sorted by mtime, |
|||
replaces the SEARCHREGEX with REPLACEREGEX in every filename. |
|||
Does NOT rename anything unless DOIT! |
|||
|
|||
Special Variables are: |
|||
%NR% - the index number for the file with the current sorting |
|||
|
|||
no captures support in regex :( |
|||
END_HELP |
|||
|
|||
|
|||
use strict; |
|||
use warnings; |
|||
|
|||
use Data::Dumper qw(Dumper); |
|||
use POSIX qw(strftime); |
|||
use Cwd; |
|||
use File::stat; |
|||
|
|||
my $dir = "-?"; |
|||
$dir = $ARGV[0]; |
|||
my $regexSearch = $ARGV[1]; |
|||
my $regexReplace = $ARGV[2]; |
|||
my $reallyDoIt = $ARGV[3]; |
|||
|
|||
if ($dir eq "-?") { |
|||
usage(); |
|||
} |
|||
|
|||
if(!$dir) { |
|||
print "Error: Need a directory.\n"; |
|||
die "\n"; |
|||
} |
|||
if(!$regexSearch) { |
|||
print "Error: Need a search regex.\n"; |
|||
die "\n"; |
|||
} |
|||
if(!$regexReplace) { |
|||
print "Error: Need a replace regex.\n\n"; |
|||
die "\n"; |
|||
} |
|||
|
|||
chdir($dir) || die "FATAL: cant chdir"; |
|||
|
|||
print getcwd()."\n"; |
|||
|
|||
opendir( DIR, getcwd() ); |
|||
my @files = readdir(DIR); |
|||
closedir(DIR); |
|||
|
|||
#filter out dirs |
|||
my @filesNoDir; |
|||
foreach (@files) { |
|||
if(! -d $_) { |
|||
push(@filesNoDir,$_); |
|||
} |
|||
} |
|||
|
|||
@files = @filesNoDir; |
|||
|
|||
my @sorted_files = sort { stat($a)->mtime <=> stat($b)->mtime } @files; |
|||
|
|||
my $fname = ""; |
|||
my $fstat = ""; |
|||
my $mtime = ""; |
|||
|
|||
my $fname_new = ""; |
|||
my $counter = 1; |
|||
my $regexReplaceNew = ""; |
|||
my $counterFmt; |
|||
for (@sorted_files) { |
|||
$fname = $_; |
|||
$fstat = stat($_); |
|||
$mtime = strftime('%Y/%m/%d %H:%M:%S',localtime($fstat->mtime)); |
|||
|
|||
$regexReplaceNew = $regexReplace; |
|||
$counterFmt = sprintf("%03s",$counter); |
|||
$regexReplaceNew =~ s/%NR%/$counterFmt/g; |
|||
|
|||
$fname_new = $fname; |
|||
$fname_new =~ s/$regexSearch/$regexReplaceNew/; |
|||
# $fname_new =~ s/(.*?)\s1-/%NR%_$1/; |
|||
|
|||
printf "%-40s%-40s%-20s\n",$fname_new,$fname,$mtime; |
|||
|
|||
if($reallyDoIt) { |
|||
rename($fname,$fname_new); |
|||
} |
|||
|
|||
$counter++; |
|||
} |
|||
|
|||
|
|||
|
|||
sub usage { |
|||
print $help; |
|||
die "\n"; |
|||
} |
@ -0,0 +1,60 @@ |
|||
#!/bin/bash |
|||
|
|||
# Replaces odd characters in filenames and directories with an underline |
|||
# When there are more consecutive odd characters what would result in ____ sth like this, it collates to a single underline. |
|||
# When the new unixified filename is already taken, a counted suffix will be added before an eventuel period in the filename, otherwise just append. |
|||
# Will never overwrite a file. |
|||
|
|||
# usage: inixify.sh [filename] |
|||
# filename The file to unixify, can be file or folder. |
|||
|
|||
|
|||
export IFS=$'\n'; |
|||
export fname_full=$1; |
|||
#export out=$2; |
|||
|
|||
function sanitise { |
|||
local retval=$(echo $1 | gsed -e 's/[^A-Za-z0-9#./_-]/_/g'); # replace everything else with _ |
|||
retval=$(echo $retval | gsed -e 's/\.\{2,\}/\./g'); # replace >1 consecutive . with one . |
|||
retval=$(echo $retval | gsed -e 's/_\{2,\}/_/g'); # replace >1 consecutive _ with one _ |
|||
|
|||
echo $retval; |
|||
} |
|||
|
|||
export current_dir=$(dirname $fname_full); |
|||
export current_file=$(basename $fname_full); |
|||
|
|||
export fname_new=$(sanitise $current_file); # unixify |
|||
|
|||
if [ "$current_file" != "$fname_new" ]; then { #any changes been needed? |
|||
|
|||
count=1; |
|||
while [ -e $current_dir/$fname_new ]; do { #new filename already taken? |
|||
#echo file exists: $current_dir/$fname_new; |
|||
|
|||
#add suffix before any dot, if no dot just append |
|||
if [[ "$fname_new" =~ \. ]]; then { |
|||
fname_new=$(echo $fname_new | sed -e "s/\./_$count\./g"); |
|||
} else { |
|||
fname_new=$fname_new"_$count" |
|||
} fi |
|||
|
|||
count=$(($count+1)); |
|||
} done |
|||
|
|||
echo -n $current_dir/$fname_new |
|||
|
|||
if [ "$count" -gt 1 ]; then { |
|||
echo " suffix nr added: $(($count-1))"; |
|||
} else { |
|||
echo ""; |
|||
} fi |
|||
|
|||
export cmd="mv \"$fname_full\" \"$current_dir/$fname_new\""; |
|||
|
|||
# echo $cmd; |
|||
eval $cmd; |
|||
} else { |
|||
echo -ne; |
|||
echo $fname_full" filename already ok."; |
|||
} fi |
Loading…
Reference in new issue